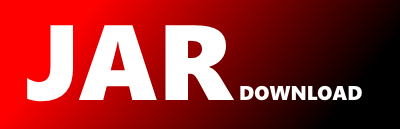
com.sap.cds.reflect.impl.CdsElementReader Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect.impl;
import static com.sap.cds.reflect.impl.CdsModelReader.findType;
import static com.sap.cds.reflect.impl.CdsModelReader.readType;
import java.util.Iterator;
import java.util.Map.Entry;
import com.fasterxml.jackson.databind.JsonNode;
import com.sap.cds.reflect.impl.reader.issuecollector.IssueCollector;
import com.sap.cds.reflect.impl.reader.issuecollector.IssueCollectorFactory;
import com.sap.cds.reflect.impl.reader.model.CdsConstants;
public class CdsElementReader {
private static final IssueCollector issueCollector = IssueCollectorFactory
.getIssueCollector(CdsElementReader.class);
private final String pathToElement;
private final String elementName;
private final CdsModelBuilder model;
public CdsElementReader(String pathToElement, String elementName, CdsModelBuilder model) {
this.pathToElement = pathToElement;
this.elementName = elementName;
this.model = model;
}
public CdsElementBuilder> read(JsonNode csn) {
CdsTypeBuilder> type = findType(csn, model).orElseGet(() -> readType(pathToElement, csn, model));
Iterator> fields = csn.fields();
boolean isKey = false;
boolean isVirtual = false;
boolean isLocalized = false;
boolean notNull = false;
while (fields.hasNext()) {
String property = fields.next().getKey();
if (!CdsAnnotationReader.isAnnotation(property)) {
switch (property) {
case CdsConstants.ITEMS:
case CdsConstants.TYPE:
case CdsConstants.LENGTH:
case CdsConstants.PRECISION:
case CdsConstants.SCALE:
case CdsConstants.ORIGIN:
case CdsConstants.DEFAULT:
case CdsConstants.ENUM:
case CdsConstants.CDS_LOCALIZED:
case CdsConstants.ELEMENTS:
case CdsConstants.INDEXNUM:
case CdsConstants.$INFERRED:
case CdsConstants.VALUE:
case CdsConstants.ON:
case CdsConstants.TARGET:
case CdsConstants.CARDINALITY:
break;
case CdsConstants.LOCALIZEDCSN:
isLocalized = csn.get(property).asBoolean();
break;
case CdsConstants.KEYS:
break;
case CdsConstants.KEY:
isKey = csn.get(property).asBoolean();
break;
case CdsConstants.VIRTUAL:
isVirtual = csn.get(property).asBoolean();
break;
case CdsConstants.NOT_NULL:
notNull = csn.get(property).asBoolean();
break;
default:
issueCollector.unrecognized(pathToElement,
"The element '%s' contains an unrecognized property '%s'.", pathToElement, property);
}
}
}
CdsElementBuilder> newElement = new CdsElementBuilder<>(CdsAnnotationReader.read(csn), elementName, type,
isKey, isVirtual, notNull, isLocalized);
return newElement;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy