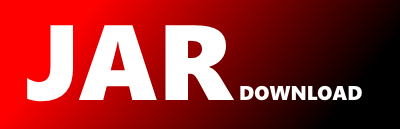
com.sap.gateway.v4.rt.jdbc.hana.api.HANAODataProvider Maven / Gradle / Ivy
package com.sap.gateway.v4.rt.jdbc.hana.api;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import org.apache.olingo.commons.api.data.Entity;
import org.apache.olingo.commons.api.edmx.EdmxReference;
import org.apache.olingo.server.api.OData;
import org.apache.olingo.server.api.ServiceMetadata;
import org.apache.olingo.server.api.uri.UriInfo;
import org.apache.olingo.server.core.uri.parser.Parser;
import com.sap.gateway.v4.rt.jdbc.hana.HANADataProvider;
import com.sap.gateway.v4.rt.jdbc.hana.edm.HanaEdmProvider;
import com.sap.gateway.v4.rt.jdbc.util.DBODataArtifact;
public class HANAODataProvider {
/*
private HANADataProvider dataProvider;
private HanaEdmProvider edmProvider;
private String DB_USERNAME;
private String DB_PASSWORD;
private String DB_HOST;
private int DB_PORT;
private String SCHEMA;
private Connection conn;
public HANAODataProvider(String DB_HOST, int DB_PORT, String DB_USERNAME, String DB_PASSWORD, String SCHEMA, List artifacts)
{
this.DB_USERNAME = DB_USERNAME;
this.DB_HOST = DB_HOST;
this.DB_PASSWORD = DB_PASSWORD;
this.DB_PORT = DB_PORT;
this.SCHEMA = SCHEMA;
edmProvider = new HanaEdmProviderImpl(getConnection(), SCHEMA, artifacts);
dataProvider = new HANADataProvider(edmProvider);
}
public Connection getConnection()
{
if(conn==null)
{
try {
Class.forName("com.sap.db.jdbc.Driver");
String DB_READ_CONNECTION_URL = "jdbc:sap://" + DB_HOST + ":" + DB_PORT ;
conn = (Connection) DriverManager.getConnection(DB_READ_CONNECTION_URL,DB_USERNAME,DB_PASSWORD);
Statement stmtschema = conn.createStatement();
stmtschema.execute("SET SCHEMA " + SCHEMA);
} catch (Exception e) {
e.printStackTrace();
}
}
return conn;
}
/*
public EntityCollection getEntityCollection(String entityName)
{
EntityCollection ec = null;
OData odata = OData.newInstance();
ServiceMetadata edm = odata.createServiceMetadata(edmProvider, new ArrayList());
try {
UriInfo uriInfo = new Parser(edm.getEdm(),odata).parseUri(entityName, null, null);
ec = dataProvider.readEntityCollection(uriInfo);
} catch (Exception e) {
e.printStackTrace();
}
return ec;
}
public Entity getEntity(String entityNamewithKey)
{
Entity entity = null;
OData odata = OData.newInstance();
ServiceMetadata edm = odata.createServiceMetadata(edmProvider, new ArrayList());
try {
UriInfo uriInfo = new Parser(edm.getEdm(),odata).parseUri("/"+entityNamewithKey, null, null);
entity = dataProvider.readEntity(uriInfo,null).getEntity();
} catch (Exception e) {
e.printStackTrace();
}
return entity;
}
/*
public EntityCollection getEntityCollectionfromURL(String url)
{
EntityCollection ec = null;
OData odata = OData.newInstance();
ServiceMetadata edm = odata.createServiceMetadata(edmProvider, new ArrayList());
try {
String[] urlsplit = url.split(Pattern.quote("?"));
int len = urlsplit.length;
UriInfo uriInfo = new Parser(edm.getEdm(),odata).parseUri((len>0?urlsplit[0]:null), (len>1? "?" + urlsplit[1]:null), null);
ec = dataProvider.readEntityCollection(uriInfo);
} catch (Exception e) {
e.printStackTrace();
}
return ec;
}
*/
/*
public InputStream getResponsefromCollection(EntityCollection ec)
{
OData odata = OData.newInstance();
SerializerResult serializerResult = null;
ServiceMetadata edm = odata.createServiceMetadata(cdsEdmProvider, new ArrayList());
try {
ODataSerializer serializer = odata.createSerializer(ContentType.JSON);
String[] urlsplit = url.split(Pattern.quote("?"));
int len = urlsplit.length;
UriInfo uriInfo = new Parser(edm.getEdm(),odata).parseUri((len>0?urlsplit[0]:null), (len>1? "?" + urlsplit[1]:null), null);
ec = dataProvider.readEntityCollection(uriInfo);
EdmEntityType targetEntityType = null;
targetEntityType = ((UriResourceEntitySet) uriInfo
.getUriResourceParts().get(0)).getEntitySet()
.getEntityType();
ContextURL contextUrl = ContextURL.with().type(targetEntityType)
.build();
EntityCollectionSerializerOptions opts = EntityCollectionSerializerOptions
.with().contextURL(contextUrl)
// .select(selectOption)
.expand(uriInfo.getExpandOption()).build();
serializerResult = serializer.entityCollection(
edm, targetEntityType, ec, opts);
} catch (Exception e) {
}
return serializerResult.getContent();
}
*/
/*
public InputStream getJsonResponse(String url)
{
EntityCollection ec = null;
OData odata = OData.newInstance();
SerializerResult serializerResult = null;
ServiceMetadata edm = odata.createServiceMetadata(edmProvider, new ArrayList());
try {
ODataSerializer serializer = odata.createSerializer(ContentType.JSON);
String[] urlsplit = url.split(Pattern.quote("?"));
int len = urlsplit.length;
UriInfo uriInfo = new Parser(edm.getEdm(),odata).parseUri((len>0?urlsplit[0]:null), (len>1? "?" + urlsplit[1]:null), null);
ec = dataProvider.readEntityCollection(uriInfo);
EdmEntityType targetEntityType = null;
targetEntityType = ((UriResourceEntitySet) uriInfo
.getUriResourceParts().get(0)).getEntitySet()
.getEntityType();
ContextURL contextUrl = ContextURL.with().type(targetEntityType)
.build();
EntityCollectionSerializerOptions opts = EntityCollectionSerializerOptions
.with().contextURL(contextUrl)
// .select(selectOption)
.expand(uriInfo.getExpandOption()).build();
serializerResult = serializer.entityCollection(
edm, targetEntityType, ec, opts);
} catch (Exception e) {
}
return serializerResult.getContent();
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy