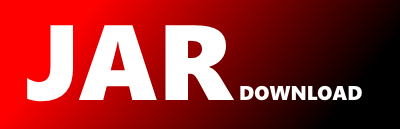
org.cloudfoundry.client.lib.domain.ImmutableCloudBuild Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.domain;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.Objects;
import java.util.UUID;
import org.cloudfoundry.client.lib.domain.annotation.Nullable;
import org.cloudfoundry.client.v3.Metadata;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link CloudBuild}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudBuild.builder()}.
*/
@Generated(from = "CloudBuild", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableCloudBuild extends CloudBuild {
private final @Nullable String name;
private final @Nullable CloudMetadata metadata;
private final @Nullable Metadata v3Metadata;
private final @Nullable CloudBuild.State state;
private final @Nullable CloudBuild.CreatedBy createdBy;
private final @Nullable DropletInfo dropletInfo;
private final @Nullable CloudBuild.PackageInfo packageInfo;
private final @Nullable String error;
private ImmutableCloudBuild(
@Nullable String name,
@Nullable CloudMetadata metadata,
@Nullable Metadata v3Metadata,
@Nullable CloudBuild.State state,
@Nullable CloudBuild.CreatedBy createdBy,
@Nullable DropletInfo dropletInfo,
@Nullable CloudBuild.PackageInfo packageInfo,
@Nullable String error) {
this.name = name;
this.metadata = metadata;
this.v3Metadata = v3Metadata;
this.state = state;
this.createdBy = createdBy;
this.dropletInfo = dropletInfo;
this.packageInfo = packageInfo;
this.error = error;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public @Nullable String getName() {
return name;
}
/**
* @return The value of the {@code metadata} attribute
*/
@JsonProperty("metadata")
@Override
public @Nullable CloudMetadata getMetadata() {
return metadata;
}
/**
* @return The value of the {@code v3Metadata} attribute
*/
@JsonProperty("v3Metadata")
@Override
public @Nullable Metadata getV3Metadata() {
return v3Metadata;
}
/**
* @return The value of the {@code state} attribute
*/
@JsonProperty("state")
@Override
public @Nullable CloudBuild.State getState() {
return state;
}
/**
* @return The value of the {@code createdBy} attribute
*/
@JsonProperty("createdBy")
@Override
public @Nullable CloudBuild.CreatedBy getCreatedBy() {
return createdBy;
}
/**
* @return The value of the {@code dropletInfo} attribute
*/
@JsonProperty("dropletInfo")
@Override
public @Nullable DropletInfo getDropletInfo() {
return dropletInfo;
}
/**
* @return The value of the {@code packageInfo} attribute
*/
@JsonProperty("packageInfo")
@Override
public @Nullable CloudBuild.PackageInfo getPackageInfo() {
return packageInfo;
}
/**
* @return The value of the {@code error} attribute
*/
@JsonProperty("error")
@Override
public @Nullable String getError() {
return error;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withName(@Nullable String value) {
if (Objects.equals(this.name, value)) return this;
return new ImmutableCloudBuild(
value,
this.metadata,
this.v3Metadata,
this.state,
this.createdBy,
this.dropletInfo,
this.packageInfo,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getMetadata() metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for metadata (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withMetadata(@Nullable CloudMetadata value) {
if (this.metadata == value) return this;
return new ImmutableCloudBuild(
this.name,
value,
this.v3Metadata,
this.state,
this.createdBy,
this.dropletInfo,
this.packageInfo,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getV3Metadata() v3Metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for v3Metadata (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withV3Metadata(@Nullable Metadata value) {
if (this.v3Metadata == value) return this;
return new ImmutableCloudBuild(
this.name,
this.metadata,
value,
this.state,
this.createdBy,
this.dropletInfo,
this.packageInfo,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getState() state} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for state (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withState(@Nullable CloudBuild.State value) {
if (this.state == value) return this;
if (Objects.equals(this.state, value)) return this;
return new ImmutableCloudBuild(
this.name,
this.metadata,
this.v3Metadata,
value,
this.createdBy,
this.dropletInfo,
this.packageInfo,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getCreatedBy() createdBy} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for createdBy (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withCreatedBy(@Nullable CloudBuild.CreatedBy value) {
if (this.createdBy == value) return this;
return new ImmutableCloudBuild(
this.name,
this.metadata,
this.v3Metadata,
this.state,
value,
this.dropletInfo,
this.packageInfo,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getDropletInfo() dropletInfo} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dropletInfo (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withDropletInfo(@Nullable DropletInfo value) {
if (this.dropletInfo == value) return this;
return new ImmutableCloudBuild(
this.name,
this.metadata,
this.v3Metadata,
this.state,
this.createdBy,
value,
this.packageInfo,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getPackageInfo() packageInfo} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for packageInfo (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withPackageInfo(@Nullable CloudBuild.PackageInfo value) {
if (this.packageInfo == value) return this;
return new ImmutableCloudBuild(
this.name,
this.metadata,
this.v3Metadata,
this.state,
this.createdBy,
this.dropletInfo,
value,
this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild#getError() error} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for error (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild withError(@Nullable String value) {
if (Objects.equals(this.error, value)) return this;
return new ImmutableCloudBuild(
this.name,
this.metadata,
this.v3Metadata,
this.state,
this.createdBy,
this.dropletInfo,
this.packageInfo,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableCloudBuild} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudBuild
&& equalTo((ImmutableCloudBuild) another);
}
private boolean equalTo(ImmutableCloudBuild another) {
return Objects.equals(name, another.name)
&& Objects.equals(metadata, another.metadata)
&& Objects.equals(v3Metadata, another.v3Metadata)
&& Objects.equals(state, another.state)
&& Objects.equals(createdBy, another.createdBy)
&& Objects.equals(dropletInfo, another.dropletInfo)
&& Objects.equals(packageInfo, another.packageInfo)
&& Objects.equals(error, another.error);
}
/**
* Computes a hash code from attributes: {@code name}, {@code metadata}, {@code v3Metadata}, {@code state}, {@code createdBy}, {@code dropletInfo}, {@code packageInfo}, {@code error}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + Objects.hashCode(metadata);
h += (h << 5) + Objects.hashCode(v3Metadata);
h += (h << 5) + Objects.hashCode(state);
h += (h << 5) + Objects.hashCode(createdBy);
h += (h << 5) + Objects.hashCode(dropletInfo);
h += (h << 5) + Objects.hashCode(packageInfo);
h += (h << 5) + Objects.hashCode(error);
return h;
}
/**
* Prints the immutable value {@code CloudBuild} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CloudBuild{"
+ "name=" + name
+ ", metadata=" + metadata
+ ", v3Metadata=" + v3Metadata
+ ", state=" + state
+ ", createdBy=" + createdBy
+ ", dropletInfo=" + dropletInfo
+ ", packageInfo=" + packageInfo
+ ", error=" + error
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudBuild", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends CloudBuild {
String name;
CloudMetadata metadata;
Metadata v3Metadata;
CloudBuild.State state;
CloudBuild.CreatedBy createdBy;
DropletInfo dropletInfo;
CloudBuild.PackageInfo packageInfo;
String error;
@JsonProperty("name")
public void setName(@Nullable String name) {
this.name = name;
}
@JsonProperty("metadata")
public void setMetadata(@Nullable CloudMetadata metadata) {
this.metadata = metadata;
}
@JsonProperty("v3Metadata")
public void setV3Metadata(@Nullable Metadata v3Metadata) {
this.v3Metadata = v3Metadata;
}
@JsonProperty("state")
public void setState(@Nullable CloudBuild.State state) {
this.state = state;
}
@JsonProperty("createdBy")
public void setCreatedBy(@Nullable CloudBuild.CreatedBy createdBy) {
this.createdBy = createdBy;
}
@JsonProperty("dropletInfo")
public void setDropletInfo(@Nullable DropletInfo dropletInfo) {
this.dropletInfo = dropletInfo;
}
@JsonProperty("packageInfo")
public void setPackageInfo(@Nullable CloudBuild.PackageInfo packageInfo) {
this.packageInfo = packageInfo;
}
@JsonProperty("error")
public void setError(@Nullable String error) {
this.error = error;
}
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public CloudMetadata getMetadata() { throw new UnsupportedOperationException(); }
@Override
public Metadata getV3Metadata() { throw new UnsupportedOperationException(); }
@Override
public CloudBuild.State getState() { throw new UnsupportedOperationException(); }
@Override
public CloudBuild.CreatedBy getCreatedBy() { throw new UnsupportedOperationException(); }
@Override
public DropletInfo getDropletInfo() { throw new UnsupportedOperationException(); }
@Override
public CloudBuild.PackageInfo getPackageInfo() { throw new UnsupportedOperationException(); }
@Override
public String getError() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudBuild fromJson(Json json) {
ImmutableCloudBuild.Builder builder = ImmutableCloudBuild.builder();
if (json.name != null) {
builder.name(json.name);
}
if (json.metadata != null) {
builder.metadata(json.metadata);
}
if (json.v3Metadata != null) {
builder.v3Metadata(json.v3Metadata);
}
if (json.state != null) {
builder.state(json.state);
}
if (json.createdBy != null) {
builder.createdBy(json.createdBy);
}
if (json.dropletInfo != null) {
builder.dropletInfo(json.dropletInfo);
}
if (json.packageInfo != null) {
builder.packageInfo(json.packageInfo);
}
if (json.error != null) {
builder.error(json.error);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link CloudBuild} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CloudBuild instance
*/
public static ImmutableCloudBuild copyOf(CloudBuild instance) {
if (instance instanceof ImmutableCloudBuild) {
return (ImmutableCloudBuild) instance;
}
return ImmutableCloudBuild.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudBuild ImmutableCloudBuild}.
*
* ImmutableCloudBuild.builder()
* .name(String | null) // nullable {@link CloudBuild#getName() name}
* .metadata(org.cloudfoundry.client.lib.domain.CloudMetadata | null) // nullable {@link CloudBuild#getMetadata() metadata}
* .v3Metadata(org.cloudfoundry.client.v3.Metadata | null) // nullable {@link CloudBuild#getV3Metadata() v3Metadata}
* .state(org.cloudfoundry.client.lib.domain.CloudBuild.State | null) // nullable {@link CloudBuild#getState() state}
* .createdBy(org.cloudfoundry.client.lib.domain.CloudBuild.CreatedBy | null) // nullable {@link CloudBuild#getCreatedBy() createdBy}
* .dropletInfo(org.cloudfoundry.client.lib.domain.DropletInfo | null) // nullable {@link CloudBuild#getDropletInfo() dropletInfo}
* .packageInfo(org.cloudfoundry.client.lib.domain.CloudBuild.PackageInfo | null) // nullable {@link CloudBuild#getPackageInfo() packageInfo}
* .error(String | null) // nullable {@link CloudBuild#getError() error}
* .build();
*
* @return A new ImmutableCloudBuild builder
*/
public static ImmutableCloudBuild.Builder builder() {
return new ImmutableCloudBuild.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudBuild ImmutableCloudBuild}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudBuild", generator = "Immutables")
public static final class Builder {
private String name;
private CloudMetadata metadata;
private Metadata v3Metadata;
private CloudBuild.State state;
private CloudBuild.CreatedBy createdBy;
private DropletInfo dropletInfo;
private CloudBuild.PackageInfo packageInfo;
private String error;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.client.lib.domain.CloudBuild} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudBuild instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.client.lib.domain.CloudEntity} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudEntity instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof CloudBuild) {
CloudBuild instance = (CloudBuild) object;
@Nullable DropletInfo dropletInfoValue = instance.getDropletInfo();
if (dropletInfoValue != null) {
dropletInfo(dropletInfoValue);
}
@Nullable CloudBuild.State stateValue = instance.getState();
if (stateValue != null) {
state(stateValue);
}
@Nullable CloudBuild.PackageInfo packageInfoValue = instance.getPackageInfo();
if (packageInfoValue != null) {
packageInfo(packageInfoValue);
}
@Nullable String errorValue = instance.getError();
if (errorValue != null) {
error(errorValue);
}
@Nullable CloudBuild.CreatedBy createdByValue = instance.getCreatedBy();
if (createdByValue != null) {
createdBy(createdByValue);
}
}
if (object instanceof CloudEntity) {
CloudEntity instance = (CloudEntity) object;
@Nullable String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
@Nullable Metadata v3MetadataValue = instance.getV3Metadata();
if (v3MetadataValue != null) {
v3Metadata(v3MetadataValue);
}
@Nullable CloudMetadata metadataValue = instance.getMetadata();
if (metadataValue != null) {
metadata(metadataValue);
}
}
}
/**
* Initializes the value for the {@link CloudBuild#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("name")
public final Builder name(@Nullable String name) {
this.name = name;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getMetadata() metadata} attribute.
* @param metadata The value for metadata (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("metadata")
public final Builder metadata(@Nullable CloudMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getV3Metadata() v3Metadata} attribute.
* @param v3Metadata The value for v3Metadata (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("v3Metadata")
public final Builder v3Metadata(@Nullable Metadata v3Metadata) {
this.v3Metadata = v3Metadata;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getState() state} attribute.
* @param state The value for state (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("state")
public final Builder state(@Nullable CloudBuild.State state) {
this.state = state;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getCreatedBy() createdBy} attribute.
* @param createdBy The value for createdBy (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("createdBy")
public final Builder createdBy(@Nullable CloudBuild.CreatedBy createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getDropletInfo() dropletInfo} attribute.
* @param dropletInfo The value for dropletInfo (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("dropletInfo")
public final Builder dropletInfo(@Nullable DropletInfo dropletInfo) {
this.dropletInfo = dropletInfo;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getPackageInfo() packageInfo} attribute.
* @param packageInfo The value for packageInfo (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("packageInfo")
public final Builder packageInfo(@Nullable CloudBuild.PackageInfo packageInfo) {
this.packageInfo = packageInfo;
return this;
}
/**
* Initializes the value for the {@link CloudBuild#getError() error} attribute.
* @param error The value for error (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("error")
public final Builder error(@Nullable String error) {
this.error = error;
return this;
}
/**
* Builds a new {@link ImmutableCloudBuild ImmutableCloudBuild}.
* @return An immutable instance of CloudBuild
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudBuild build() {
return new ImmutableCloudBuild(name, metadata, v3Metadata, state, createdBy, dropletInfo, packageInfo, error);
}
}
/**
* Immutable implementation of {@link CloudBuild.PackageInfo}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudBuild.ImmutablePackageInfo.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableCloudBuild.ImmutablePackageInfo.of()}.
*/
@Generated(from = "CloudBuild.PackageInfo", generator = "Immutables")
public static final class ImmutablePackageInfo implements CloudBuild.PackageInfo {
private final @Nullable UUID guid;
private ImmutablePackageInfo(@Nullable UUID guid) {
this.guid = guid;
}
/**
* @return The value of the {@code guid} attribute
*/
@JsonProperty("guid")
@Override
public @Nullable UUID getGuid() {
return guid;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild.PackageInfo#getGuid() guid} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for guid (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild.ImmutablePackageInfo withGuid(@Nullable UUID value) {
if (this.guid == value) return this;
return new ImmutableCloudBuild.ImmutablePackageInfo(value);
}
/**
* This instance is equal to all instances of {@code ImmutablePackageInfo} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudBuild.ImmutablePackageInfo
&& equalTo((ImmutableCloudBuild.ImmutablePackageInfo) another);
}
private boolean equalTo(ImmutableCloudBuild.ImmutablePackageInfo another) {
return Objects.equals(guid, another.guid);
}
/**
* Computes a hash code from attributes: {@code guid}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(guid);
return h;
}
/**
* Prints the immutable value {@code PackageInfo} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "PackageInfo{"
+ "guid=" + guid
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudBuild.PackageInfo", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements CloudBuild.PackageInfo {
UUID guid;
@JsonProperty("guid")
public void setGuid(@Nullable UUID guid) {
this.guid = guid;
}
@Override
public UUID getGuid() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudBuild.ImmutablePackageInfo fromJson(Json json) {
ImmutableCloudBuild.ImmutablePackageInfo.Builder builder = ImmutableCloudBuild.ImmutablePackageInfo.builder();
if (json.guid != null) {
builder.guid(json.guid);
}
return builder.build();
}
/**
* Construct a new immutable {@code PackageInfo} instance.
* @param guid The value for the {@code guid} attribute
* @return An immutable PackageInfo instance
*/
public static ImmutableCloudBuild.ImmutablePackageInfo of(@Nullable UUID guid) {
return new ImmutableCloudBuild.ImmutablePackageInfo(guid);
}
/**
* Creates an immutable copy of a {@link CloudBuild.PackageInfo} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable PackageInfo instance
*/
public static ImmutableCloudBuild.ImmutablePackageInfo copyOf(CloudBuild.PackageInfo instance) {
if (instance instanceof ImmutableCloudBuild.ImmutablePackageInfo) {
return (ImmutableCloudBuild.ImmutablePackageInfo) instance;
}
return ImmutableCloudBuild.ImmutablePackageInfo.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudBuild.ImmutablePackageInfo ImmutablePackageInfo}.
*
* ImmutableCloudBuild.ImmutablePackageInfo.builder()
* .guid(UUID | null) // nullable {@link CloudBuild.PackageInfo#getGuid() guid}
* .build();
*
* @return A new ImmutablePackageInfo builder
*/
public static ImmutableCloudBuild.ImmutablePackageInfo.Builder builder() {
return new ImmutableCloudBuild.ImmutablePackageInfo.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudBuild.ImmutablePackageInfo ImmutablePackageInfo}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudBuild.PackageInfo", generator = "Immutables")
public static final class Builder {
private UUID guid;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code PackageInfo} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudBuild.PackageInfo instance) {
Objects.requireNonNull(instance, "instance");
@Nullable UUID guidValue = instance.getGuid();
if (guidValue != null) {
guid(guidValue);
}
return this;
}
/**
* Initializes the value for the {@link CloudBuild.PackageInfo#getGuid() guid} attribute.
* @param guid The value for guid (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("guid")
public final Builder guid(@Nullable UUID guid) {
this.guid = guid;
return this;
}
/**
* Builds a new {@link ImmutableCloudBuild.ImmutablePackageInfo ImmutablePackageInfo}.
* @return An immutable instance of PackageInfo
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudBuild.ImmutablePackageInfo build() {
return new ImmutableCloudBuild.ImmutablePackageInfo(guid);
}
}
}
/**
* Immutable implementation of {@link CloudBuild.CreatedBy}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudBuild.ImmutableCreatedBy.builder()}.
*/
@Generated(from = "CloudBuild.CreatedBy", generator = "Immutables")
public static final class ImmutableCreatedBy implements CloudBuild.CreatedBy {
private final @Nullable UUID guid;
private final @Nullable String name;
private ImmutableCreatedBy(
@Nullable UUID guid,
@Nullable String name) {
this.guid = guid;
this.name = name;
}
/**
* @return The value of the {@code guid} attribute
*/
@JsonProperty("guid")
@Override
public @Nullable UUID getGuid() {
return guid;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public @Nullable String getName() {
return name;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild.CreatedBy#getGuid() guid} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for guid (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild.ImmutableCreatedBy withGuid(@Nullable UUID value) {
if (this.guid == value) return this;
return new ImmutableCloudBuild.ImmutableCreatedBy(value, this.name);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudBuild.CreatedBy#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudBuild.ImmutableCreatedBy withName(@Nullable String value) {
if (Objects.equals(this.name, value)) return this;
return new ImmutableCloudBuild.ImmutableCreatedBy(this.guid, value);
}
/**
* This instance is equal to all instances of {@code ImmutableCreatedBy} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudBuild.ImmutableCreatedBy
&& equalTo((ImmutableCloudBuild.ImmutableCreatedBy) another);
}
private boolean equalTo(ImmutableCloudBuild.ImmutableCreatedBy another) {
return Objects.equals(guid, another.guid)
&& Objects.equals(name, another.name);
}
/**
* Computes a hash code from attributes: {@code guid}, {@code name}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(guid);
h += (h << 5) + Objects.hashCode(name);
return h;
}
/**
* Prints the immutable value {@code CreatedBy} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CreatedBy{"
+ "guid=" + guid
+ ", name=" + name
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudBuild.CreatedBy", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements CloudBuild.CreatedBy {
UUID guid;
String name;
@JsonProperty("guid")
public void setGuid(@Nullable UUID guid) {
this.guid = guid;
}
@JsonProperty("name")
public void setName(@Nullable String name) {
this.name = name;
}
@Override
public UUID getGuid() { throw new UnsupportedOperationException(); }
@Override
public String getName() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudBuild.ImmutableCreatedBy fromJson(Json json) {
ImmutableCloudBuild.ImmutableCreatedBy.Builder builder = ImmutableCloudBuild.ImmutableCreatedBy.builder();
if (json.guid != null) {
builder.guid(json.guid);
}
if (json.name != null) {
builder.name(json.name);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link CloudBuild.CreatedBy} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CreatedBy instance
*/
public static ImmutableCloudBuild.ImmutableCreatedBy copyOf(CloudBuild.CreatedBy instance) {
if (instance instanceof ImmutableCloudBuild.ImmutableCreatedBy) {
return (ImmutableCloudBuild.ImmutableCreatedBy) instance;
}
return ImmutableCloudBuild.ImmutableCreatedBy.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudBuild.ImmutableCreatedBy ImmutableCreatedBy}.
*
* ImmutableCloudBuild.ImmutableCreatedBy.builder()
* .guid(UUID | null) // nullable {@link CloudBuild.CreatedBy#getGuid() guid}
* .name(String | null) // nullable {@link CloudBuild.CreatedBy#getName() name}
* .build();
*
* @return A new ImmutableCreatedBy builder
*/
public static ImmutableCloudBuild.ImmutableCreatedBy.Builder builder() {
return new ImmutableCloudBuild.ImmutableCreatedBy.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudBuild.ImmutableCreatedBy ImmutableCreatedBy}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudBuild.CreatedBy", generator = "Immutables")
public static final class Builder {
private UUID guid;
private String name;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CreatedBy} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudBuild.CreatedBy instance) {
Objects.requireNonNull(instance, "instance");
@Nullable UUID guidValue = instance.getGuid();
if (guidValue != null) {
guid(guidValue);
}
@Nullable String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
return this;
}
/**
* Initializes the value for the {@link CloudBuild.CreatedBy#getGuid() guid} attribute.
* @param guid The value for guid (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("guid")
public final Builder guid(@Nullable UUID guid) {
this.guid = guid;
return this;
}
/**
* Initializes the value for the {@link CloudBuild.CreatedBy#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("name")
public final Builder name(@Nullable String name) {
this.name = name;
return this;
}
/**
* Builds a new {@link ImmutableCloudBuild.ImmutableCreatedBy ImmutableCreatedBy}.
* @return An immutable instance of CreatedBy
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudBuild.ImmutableCreatedBy build() {
return new ImmutableCloudBuild.ImmutableCreatedBy(guid, name);
}
}
}
}