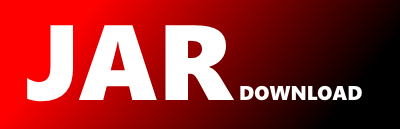
com.sap.cds.reflect.impl.CdsVersion Maven / Gradle / Ivy
/*******************************************************************
* © 2021 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect.impl;
public final class CdsVersion implements Comparable {
private int major;
private int minor;
private int micro;
private int buildNumber;
public CdsVersion(int major, int minor, int micro, int buildNumber) {
super();
this.major = major;
this.minor = minor;
this.micro = micro;
this.buildNumber = buildNumber;
}
public int major() {
return major;
}
public int minor() {
return minor;
}
public int micro() {
return micro;
}
public int buildNumber() {
return buildNumber;
}
/**
* Compares the compiler versions based on the normalized version which is
* calculated as major*10^p*3 + minor*10^p*2 + micro*10^p +
* buildNumber, where p - power, is the max precision
* length of the version segment.
*
* @param other the version to be compared
* @return a negative integer, zero, or a positive integer as this version is
* less than, equal to, or greater than the specified version
*/
@Override
public int compareTo(CdsVersion other) {
int me[] = new int[] { major, minor, micro, buildNumber };
int another[] = new int[] { other.major, other.minor, other.micro, other.buildNumber };
return compare(me, another);
}
private int compare(int[] me, int another[]) {
for (int x = 0; x < me.length; x++) {
if (me[x] != another[x]) {
return Integer.compare(me[x], another[x]);
}
}
return 0;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof CdsVersion) {
return compareTo((CdsVersion) obj) == 0;
}
return false;
}
@Override
public int hashCode() {
return major << 12 + minor << 8 + micro << 4 + buildNumber;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy