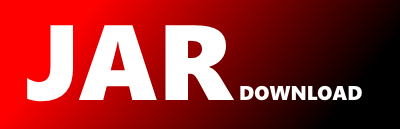
org.cloudfoundry.client.lib.adapters.ImmutableRawCloudApplication Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.adapters;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.client.lib.domain.CloudSpace;
import org.cloudfoundry.client.lib.domain.CloudStack;
import org.cloudfoundry.client.lib.domain.Derivable;
import org.cloudfoundry.client.v2.Resource;
import org.cloudfoundry.client.v2.applications.ApplicationEntity;
import org.cloudfoundry.client.v2.applications.SummaryApplicationResponse;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link RawCloudApplication}.
*
* Use the builder to create immutable instances:
* {@code ImmutableRawCloudApplication.builder()}.
*/
@Generated(from = "RawCloudApplication", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableRawCloudApplication
extends RawCloudApplication {
private final Resource resource;
private final SummaryApplicationResponse summary;
private final Derivable stack;
private final Derivable space;
private ImmutableRawCloudApplication(
Resource resource,
SummaryApplicationResponse summary,
Derivable stack,
Derivable space) {
this.resource = resource;
this.summary = summary;
this.stack = stack;
this.space = space;
}
/**
* @return The value of the {@code resource} attribute
*/
@Override
public Resource getResource() {
return resource;
}
/**
* @return The value of the {@code summary} attribute
*/
@Override
public SummaryApplicationResponse getSummary() {
return summary;
}
/**
* @return The value of the {@code stack} attribute
*/
@Override
public Derivable getStack() {
return stack;
}
/**
* @return The value of the {@code space} attribute
*/
@Override
public Derivable getSpace() {
return space;
}
/**
* Copy the current immutable object by setting a value for the {@link RawCloudApplication#getResource() resource} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for resource
* @return A modified copy of the {@code this} object
*/
public final ImmutableRawCloudApplication withResource(Resource value) {
if (this.resource == value) return this;
Resource newValue = Objects.requireNonNull(value, "resource");
return new ImmutableRawCloudApplication(newValue, this.summary, this.stack, this.space);
}
/**
* Copy the current immutable object by setting a value for the {@link RawCloudApplication#getSummary() summary} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for summary
* @return A modified copy of the {@code this} object
*/
public final ImmutableRawCloudApplication withSummary(SummaryApplicationResponse value) {
if (this.summary == value) return this;
SummaryApplicationResponse newValue = Objects.requireNonNull(value, "summary");
return new ImmutableRawCloudApplication(this.resource, newValue, this.stack, this.space);
}
/**
* Copy the current immutable object by setting a value for the {@link RawCloudApplication#getStack() stack} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stack
* @return A modified copy of the {@code this} object
*/
public final ImmutableRawCloudApplication withStack(Derivable value) {
if (this.stack == value) return this;
Derivable newValue = Objects.requireNonNull(value, "stack");
return new ImmutableRawCloudApplication(this.resource, this.summary, newValue, this.space);
}
/**
* Copy the current immutable object by setting a value for the {@link RawCloudApplication#getSpace() space} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for space
* @return A modified copy of the {@code this} object
*/
public final ImmutableRawCloudApplication withSpace(Derivable value) {
if (this.space == value) return this;
Derivable newValue = Objects.requireNonNull(value, "space");
return new ImmutableRawCloudApplication(this.resource, this.summary, this.stack, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableRawCloudApplication} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableRawCloudApplication
&& equalTo((ImmutableRawCloudApplication) another);
}
private boolean equalTo(ImmutableRawCloudApplication another) {
return resource.equals(another.resource)
&& summary.equals(another.summary)
&& stack.equals(another.stack)
&& space.equals(another.space);
}
/**
* Computes a hash code from attributes: {@code resource}, {@code summary}, {@code stack}, {@code space}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + resource.hashCode();
h += (h << 5) + summary.hashCode();
h += (h << 5) + stack.hashCode();
h += (h << 5) + space.hashCode();
return h;
}
/**
* Prints the immutable value {@code RawCloudApplication} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "RawCloudApplication{"
+ "resource=" + resource
+ ", summary=" + summary
+ ", stack=" + stack
+ ", space=" + space
+ "}";
}
/**
* Creates an immutable copy of a {@link RawCloudApplication} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable RawCloudApplication instance
*/
public static ImmutableRawCloudApplication copyOf(RawCloudApplication instance) {
if (instance instanceof ImmutableRawCloudApplication) {
return (ImmutableRawCloudApplication) instance;
}
return ImmutableRawCloudApplication.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableRawCloudApplication ImmutableRawCloudApplication}.
*
* ImmutableRawCloudApplication.builder()
* .resource(org.cloudfoundry.client.v2.Resource<org.cloudfoundry.client.v2.applications.ApplicationEntity>) // required {@link RawCloudApplication#getResource() resource}
* .summary(org.cloudfoundry.client.v2.applications.SummaryApplicationResponse) // required {@link RawCloudApplication#getSummary() summary}
* .stack(org.cloudfoundry.client.lib.domain.Derivable<org.cloudfoundry.client.lib.domain.CloudStack>) // required {@link RawCloudApplication#getStack() stack}
* .space(org.cloudfoundry.client.lib.domain.Derivable<org.cloudfoundry.client.lib.domain.CloudSpace>) // required {@link RawCloudApplication#getSpace() space}
* .build();
*
* @return A new ImmutableRawCloudApplication builder
*/
public static ImmutableRawCloudApplication.Builder builder() {
return new ImmutableRawCloudApplication.Builder();
}
/**
* Builds instances of type {@link ImmutableRawCloudApplication ImmutableRawCloudApplication}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "RawCloudApplication", generator = "Immutables")
public static final class Builder {
private static final long INIT_BIT_RESOURCE = 0x1L;
private static final long INIT_BIT_SUMMARY = 0x2L;
private static final long INIT_BIT_STACK = 0x4L;
private static final long INIT_BIT_SPACE = 0x8L;
private long initBits = 0xfL;
private Resource resource;
private SummaryApplicationResponse summary;
private Derivable stack;
private Derivable space;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code RawCloudApplication} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(RawCloudApplication instance) {
Objects.requireNonNull(instance, "instance");
resource(instance.getResource());
summary(instance.getSummary());
stack(instance.getStack());
space(instance.getSpace());
return this;
}
/**
* Initializes the value for the {@link RawCloudApplication#getResource() resource} attribute.
* @param resource The value for resource
* @return {@code this} builder for use in a chained invocation
*/
public final Builder resource(Resource resource) {
this.resource = Objects.requireNonNull(resource, "resource");
initBits &= ~INIT_BIT_RESOURCE;
return this;
}
/**
* Initializes the value for the {@link RawCloudApplication#getSummary() summary} attribute.
* @param summary The value for summary
* @return {@code this} builder for use in a chained invocation
*/
public final Builder summary(SummaryApplicationResponse summary) {
this.summary = Objects.requireNonNull(summary, "summary");
initBits &= ~INIT_BIT_SUMMARY;
return this;
}
/**
* Initializes the value for the {@link RawCloudApplication#getStack() stack} attribute.
* @param stack The value for stack
* @return {@code this} builder for use in a chained invocation
*/
public final Builder stack(Derivable stack) {
this.stack = Objects.requireNonNull(stack, "stack");
initBits &= ~INIT_BIT_STACK;
return this;
}
/**
* Initializes the value for the {@link RawCloudApplication#getSpace() space} attribute.
* @param space The value for space
* @return {@code this} builder for use in a chained invocation
*/
public final Builder space(Derivable space) {
this.space = Objects.requireNonNull(space, "space");
initBits &= ~INIT_BIT_SPACE;
return this;
}
/**
* Builds a new {@link ImmutableRawCloudApplication ImmutableRawCloudApplication}.
* @return An immutable instance of RawCloudApplication
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableRawCloudApplication build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableRawCloudApplication(resource, summary, stack, space);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_RESOURCE) != 0) attributes.add("resource");
if ((initBits & INIT_BIT_SUMMARY) != 0) attributes.add("summary");
if ((initBits & INIT_BIT_STACK) != 0) attributes.add("stack");
if ((initBits & INIT_BIT_SPACE) != 0) attributes.add("space");
return "Cannot build RawCloudApplication, some of required attributes are not set " + attributes;
}
}
}