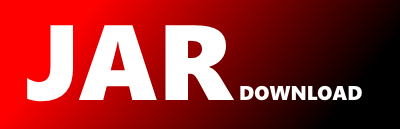
com.sap.gateway.v4.rt.jdbc.hana.HanaSqlHelper Maven / Gradle / Ivy
/*package com.sap.gateway.v4.rt.jdbc.hana;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.sql.Types;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.olingo.commons.api.edm.EdmEntityType;
import org.apache.olingo.commons.api.edm.EdmKeyPropertyRef;
import org.apache.olingo.commons.api.edm.EdmNavigationProperty;
import org.apache.olingo.commons.api.edm.EdmReferentialConstraint;
import org.apache.olingo.server.api.uri.UriParameter;
public class HanaSqlHelper {
static int c = 0;
public static List getKeyPropertyNamesFromEntityType(EdmEntityType eType,String label) {
List kpRef = eType.getKeyPropertyRefs();
List keyPropertyNames = new ArrayList();
for(EdmKeyPropertyRef propertyRef : kpRef){
if(label != null)
keyPropertyNames.add('"' + label + '"' + '.' + '"' + propertyRef.getProperty().getName() + '"');
else
keyPropertyNames.add(propertyRef.getProperty().getName());
}
return keyPropertyNames;
}
public static String constructOverInSQL(List orderby) {
String overFormula = " row_number() over";
String orderByString = "";
if(orderby.isEmpty()) {
return overFormula + " () " + "1row";
}
overFormula += "(ORDER BY ";
for(String orderByProp : orderby) {
if(!orderByString.equals(""))
orderByString += ',';
orderByString += orderByProp;
}
return overFormula + orderByString + ") \"1row\" ";
}
public static String getSQLQueryWith0123AliasedKeysForSelect(EdmEntityType entityType, String table, String schema) {
String schemaPrefix = "";
List keys = getKeyPropertyNamesFromEntityType(entityType,null);
if(schema!=null){
if(!(schema.equals(""))) {
schemaPrefix = '"' + schema + '"' + '.';
}
}
String keySql = "";
for(String key : keys) {
if(keySql != "")
keySql += ',';
keySql += schemaPrefix + '"' + table + '"' + '.' + '"' + key + '"' + ' ' + "\"" + inc() + "\"";
}
c = 0;
return keySql.equals("") ? "1":keySql;
}
private static int inc() {
return c++;
}
public static String getWhereStringFromKeyPredicates(List keyPredicates,String table, String schema) {
String schemaTablePrefix = "";
if(schema!=null){
if(!(schema.equals(""))) {
schemaTablePrefix = '"' + schema + '"' + '.' + '"' + table + '"';
}
}
else
schemaTablePrefix = '"' + table + '"';
String whereQuery = "";
for(UriParameter kp : keyPredicates) {
if(!whereQuery.equals(""))
whereQuery += " AND ";
whereQuery += schemaTablePrefix + '.' + '"' + kp.getName() + '"' + " = " + kp.getText();
}
return whereQuery;
}
public static String getJoinConditionsFromNavigationPropery(String previousTempTable, String currentTable,EdmNavigationProperty np, String schema) {
String joinQuery = "";
String previousTableWithQuotes = "";
String currentTableWithQuotes = "";
if(!previousTempTable.startsWith("#") && schema != null)
previousTableWithQuotes = '"' + schema + '"' + '.' + '"' + previousTempTable + '"';
else
previousTableWithQuotes = '"' + previousTempTable + '"';
if(!currentTable.startsWith("#") && schema != null)
currentTableWithQuotes = '"' + schema + '"' + '.' + '"' + currentTable + '"';
else
currentTableWithQuotes = '"' + currentTable + '"';
EdmNavigationProperty pp = np.getPartner();
List refCon = np.getReferentialConstraints();
if(refCon != null && !refCon.isEmpty()) {
for(EdmReferentialConstraint rc : refCon) {
if(!joinQuery.equals(""))
joinQuery += " AND ";
joinQuery += previousTableWithQuotes + '.' + '"' + rc.getPropertyName() + '"' + '=' + currentTableWithQuotes + '.' + '"' + rc.getReferencedPropertyName() + '"';
}
}
else {
List partnerRefCon = pp.getReferentialConstraints();
for(EdmReferentialConstraint rc : partnerRefCon) {
if(!joinQuery.equals(""))
joinQuery += " AND ";
joinQuery += currentTableWithQuotes + '.' + '"' + rc.getPropertyName() + '"' + '=' + previousTableWithQuotes + '.' + '"' + rc.getReferencedPropertyName() + '"';
}
}
return joinQuery;
}
public static String getSQLQueryWith0123AliasedKeysForCreate(EdmEntityType entityType) {
List keys = getKeyPropertyNamesFromEntityType(entityType,null);
String createSql = "";
for(String key : keys) {
if(createSql != "")
createSql += ',';
createSql += "\"" + inc() + "\" INTEGER";
}
c = 0;
return createSql;
}
public static String getTemporaryTableDefinition(EdmEntityType entityType, String tableDef)
{
c = 0;
List keys = getKeyPropertyNamesFromEntityType(entityType, null);
String hanaType = "";
String createSql = "";
String regex;
Pattern p;
Matcher m;
for (String key : keys) {
regex = "\"" + key + "\" [^,]*";
p = Pattern.compile(regex);
m = p.matcher(tableDef);
if (m.find())
hanaType = m.group().split(" ")[1];
if (createSql != "")
createSql = createSql + ',';
createSql = createSql + "\"" + inc() + "\" " + hanaType;
}
return createSql + tableDef;
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy