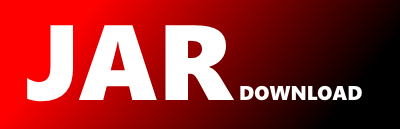
org.cloudfoundry.client.lib.domain.ImmutableCloudServiceOffering Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.domain;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.client.lib.domain.annotation.Nullable;
import org.cloudfoundry.client.v3.Metadata;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link CloudServiceOffering}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudServiceOffering.builder()}.
*/
@Generated(from = "CloudServiceOffering", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableCloudServiceOffering
extends CloudServiceOffering {
private final @Nullable String name;
private final @Nullable CloudMetadata metadata;
private final @Nullable Metadata v3Metadata;
private final @Nullable Boolean isActive;
private final @Nullable Boolean isBindable;
private final List servicePlans;
private final @Nullable String description;
private final @Nullable String docUrl;
private final @Nullable String extra;
private final @Nullable String infoUrl;
private final @Nullable String provider;
private final @Nullable String brokerName;
private final @Nullable String uniqueId;
private final @Nullable String url;
private final @Nullable String version;
private ImmutableCloudServiceOffering(
@Nullable String name,
@Nullable CloudMetadata metadata,
@Nullable Metadata v3Metadata,
@Nullable Boolean isActive,
@Nullable Boolean isBindable,
List servicePlans,
@Nullable String description,
@Nullable String docUrl,
@Nullable String extra,
@Nullable String infoUrl,
@Nullable String provider,
@Nullable String brokerName,
@Nullable String uniqueId,
@Nullable String url,
@Nullable String version) {
this.name = name;
this.metadata = metadata;
this.v3Metadata = v3Metadata;
this.isActive = isActive;
this.isBindable = isBindable;
this.servicePlans = servicePlans;
this.description = description;
this.docUrl = docUrl;
this.extra = extra;
this.infoUrl = infoUrl;
this.provider = provider;
this.brokerName = brokerName;
this.uniqueId = uniqueId;
this.url = url;
this.version = version;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public @Nullable String getName() {
return name;
}
/**
* @return The value of the {@code metadata} attribute
*/
@JsonProperty("metadata")
@Override
public @Nullable CloudMetadata getMetadata() {
return metadata;
}
/**
* @return The value of the {@code v3Metadata} attribute
*/
@JsonProperty("v3Metadata")
@Override
public @Nullable Metadata getV3Metadata() {
return v3Metadata;
}
/**
* @return The value of the {@code isActive} attribute
*/
@JsonProperty("isActive")
@Override
public @Nullable Boolean isActive() {
return isActive;
}
/**
* @return The value of the {@code isBindable} attribute
*/
@JsonProperty("isBindable")
@Override
public @Nullable Boolean isBindable() {
return isBindable;
}
/**
* @return The value of the {@code servicePlans} attribute
*/
@JsonProperty("servicePlans")
@Override
public List getServicePlans() {
return servicePlans;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public @Nullable String getDescription() {
return description;
}
/**
* @return The value of the {@code docUrl} attribute
*/
@JsonProperty("docUrl")
@Override
public @Nullable String getDocUrl() {
return docUrl;
}
/**
* @return The value of the {@code extra} attribute
*/
@JsonProperty("extra")
@Override
public @Nullable String getExtra() {
return extra;
}
/**
* @return The value of the {@code infoUrl} attribute
*/
@JsonProperty("infoUrl")
@Override
public @Nullable String getInfoUrl() {
return infoUrl;
}
/**
* @return The value of the {@code provider} attribute
*/
@JsonProperty("provider")
@Override
public @Nullable String getProvider() {
return provider;
}
/**
* @return The value of the {@code brokerName} attribute
*/
@JsonProperty("brokerName")
@Override
public @Nullable String getBrokerName() {
return brokerName;
}
/**
* @return The value of the {@code uniqueId} attribute
*/
@JsonProperty("uniqueId")
@Override
public @Nullable String getUniqueId() {
return uniqueId;
}
/**
* @return The value of the {@code url} attribute
*/
@JsonProperty("url")
@Override
public @Nullable String getUrl() {
return url;
}
/**
* @return The value of the {@code version} attribute
*/
@JsonProperty("version")
@Override
public @Nullable String getVersion() {
return version;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withName(@Nullable String value) {
if (Objects.equals(this.name, value)) return this;
return new ImmutableCloudServiceOffering(
value,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getMetadata() metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for metadata (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withMetadata(@Nullable CloudMetadata value) {
if (this.metadata == value) return this;
return new ImmutableCloudServiceOffering(
this.name,
value,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getV3Metadata() v3Metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for v3Metadata (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withV3Metadata(@Nullable Metadata value) {
if (this.v3Metadata == value) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
value,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#isActive() isActive} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isActive (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withIsActive(@Nullable Boolean value) {
if (Objects.equals(this.isActive, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
value,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#isBindable() isBindable} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isBindable (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withIsBindable(@Nullable Boolean value) {
if (Objects.equals(this.isBindable, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
value,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CloudServiceOffering#getServicePlans() servicePlans}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudServiceOffering withServicePlans(CloudServicePlan... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
newValue,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CloudServiceOffering#getServicePlans() servicePlans}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of servicePlans elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudServiceOffering withServicePlans(Iterable extends CloudServicePlan> elements) {
if (this.servicePlans == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
newValue,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getDescription() description} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for description (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withDescription(@Nullable String value) {
if (Objects.equals(this.description, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
value,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getDocUrl() docUrl} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for docUrl (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withDocUrl(@Nullable String value) {
if (Objects.equals(this.docUrl, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
value,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getExtra() extra} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for extra (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withExtra(@Nullable String value) {
if (Objects.equals(this.extra, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
value,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getInfoUrl() infoUrl} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for infoUrl (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withInfoUrl(@Nullable String value) {
if (Objects.equals(this.infoUrl, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
value,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getProvider() provider} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for provider (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withProvider(@Nullable String value) {
if (Objects.equals(this.provider, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
value,
this.brokerName,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getBrokerName() brokerName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for brokerName (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withBrokerName(@Nullable String value) {
if (Objects.equals(this.brokerName, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
value,
this.uniqueId,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getUniqueId() uniqueId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for uniqueId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withUniqueId(@Nullable String value) {
if (Objects.equals(this.uniqueId, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
value,
this.url,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getUrl() url} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for url (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withUrl(@Nullable String value) {
if (Objects.equals(this.url, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
value,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudServiceOffering#getVersion() version} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for version (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudServiceOffering withVersion(@Nullable String value) {
if (Objects.equals(this.version, value)) return this;
return new ImmutableCloudServiceOffering(
this.name,
this.metadata,
this.v3Metadata,
this.isActive,
this.isBindable,
this.servicePlans,
this.description,
this.docUrl,
this.extra,
this.infoUrl,
this.provider,
this.brokerName,
this.uniqueId,
this.url,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableCloudServiceOffering} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudServiceOffering
&& equalTo((ImmutableCloudServiceOffering) another);
}
private boolean equalTo(ImmutableCloudServiceOffering another) {
return Objects.equals(name, another.name)
&& Objects.equals(metadata, another.metadata)
&& Objects.equals(v3Metadata, another.v3Metadata)
&& Objects.equals(isActive, another.isActive)
&& Objects.equals(isBindable, another.isBindable)
&& servicePlans.equals(another.servicePlans)
&& Objects.equals(description, another.description)
&& Objects.equals(docUrl, another.docUrl)
&& Objects.equals(extra, another.extra)
&& Objects.equals(infoUrl, another.infoUrl)
&& Objects.equals(provider, another.provider)
&& Objects.equals(brokerName, another.brokerName)
&& Objects.equals(uniqueId, another.uniqueId)
&& Objects.equals(url, another.url)
&& Objects.equals(version, another.version);
}
/**
* Computes a hash code from attributes: {@code name}, {@code metadata}, {@code v3Metadata}, {@code isActive}, {@code isBindable}, {@code servicePlans}, {@code description}, {@code docUrl}, {@code extra}, {@code infoUrl}, {@code provider}, {@code brokerName}, {@code uniqueId}, {@code url}, {@code version}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + Objects.hashCode(metadata);
h += (h << 5) + Objects.hashCode(v3Metadata);
h += (h << 5) + Objects.hashCode(isActive);
h += (h << 5) + Objects.hashCode(isBindable);
h += (h << 5) + servicePlans.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + Objects.hashCode(docUrl);
h += (h << 5) + Objects.hashCode(extra);
h += (h << 5) + Objects.hashCode(infoUrl);
h += (h << 5) + Objects.hashCode(provider);
h += (h << 5) + Objects.hashCode(brokerName);
h += (h << 5) + Objects.hashCode(uniqueId);
h += (h << 5) + Objects.hashCode(url);
h += (h << 5) + Objects.hashCode(version);
return h;
}
/**
* Prints the immutable value {@code CloudServiceOffering} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CloudServiceOffering{"
+ "name=" + name
+ ", metadata=" + metadata
+ ", v3Metadata=" + v3Metadata
+ ", isActive=" + isActive
+ ", isBindable=" + isBindable
+ ", servicePlans=" + servicePlans
+ ", description=" + description
+ ", docUrl=" + docUrl
+ ", extra=" + extra
+ ", infoUrl=" + infoUrl
+ ", provider=" + provider
+ ", brokerName=" + brokerName
+ ", uniqueId=" + uniqueId
+ ", url=" + url
+ ", version=" + version
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudServiceOffering", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends CloudServiceOffering {
String name;
CloudMetadata metadata;
Metadata v3Metadata;
Boolean isActive;
Boolean isBindable;
List servicePlans = Collections.emptyList();
String description;
String docUrl;
String extra;
String infoUrl;
String provider;
String brokerName;
String uniqueId;
String url;
String version;
@JsonProperty("name")
public void setName(@Nullable String name) {
this.name = name;
}
@JsonProperty("metadata")
public void setMetadata(@Nullable CloudMetadata metadata) {
this.metadata = metadata;
}
@JsonProperty("v3Metadata")
public void setV3Metadata(@Nullable Metadata v3Metadata) {
this.v3Metadata = v3Metadata;
}
@JsonProperty("isActive")
public void setIsActive(@Nullable Boolean isActive) {
this.isActive = isActive;
}
@JsonProperty("isBindable")
public void setIsBindable(@Nullable Boolean isBindable) {
this.isBindable = isBindable;
}
@JsonProperty("servicePlans")
public void setServicePlans(List servicePlans) {
this.servicePlans = servicePlans;
}
@JsonProperty("description")
public void setDescription(@Nullable String description) {
this.description = description;
}
@JsonProperty("docUrl")
public void setDocUrl(@Nullable String docUrl) {
this.docUrl = docUrl;
}
@JsonProperty("extra")
public void setExtra(@Nullable String extra) {
this.extra = extra;
}
@JsonProperty("infoUrl")
public void setInfoUrl(@Nullable String infoUrl) {
this.infoUrl = infoUrl;
}
@JsonProperty("provider")
public void setProvider(@Nullable String provider) {
this.provider = provider;
}
@JsonProperty("brokerName")
public void setBrokerName(@Nullable String brokerName) {
this.brokerName = brokerName;
}
@JsonProperty("uniqueId")
public void setUniqueId(@Nullable String uniqueId) {
this.uniqueId = uniqueId;
}
@JsonProperty("url")
public void setUrl(@Nullable String url) {
this.url = url;
}
@JsonProperty("version")
public void setVersion(@Nullable String version) {
this.version = version;
}
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public CloudMetadata getMetadata() { throw new UnsupportedOperationException(); }
@Override
public Metadata getV3Metadata() { throw new UnsupportedOperationException(); }
@Override
public Boolean isActive() { throw new UnsupportedOperationException(); }
@Override
public Boolean isBindable() { throw new UnsupportedOperationException(); }
@Override
public List getServicePlans() { throw new UnsupportedOperationException(); }
@Override
public String getDescription() { throw new UnsupportedOperationException(); }
@Override
public String getDocUrl() { throw new UnsupportedOperationException(); }
@Override
public String getExtra() { throw new UnsupportedOperationException(); }
@Override
public String getInfoUrl() { throw new UnsupportedOperationException(); }
@Override
public String getProvider() { throw new UnsupportedOperationException(); }
@Override
public String getBrokerName() { throw new UnsupportedOperationException(); }
@Override
public String getUniqueId() { throw new UnsupportedOperationException(); }
@Override
public String getUrl() { throw new UnsupportedOperationException(); }
@Override
public String getVersion() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudServiceOffering fromJson(Json json) {
ImmutableCloudServiceOffering.Builder builder = ImmutableCloudServiceOffering.builder();
if (json.name != null) {
builder.name(json.name);
}
if (json.metadata != null) {
builder.metadata(json.metadata);
}
if (json.v3Metadata != null) {
builder.v3Metadata(json.v3Metadata);
}
if (json.isActive != null) {
builder.isActive(json.isActive);
}
if (json.isBindable != null) {
builder.isBindable(json.isBindable);
}
if (json.servicePlans != null) {
builder.addAllServicePlans(json.servicePlans);
}
if (json.description != null) {
builder.description(json.description);
}
if (json.docUrl != null) {
builder.docUrl(json.docUrl);
}
if (json.extra != null) {
builder.extra(json.extra);
}
if (json.infoUrl != null) {
builder.infoUrl(json.infoUrl);
}
if (json.provider != null) {
builder.provider(json.provider);
}
if (json.brokerName != null) {
builder.brokerName(json.brokerName);
}
if (json.uniqueId != null) {
builder.uniqueId(json.uniqueId);
}
if (json.url != null) {
builder.url(json.url);
}
if (json.version != null) {
builder.version(json.version);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link CloudServiceOffering} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CloudServiceOffering instance
*/
public static ImmutableCloudServiceOffering copyOf(CloudServiceOffering instance) {
if (instance instanceof ImmutableCloudServiceOffering) {
return (ImmutableCloudServiceOffering) instance;
}
return ImmutableCloudServiceOffering.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudServiceOffering ImmutableCloudServiceOffering}.
*
* ImmutableCloudServiceOffering.builder()
* .name(String | null) // nullable {@link CloudServiceOffering#getName() name}
* .metadata(org.cloudfoundry.client.lib.domain.CloudMetadata | null) // nullable {@link CloudServiceOffering#getMetadata() metadata}
* .v3Metadata(org.cloudfoundry.client.v3.Metadata | null) // nullable {@link CloudServiceOffering#getV3Metadata() v3Metadata}
* .isActive(Boolean | null) // nullable {@link CloudServiceOffering#isActive() isActive}
* .isBindable(Boolean | null) // nullable {@link CloudServiceOffering#isBindable() isBindable}
* .addServicePlan|addAllServicePlans(org.cloudfoundry.client.lib.domain.CloudServicePlan) // {@link CloudServiceOffering#getServicePlans() servicePlans} elements
* .description(String | null) // nullable {@link CloudServiceOffering#getDescription() description}
* .docUrl(String | null) // nullable {@link CloudServiceOffering#getDocUrl() docUrl}
* .extra(String | null) // nullable {@link CloudServiceOffering#getExtra() extra}
* .infoUrl(String | null) // nullable {@link CloudServiceOffering#getInfoUrl() infoUrl}
* .provider(String | null) // nullable {@link CloudServiceOffering#getProvider() provider}
* .brokerName(String | null) // nullable {@link CloudServiceOffering#getBrokerName() brokerName}
* .uniqueId(String | null) // nullable {@link CloudServiceOffering#getUniqueId() uniqueId}
* .url(String | null) // nullable {@link CloudServiceOffering#getUrl() url}
* .version(String | null) // nullable {@link CloudServiceOffering#getVersion() version}
* .build();
*
* @return A new ImmutableCloudServiceOffering builder
*/
public static ImmutableCloudServiceOffering.Builder builder() {
return new ImmutableCloudServiceOffering.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudServiceOffering ImmutableCloudServiceOffering}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudServiceOffering", generator = "Immutables")
public static final class Builder {
private String name;
private CloudMetadata metadata;
private Metadata v3Metadata;
private Boolean isActive;
private Boolean isBindable;
private List servicePlans = new ArrayList();
private String description;
private String docUrl;
private String extra;
private String infoUrl;
private String provider;
private String brokerName;
private String uniqueId;
private String url;
private String version;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.client.lib.domain.CloudServiceOffering} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudServiceOffering instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.client.lib.domain.CloudEntity} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudEntity instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof CloudServiceOffering) {
CloudServiceOffering instance = (CloudServiceOffering) object;
@Nullable String infoUrlValue = instance.getInfoUrl();
if (infoUrlValue != null) {
infoUrl(infoUrlValue);
}
@Nullable String providerValue = instance.getProvider();
if (providerValue != null) {
provider(providerValue);
}
@Nullable String extraValue = instance.getExtra();
if (extraValue != null) {
extra(extraValue);
}
@Nullable String docUrlValue = instance.getDocUrl();
if (docUrlValue != null) {
docUrl(docUrlValue);
}
@Nullable String descriptionValue = instance.getDescription();
if (descriptionValue != null) {
description(descriptionValue);
}
addAllServicePlans(instance.getServicePlans());
@Nullable Boolean isActiveValue = instance.isActive();
if (isActiveValue != null) {
isActive(isActiveValue);
}
@Nullable String brokerNameValue = instance.getBrokerName();
if (brokerNameValue != null) {
brokerName(brokerNameValue);
}
@Nullable String versionValue = instance.getVersion();
if (versionValue != null) {
version(versionValue);
}
@Nullable Boolean isBindableValue = instance.isBindable();
if (isBindableValue != null) {
isBindable(isBindableValue);
}
@Nullable String urlValue = instance.getUrl();
if (urlValue != null) {
url(urlValue);
}
@Nullable String uniqueIdValue = instance.getUniqueId();
if (uniqueIdValue != null) {
uniqueId(uniqueIdValue);
}
}
if (object instanceof CloudEntity) {
CloudEntity instance = (CloudEntity) object;
@Nullable Metadata v3MetadataValue = instance.getV3Metadata();
if (v3MetadataValue != null) {
v3Metadata(v3MetadataValue);
}
@Nullable String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
@Nullable CloudMetadata metadataValue = instance.getMetadata();
if (metadataValue != null) {
metadata(metadataValue);
}
}
}
/**
* Initializes the value for the {@link CloudServiceOffering#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("name")
public final Builder name(@Nullable String name) {
this.name = name;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getMetadata() metadata} attribute.
* @param metadata The value for metadata (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("metadata")
public final Builder metadata(@Nullable CloudMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getV3Metadata() v3Metadata} attribute.
* @param v3Metadata The value for v3Metadata (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("v3Metadata")
public final Builder v3Metadata(@Nullable Metadata v3Metadata) {
this.v3Metadata = v3Metadata;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#isActive() isActive} attribute.
* @param isActive The value for isActive (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("isActive")
public final Builder isActive(@Nullable Boolean isActive) {
this.isActive = isActive;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#isBindable() isBindable} attribute.
* @param isBindable The value for isBindable (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("isBindable")
public final Builder isBindable(@Nullable Boolean isBindable) {
this.isBindable = isBindable;
return this;
}
/**
* Adds one element to {@link CloudServiceOffering#getServicePlans() servicePlans} list.
* @param element A servicePlans element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addServicePlan(CloudServicePlan element) {
this.servicePlans.add(Objects.requireNonNull(element, "servicePlans element"));
return this;
}
/**
* Adds elements to {@link CloudServiceOffering#getServicePlans() servicePlans} list.
* @param elements An array of servicePlans elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addServicePlans(CloudServicePlan... elements) {
for (CloudServicePlan element : elements) {
this.servicePlans.add(Objects.requireNonNull(element, "servicePlans element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link CloudServiceOffering#getServicePlans() servicePlans} list.
* @param elements An iterable of servicePlans elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("servicePlans")
public final Builder servicePlans(Iterable extends CloudServicePlan> elements) {
this.servicePlans.clear();
return addAllServicePlans(elements);
}
/**
* Adds elements to {@link CloudServiceOffering#getServicePlans() servicePlans} list.
* @param elements An iterable of servicePlans elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllServicePlans(Iterable extends CloudServicePlan> elements) {
for (CloudServicePlan element : elements) {
this.servicePlans.add(Objects.requireNonNull(element, "servicePlans element"));
}
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getDescription() description} attribute.
* @param description The value for description (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("description")
public final Builder description(@Nullable String description) {
this.description = description;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getDocUrl() docUrl} attribute.
* @param docUrl The value for docUrl (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("docUrl")
public final Builder docUrl(@Nullable String docUrl) {
this.docUrl = docUrl;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getExtra() extra} attribute.
* @param extra The value for extra (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("extra")
public final Builder extra(@Nullable String extra) {
this.extra = extra;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getInfoUrl() infoUrl} attribute.
* @param infoUrl The value for infoUrl (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("infoUrl")
public final Builder infoUrl(@Nullable String infoUrl) {
this.infoUrl = infoUrl;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getProvider() provider} attribute.
* @param provider The value for provider (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("provider")
public final Builder provider(@Nullable String provider) {
this.provider = provider;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getBrokerName() brokerName} attribute.
* @param brokerName The value for brokerName (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("brokerName")
public final Builder brokerName(@Nullable String brokerName) {
this.brokerName = brokerName;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getUniqueId() uniqueId} attribute.
* @param uniqueId The value for uniqueId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("uniqueId")
public final Builder uniqueId(@Nullable String uniqueId) {
this.uniqueId = uniqueId;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getUrl() url} attribute.
* @param url The value for url (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("url")
public final Builder url(@Nullable String url) {
this.url = url;
return this;
}
/**
* Initializes the value for the {@link CloudServiceOffering#getVersion() version} attribute.
* @param version The value for version (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("version")
public final Builder version(@Nullable String version) {
this.version = version;
return this;
}
/**
* Builds a new {@link ImmutableCloudServiceOffering ImmutableCloudServiceOffering}.
* @return An immutable instance of CloudServiceOffering
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudServiceOffering build() {
return new ImmutableCloudServiceOffering(
name,
metadata,
v3Metadata,
isActive,
isBindable,
createUnmodifiableList(true, servicePlans),
description,
docUrl,
extra,
infoUrl,
provider,
brokerName,
uniqueId,
url,
version);
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}