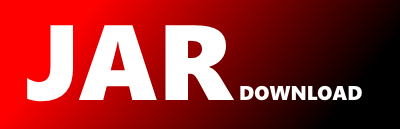
com.sap.cds.ql.impl.UpdateBuilder Maven / Gradle / Ivy
/**************************************************************************
* (C) 2019-2023 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.ql.impl;
import static com.sap.cds.impl.builder.model.StructuredTypeImpl.structuredType;
import static com.sap.cds.impl.builder.model.StructuredTypeRefImpl.typeRef;
import static com.sap.cds.util.DataUtils.deepMapKeys;
import static java.util.Collections.emptyMap;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.stream.Stream;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.sap.cds.impl.parser.token.Jsonizer;
import com.sap.cds.ql.StructuredType;
import com.sap.cds.ql.Update;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.CqnUpdate;
import com.sap.cds.ql.cqn.Modifier;
import com.sap.cds.util.DataUtils;
public class UpdateBuilder> extends FilterableStatementBuilder>
implements Update {
private final T entity;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy