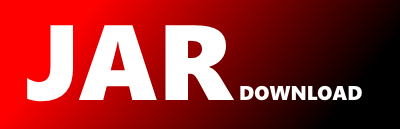
com.sap.cloud.alert.notification.client.model.configuration.Action Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clm-sl-alert-notification-client Show documentation
Show all versions of clm-sl-alert-notification-client Show documentation
Means for out-of-the-box events management in SAP Alert Notification service for SAP BTP service.
package com.sap.cloud.alert.notification.client.model.configuration;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.EqualsAndHashCode;
import lombok.ToString;
import org.apache.commons.collections4.CollectionUtils;
import java.io.Serializable;
import java.util.*;
import static java.util.Collections.unmodifiableMap;
import static java.util.Collections.unmodifiableSet;
import static org.apache.commons.collections4.MapUtils.emptyIfNull;
@JsonIgnoreProperties(ignoreUnknown = true)
@ToString(exclude = "properties", doNotUseGetters = true)
@EqualsAndHashCode(doNotUseGetters = true, exclude = { "timeCreated", "lastModified" })
public class Action implements Serializable {
private static final long serialVersionUID = 1L;
private final String id;
private final String type;
private final String name;
private final State state;
private final String description;
private final Set labels;
private final Integer discardAfter;
private final Integer fallbackTime;
private final String fallbackAction;
private final Map properties;
private final Long timeCreated;
private final Long lastModified;
@JsonCreator
public Action(
@JsonProperty("id") String id,
@JsonProperty("type") String type,
@JsonProperty("name") String name,
@JsonProperty("state") State state,
@JsonProperty("description") String description,
@JsonProperty("labels") Collection labels,
@JsonProperty("discardAfter") Integer discardAfter,
@JsonProperty("fallbackTime") Integer fallbackTime,
@JsonProperty("fallbackAction") String fallbackAction,
@JsonProperty("properties") Map properties,
@JsonProperty("timeCreated") Long timeCreated,
@JsonProperty("lastModified") Long lastModified
) {
this.id = id;
this.type = type;
this.name = name;
this.state = state;
this.description = description;
this.labels = unmodifiableSet(new HashSet<>(CollectionUtils.emptyIfNull(labels)));
this.discardAfter = discardAfter;
this.fallbackTime = fallbackTime;
this.fallbackAction = fallbackAction;
this.properties = unmodifiableMap(new HashMap<>(emptyIfNull(properties)));
this.timeCreated = timeCreated;
this.lastModified = lastModified;
}
public Action(
String type,
String name,
State state,
String description,
Collection labels,
Integer discardAfter,
Integer fallbackTime,
String fallbackAction,
Map properties
) {
this(null, type, name, state, description, labels, discardAfter, fallbackTime, fallbackAction, properties, null, null);
}
@JsonProperty("id")
public String getId() {
return id;
}
@JsonProperty("name")
public String getName() {
return name;
}
@JsonProperty("type")
public String getType() {
return type;
}
@JsonProperty("state")
public State getState() {
return state;
}
@JsonProperty("labels")
public Collection getLabels() {
return unmodifiableSet(labels);
}
@JsonProperty("properties")
public Map getProperties() {
return unmodifiableMap(properties);
}
@JsonProperty("description")
public String getDescription() {
return description;
}
@JsonProperty("fallbackAction")
public String getFallbackAction() {
return fallbackAction;
}
@JsonProperty("discardAfter")
public Integer getDiscardAfter() {
return discardAfter;
}
@JsonProperty("fallbackTime")
public Integer getFallbackTime() {
return fallbackTime;
}
@JsonProperty("timeCreated")
public Long getTimeCreated() {
return timeCreated;
}
@JsonProperty("lastModified")
public Long getLastModified() {
return lastModified;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy