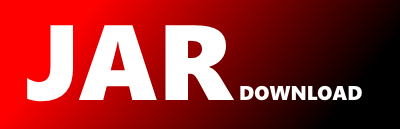
org.cloudfoundry.client.lib.adapters.ImmutableCloudFoundryClientFactory Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.adapters;
import java.time.Duration;
import java.util.Objects;
import java.util.Optional;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link CloudFoundryClientFactory}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudFoundryClientFactory.builder()}.
*/
@Generated(from = "CloudFoundryClientFactory", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableCloudFoundryClientFactory
extends CloudFoundryClientFactory {
private final Duration sslHandshakeTimeout;
private final Duration connectTimeout;
private final Integer connectionPoolSize;
private final Integer threadPoolSize;
private ImmutableCloudFoundryClientFactory(
Duration sslHandshakeTimeout,
Duration connectTimeout,
Integer connectionPoolSize,
Integer threadPoolSize) {
this.sslHandshakeTimeout = sslHandshakeTimeout;
this.connectTimeout = connectTimeout;
this.connectionPoolSize = connectionPoolSize;
this.threadPoolSize = threadPoolSize;
}
/**
* @return The value of the {@code sslHandshakeTimeout} attribute
*/
@Override
public Optional getSslHandshakeTimeout() {
return Optional.ofNullable(sslHandshakeTimeout);
}
/**
* @return The value of the {@code connectTimeout} attribute
*/
@Override
public Optional getConnectTimeout() {
return Optional.ofNullable(connectTimeout);
}
/**
* @return The value of the {@code connectionPoolSize} attribute
*/
@Override
public Optional getConnectionPoolSize() {
return Optional.ofNullable(connectionPoolSize);
}
/**
* @return The value of the {@code threadPoolSize} attribute
*/
@Override
public Optional getThreadPoolSize() {
return Optional.ofNullable(threadPoolSize);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudFoundryClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} attribute.
* @param value The value for sslHandshakeTimeout
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudFoundryClientFactory withSslHandshakeTimeout(Duration value) {
Duration newValue = Objects.requireNonNull(value, "sslHandshakeTimeout");
if (this.sslHandshakeTimeout == newValue) return this;
return new ImmutableCloudFoundryClientFactory(newValue, this.connectTimeout, this.connectionPoolSize, this.threadPoolSize);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudFoundryClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for sslHandshakeTimeout
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableCloudFoundryClientFactory withSslHandshakeTimeout(Optional extends Duration> optional) {
Duration value = optional.orElse(null);
if (this.sslHandshakeTimeout == value) return this;
return new ImmutableCloudFoundryClientFactory(value, this.connectTimeout, this.connectionPoolSize, this.threadPoolSize);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudFoundryClientFactory#getConnectTimeout() connectTimeout} attribute.
* @param value The value for connectTimeout
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudFoundryClientFactory withConnectTimeout(Duration value) {
Duration newValue = Objects.requireNonNull(value, "connectTimeout");
if (this.connectTimeout == newValue) return this;
return new ImmutableCloudFoundryClientFactory(this.sslHandshakeTimeout, newValue, this.connectionPoolSize, this.threadPoolSize);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudFoundryClientFactory#getConnectTimeout() connectTimeout} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for connectTimeout
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableCloudFoundryClientFactory withConnectTimeout(Optional extends Duration> optional) {
Duration value = optional.orElse(null);
if (this.connectTimeout == value) return this;
return new ImmutableCloudFoundryClientFactory(this.sslHandshakeTimeout, value, this.connectionPoolSize, this.threadPoolSize);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudFoundryClientFactory#getConnectionPoolSize() connectionPoolSize} attribute.
* @param value The value for connectionPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudFoundryClientFactory withConnectionPoolSize(int value) {
Integer newValue = value;
if (Objects.equals(this.connectionPoolSize, newValue)) return this;
return new ImmutableCloudFoundryClientFactory(this.sslHandshakeTimeout, this.connectTimeout, newValue, this.threadPoolSize);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudFoundryClientFactory#getConnectionPoolSize() connectionPoolSize} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for connectionPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudFoundryClientFactory withConnectionPoolSize(Optional optional) {
Integer value = optional.orElse(null);
if (Objects.equals(this.connectionPoolSize, value)) return this;
return new ImmutableCloudFoundryClientFactory(this.sslHandshakeTimeout, this.connectTimeout, value, this.threadPoolSize);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudFoundryClientFactory#getThreadPoolSize() threadPoolSize} attribute.
* @param value The value for threadPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudFoundryClientFactory withThreadPoolSize(int value) {
Integer newValue = value;
if (Objects.equals(this.threadPoolSize, newValue)) return this;
return new ImmutableCloudFoundryClientFactory(this.sslHandshakeTimeout, this.connectTimeout, this.connectionPoolSize, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudFoundryClientFactory#getThreadPoolSize() threadPoolSize} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for threadPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudFoundryClientFactory withThreadPoolSize(Optional optional) {
Integer value = optional.orElse(null);
if (Objects.equals(this.threadPoolSize, value)) return this;
return new ImmutableCloudFoundryClientFactory(this.sslHandshakeTimeout, this.connectTimeout, this.connectionPoolSize, value);
}
/**
* This instance is equal to all instances of {@code ImmutableCloudFoundryClientFactory} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudFoundryClientFactory
&& equalTo((ImmutableCloudFoundryClientFactory) another);
}
private boolean equalTo(ImmutableCloudFoundryClientFactory another) {
return Objects.equals(sslHandshakeTimeout, another.sslHandshakeTimeout)
&& Objects.equals(connectTimeout, another.connectTimeout)
&& Objects.equals(connectionPoolSize, another.connectionPoolSize)
&& Objects.equals(threadPoolSize, another.threadPoolSize);
}
/**
* Computes a hash code from attributes: {@code sslHandshakeTimeout}, {@code connectTimeout}, {@code connectionPoolSize}, {@code threadPoolSize}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(sslHandshakeTimeout);
h += (h << 5) + Objects.hashCode(connectTimeout);
h += (h << 5) + Objects.hashCode(connectionPoolSize);
h += (h << 5) + Objects.hashCode(threadPoolSize);
return h;
}
/**
* Prints the immutable value {@code CloudFoundryClientFactory} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("CloudFoundryClientFactory{");
if (sslHandshakeTimeout != null) {
builder.append("sslHandshakeTimeout=").append(sslHandshakeTimeout);
}
if (connectTimeout != null) {
if (builder.length() > 26) builder.append(", ");
builder.append("connectTimeout=").append(connectTimeout);
}
if (connectionPoolSize != null) {
if (builder.length() > 26) builder.append(", ");
builder.append("connectionPoolSize=").append(connectionPoolSize);
}
if (threadPoolSize != null) {
if (builder.length() > 26) builder.append(", ");
builder.append("threadPoolSize=").append(threadPoolSize);
}
return builder.append("}").toString();
}
/**
* Creates an immutable copy of a {@link CloudFoundryClientFactory} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CloudFoundryClientFactory instance
*/
public static ImmutableCloudFoundryClientFactory copyOf(CloudFoundryClientFactory instance) {
if (instance instanceof ImmutableCloudFoundryClientFactory) {
return (ImmutableCloudFoundryClientFactory) instance;
}
return ImmutableCloudFoundryClientFactory.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudFoundryClientFactory ImmutableCloudFoundryClientFactory}.
*
* ImmutableCloudFoundryClientFactory.builder()
* .sslHandshakeTimeout(java.time.Duration) // optional {@link CloudFoundryClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout}
* .connectTimeout(java.time.Duration) // optional {@link CloudFoundryClientFactory#getConnectTimeout() connectTimeout}
* .connectionPoolSize(Integer) // optional {@link CloudFoundryClientFactory#getConnectionPoolSize() connectionPoolSize}
* .threadPoolSize(Integer) // optional {@link CloudFoundryClientFactory#getThreadPoolSize() threadPoolSize}
* .build();
*
* @return A new ImmutableCloudFoundryClientFactory builder
*/
public static ImmutableCloudFoundryClientFactory.Builder builder() {
return new ImmutableCloudFoundryClientFactory.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudFoundryClientFactory ImmutableCloudFoundryClientFactory}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudFoundryClientFactory", generator = "Immutables")
public static final class Builder {
private Duration sslHandshakeTimeout;
private Duration connectTimeout;
private Integer connectionPoolSize;
private Integer threadPoolSize;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CloudFoundryClientFactory} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudFoundryClientFactory instance) {
Objects.requireNonNull(instance, "instance");
Optional sslHandshakeTimeoutOptional = instance.getSslHandshakeTimeout();
if (sslHandshakeTimeoutOptional.isPresent()) {
sslHandshakeTimeout(sslHandshakeTimeoutOptional);
}
Optional connectTimeoutOptional = instance.getConnectTimeout();
if (connectTimeoutOptional.isPresent()) {
connectTimeout(connectTimeoutOptional);
}
Optional connectionPoolSizeOptional = instance.getConnectionPoolSize();
if (connectionPoolSizeOptional.isPresent()) {
connectionPoolSize(connectionPoolSizeOptional);
}
Optional threadPoolSizeOptional = instance.getThreadPoolSize();
if (threadPoolSizeOptional.isPresent()) {
threadPoolSize(threadPoolSizeOptional);
}
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} to sslHandshakeTimeout.
* @param sslHandshakeTimeout The value for sslHandshakeTimeout
* @return {@code this} builder for chained invocation
*/
public final Builder sslHandshakeTimeout(Duration sslHandshakeTimeout) {
this.sslHandshakeTimeout = Objects.requireNonNull(sslHandshakeTimeout, "sslHandshakeTimeout");
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} to sslHandshakeTimeout.
* @param sslHandshakeTimeout The value for sslHandshakeTimeout
* @return {@code this} builder for use in a chained invocation
*/
public final Builder sslHandshakeTimeout(Optional extends Duration> sslHandshakeTimeout) {
this.sslHandshakeTimeout = sslHandshakeTimeout.orElse(null);
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getConnectTimeout() connectTimeout} to connectTimeout.
* @param connectTimeout The value for connectTimeout
* @return {@code this} builder for chained invocation
*/
public final Builder connectTimeout(Duration connectTimeout) {
this.connectTimeout = Objects.requireNonNull(connectTimeout, "connectTimeout");
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getConnectTimeout() connectTimeout} to connectTimeout.
* @param connectTimeout The value for connectTimeout
* @return {@code this} builder for use in a chained invocation
*/
public final Builder connectTimeout(Optional extends Duration> connectTimeout) {
this.connectTimeout = connectTimeout.orElse(null);
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getConnectionPoolSize() connectionPoolSize} to connectionPoolSize.
* @param connectionPoolSize The value for connectionPoolSize
* @return {@code this} builder for chained invocation
*/
public final Builder connectionPoolSize(int connectionPoolSize) {
this.connectionPoolSize = connectionPoolSize;
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getConnectionPoolSize() connectionPoolSize} to connectionPoolSize.
* @param connectionPoolSize The value for connectionPoolSize
* @return {@code this} builder for use in a chained invocation
*/
public final Builder connectionPoolSize(Optional connectionPoolSize) {
this.connectionPoolSize = connectionPoolSize.orElse(null);
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getThreadPoolSize() threadPoolSize} to threadPoolSize.
* @param threadPoolSize The value for threadPoolSize
* @return {@code this} builder for chained invocation
*/
public final Builder threadPoolSize(int threadPoolSize) {
this.threadPoolSize = threadPoolSize;
return this;
}
/**
* Initializes the optional value {@link CloudFoundryClientFactory#getThreadPoolSize() threadPoolSize} to threadPoolSize.
* @param threadPoolSize The value for threadPoolSize
* @return {@code this} builder for use in a chained invocation
*/
public final Builder threadPoolSize(Optional threadPoolSize) {
this.threadPoolSize = threadPoolSize.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableCloudFoundryClientFactory ImmutableCloudFoundryClientFactory}.
* @return An immutable instance of CloudFoundryClientFactory
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudFoundryClientFactory build() {
return new ImmutableCloudFoundryClientFactory(sslHandshakeTimeout, connectTimeout, connectionPoolSize, threadPoolSize);
}
}
}