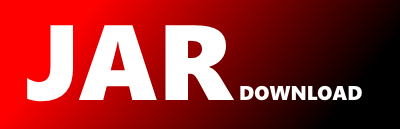
org.cloudfoundry.client.lib.domain.ImmutableCloudTask Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.domain;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.Objects;
import org.cloudfoundry.client.lib.domain.annotation.Nullable;
import org.cloudfoundry.client.v3.Metadata;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link CloudTask}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudTask.builder()}.
*/
@Generated(from = "CloudTask", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableCloudTask extends CloudTask {
private final @Nullable String name;
private final @Nullable CloudMetadata metadata;
private final @Nullable Metadata v3Metadata;
private final @Nullable String command;
private final @Nullable CloudTask.Limits limits;
private final @Nullable CloudTask.Result result;
private final @Nullable CloudTask.State state;
private ImmutableCloudTask(
@Nullable String name,
@Nullable CloudMetadata metadata,
@Nullable Metadata v3Metadata,
@Nullable String command,
@Nullable CloudTask.Limits limits,
@Nullable CloudTask.Result result,
@Nullable CloudTask.State state) {
this.name = name;
this.metadata = metadata;
this.v3Metadata = v3Metadata;
this.command = command;
this.limits = limits;
this.result = result;
this.state = state;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public @Nullable String getName() {
return name;
}
/**
* @return The value of the {@code metadata} attribute
*/
@JsonProperty("metadata")
@Override
public @Nullable CloudMetadata getMetadata() {
return metadata;
}
/**
* @return The value of the {@code v3Metadata} attribute
*/
@JsonProperty("v3Metadata")
@Override
public @Nullable Metadata getV3Metadata() {
return v3Metadata;
}
/**
* @return The value of the {@code command} attribute
*/
@JsonProperty("command")
@Override
public @Nullable String getCommand() {
return command;
}
/**
* @return The value of the {@code limits} attribute
*/
@JsonProperty("limits")
@Override
public @Nullable CloudTask.Limits getLimits() {
return limits;
}
/**
* @return The value of the {@code result} attribute
*/
@JsonProperty("result")
@Override
public @Nullable CloudTask.Result getResult() {
return result;
}
/**
* @return The value of the {@code state} attribute
*/
@JsonProperty("state")
@Override
public @Nullable CloudTask.State getState() {
return state;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withName(@Nullable String value) {
if (Objects.equals(this.name, value)) return this;
return new ImmutableCloudTask(value, this.metadata, this.v3Metadata, this.command, this.limits, this.result, this.state);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getMetadata() metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for metadata (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withMetadata(@Nullable CloudMetadata value) {
if (this.metadata == value) return this;
return new ImmutableCloudTask(this.name, value, this.v3Metadata, this.command, this.limits, this.result, this.state);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getV3Metadata() v3Metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for v3Metadata (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withV3Metadata(@Nullable Metadata value) {
if (this.v3Metadata == value) return this;
return new ImmutableCloudTask(this.name, this.metadata, value, this.command, this.limits, this.result, this.state);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getCommand() command} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for command (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withCommand(@Nullable String value) {
if (Objects.equals(this.command, value)) return this;
return new ImmutableCloudTask(this.name, this.metadata, this.v3Metadata, value, this.limits, this.result, this.state);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getLimits() limits} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for limits (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withLimits(@Nullable CloudTask.Limits value) {
if (this.limits == value) return this;
return new ImmutableCloudTask(this.name, this.metadata, this.v3Metadata, this.command, value, this.result, this.state);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getResult() result} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for result (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withResult(@Nullable CloudTask.Result value) {
if (this.result == value) return this;
return new ImmutableCloudTask(this.name, this.metadata, this.v3Metadata, this.command, this.limits, value, this.state);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask#getState() state} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for state (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask withState(@Nullable CloudTask.State value) {
if (this.state == value) return this;
if (Objects.equals(this.state, value)) return this;
return new ImmutableCloudTask(this.name, this.metadata, this.v3Metadata, this.command, this.limits, this.result, value);
}
/**
* This instance is equal to all instances of {@code ImmutableCloudTask} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudTask
&& equalTo((ImmutableCloudTask) another);
}
private boolean equalTo(ImmutableCloudTask another) {
return Objects.equals(name, another.name)
&& Objects.equals(metadata, another.metadata)
&& Objects.equals(v3Metadata, another.v3Metadata)
&& Objects.equals(command, another.command)
&& Objects.equals(limits, another.limits)
&& Objects.equals(result, another.result)
&& Objects.equals(state, another.state);
}
/**
* Computes a hash code from attributes: {@code name}, {@code metadata}, {@code v3Metadata}, {@code command}, {@code limits}, {@code result}, {@code state}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + Objects.hashCode(metadata);
h += (h << 5) + Objects.hashCode(v3Metadata);
h += (h << 5) + Objects.hashCode(command);
h += (h << 5) + Objects.hashCode(limits);
h += (h << 5) + Objects.hashCode(result);
h += (h << 5) + Objects.hashCode(state);
return h;
}
/**
* Prints the immutable value {@code CloudTask} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CloudTask{"
+ "name=" + name
+ ", metadata=" + metadata
+ ", v3Metadata=" + v3Metadata
+ ", command=" + command
+ ", limits=" + limits
+ ", result=" + result
+ ", state=" + state
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudTask", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends CloudTask {
String name;
CloudMetadata metadata;
Metadata v3Metadata;
String command;
CloudTask.Limits limits;
CloudTask.Result result;
CloudTask.State state;
@JsonProperty("name")
public void setName(@Nullable String name) {
this.name = name;
}
@JsonProperty("metadata")
public void setMetadata(@Nullable CloudMetadata metadata) {
this.metadata = metadata;
}
@JsonProperty("v3Metadata")
public void setV3Metadata(@Nullable Metadata v3Metadata) {
this.v3Metadata = v3Metadata;
}
@JsonProperty("command")
public void setCommand(@Nullable String command) {
this.command = command;
}
@JsonProperty("limits")
public void setLimits(@Nullable CloudTask.Limits limits) {
this.limits = limits;
}
@JsonProperty("result")
public void setResult(@Nullable CloudTask.Result result) {
this.result = result;
}
@JsonProperty("state")
public void setState(@Nullable CloudTask.State state) {
this.state = state;
}
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public CloudMetadata getMetadata() { throw new UnsupportedOperationException(); }
@Override
public Metadata getV3Metadata() { throw new UnsupportedOperationException(); }
@Override
public String getCommand() { throw new UnsupportedOperationException(); }
@Override
public CloudTask.Limits getLimits() { throw new UnsupportedOperationException(); }
@Override
public CloudTask.Result getResult() { throw new UnsupportedOperationException(); }
@Override
public CloudTask.State getState() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudTask fromJson(Json json) {
ImmutableCloudTask.Builder builder = ImmutableCloudTask.builder();
if (json.name != null) {
builder.name(json.name);
}
if (json.metadata != null) {
builder.metadata(json.metadata);
}
if (json.v3Metadata != null) {
builder.v3Metadata(json.v3Metadata);
}
if (json.command != null) {
builder.command(json.command);
}
if (json.limits != null) {
builder.limits(json.limits);
}
if (json.result != null) {
builder.result(json.result);
}
if (json.state != null) {
builder.state(json.state);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link CloudTask} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CloudTask instance
*/
public static ImmutableCloudTask copyOf(CloudTask instance) {
if (instance instanceof ImmutableCloudTask) {
return (ImmutableCloudTask) instance;
}
return ImmutableCloudTask.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudTask ImmutableCloudTask}.
*
* ImmutableCloudTask.builder()
* .name(String | null) // nullable {@link CloudTask#getName() name}
* .metadata(org.cloudfoundry.client.lib.domain.CloudMetadata | null) // nullable {@link CloudTask#getMetadata() metadata}
* .v3Metadata(org.cloudfoundry.client.v3.Metadata | null) // nullable {@link CloudTask#getV3Metadata() v3Metadata}
* .command(String | null) // nullable {@link CloudTask#getCommand() command}
* .limits(org.cloudfoundry.client.lib.domain.CloudTask.Limits | null) // nullable {@link CloudTask#getLimits() limits}
* .result(org.cloudfoundry.client.lib.domain.CloudTask.Result | null) // nullable {@link CloudTask#getResult() result}
* .state(org.cloudfoundry.client.lib.domain.CloudTask.State | null) // nullable {@link CloudTask#getState() state}
* .build();
*
* @return A new ImmutableCloudTask builder
*/
public static ImmutableCloudTask.Builder builder() {
return new ImmutableCloudTask.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudTask ImmutableCloudTask}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudTask", generator = "Immutables")
public static final class Builder {
private String name;
private CloudMetadata metadata;
private Metadata v3Metadata;
private String command;
private CloudTask.Limits limits;
private CloudTask.Result result;
private CloudTask.State state;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.client.lib.domain.CloudEntity} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudEntity instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.client.lib.domain.CloudTask} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudTask instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof CloudEntity) {
CloudEntity instance = (CloudEntity) object;
@Nullable String nameValue = instance.getName();
if (nameValue != null) {
name(nameValue);
}
@Nullable Metadata v3MetadataValue = instance.getV3Metadata();
if (v3MetadataValue != null) {
v3Metadata(v3MetadataValue);
}
@Nullable CloudMetadata metadataValue = instance.getMetadata();
if (metadataValue != null) {
metadata(metadataValue);
}
}
if (object instanceof CloudTask) {
CloudTask instance = (CloudTask) object;
@Nullable CloudTask.Result resultValue = instance.getResult();
if (resultValue != null) {
result(resultValue);
}
@Nullable CloudTask.State stateValue = instance.getState();
if (stateValue != null) {
state(stateValue);
}
@Nullable String commandValue = instance.getCommand();
if (commandValue != null) {
command(commandValue);
}
@Nullable CloudTask.Limits limitsValue = instance.getLimits();
if (limitsValue != null) {
limits(limitsValue);
}
}
}
/**
* Initializes the value for the {@link CloudTask#getName() name} attribute.
* @param name The value for name (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("name")
public final Builder name(@Nullable String name) {
this.name = name;
return this;
}
/**
* Initializes the value for the {@link CloudTask#getMetadata() metadata} attribute.
* @param metadata The value for metadata (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("metadata")
public final Builder metadata(@Nullable CloudMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Initializes the value for the {@link CloudTask#getV3Metadata() v3Metadata} attribute.
* @param v3Metadata The value for v3Metadata (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("v3Metadata")
public final Builder v3Metadata(@Nullable Metadata v3Metadata) {
this.v3Metadata = v3Metadata;
return this;
}
/**
* Initializes the value for the {@link CloudTask#getCommand() command} attribute.
* @param command The value for command (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("command")
public final Builder command(@Nullable String command) {
this.command = command;
return this;
}
/**
* Initializes the value for the {@link CloudTask#getLimits() limits} attribute.
* @param limits The value for limits (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("limits")
public final Builder limits(@Nullable CloudTask.Limits limits) {
this.limits = limits;
return this;
}
/**
* Initializes the value for the {@link CloudTask#getResult() result} attribute.
* @param result The value for result (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("result")
public final Builder result(@Nullable CloudTask.Result result) {
this.result = result;
return this;
}
/**
* Initializes the value for the {@link CloudTask#getState() state} attribute.
* @param state The value for state (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("state")
public final Builder state(@Nullable CloudTask.State state) {
this.state = state;
return this;
}
/**
* Builds a new {@link ImmutableCloudTask ImmutableCloudTask}.
* @return An immutable instance of CloudTask
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudTask build() {
return new ImmutableCloudTask(name, metadata, v3Metadata, command, limits, result, state);
}
}
/**
* Immutable implementation of {@link CloudTask.Result}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudTask.ImmutableResult.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableCloudTask.ImmutableResult.of()}.
*/
@Generated(from = "CloudTask.Result", generator = "Immutables")
public static final class ImmutableResult implements CloudTask.Result {
private final @Nullable String failureReason;
private ImmutableResult(@Nullable String failureReason) {
this.failureReason = failureReason;
}
/**
* @return The value of the {@code failureReason} attribute
*/
@JsonProperty("failureReason")
@Override
public @Nullable String getFailureReason() {
return failureReason;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask.Result#getFailureReason() failureReason} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for failureReason (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask.ImmutableResult withFailureReason(@Nullable String value) {
if (Objects.equals(this.failureReason, value)) return this;
return new ImmutableCloudTask.ImmutableResult(value);
}
/**
* This instance is equal to all instances of {@code ImmutableResult} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudTask.ImmutableResult
&& equalTo((ImmutableCloudTask.ImmutableResult) another);
}
private boolean equalTo(ImmutableCloudTask.ImmutableResult another) {
return Objects.equals(failureReason, another.failureReason);
}
/**
* Computes a hash code from attributes: {@code failureReason}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(failureReason);
return h;
}
/**
* Prints the immutable value {@code Result} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "Result{"
+ "failureReason=" + failureReason
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudTask.Result", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements CloudTask.Result {
String failureReason;
@JsonProperty("failureReason")
public void setFailureReason(@Nullable String failureReason) {
this.failureReason = failureReason;
}
@Override
public String getFailureReason() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudTask.ImmutableResult fromJson(Json json) {
ImmutableCloudTask.ImmutableResult.Builder builder = ImmutableCloudTask.ImmutableResult.builder();
if (json.failureReason != null) {
builder.failureReason(json.failureReason);
}
return builder.build();
}
/**
* Construct a new immutable {@code Result} instance.
* @param failureReason The value for the {@code failureReason} attribute
* @return An immutable Result instance
*/
public static ImmutableCloudTask.ImmutableResult of(@Nullable String failureReason) {
return new ImmutableCloudTask.ImmutableResult(failureReason);
}
/**
* Creates an immutable copy of a {@link CloudTask.Result} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Result instance
*/
public static ImmutableCloudTask.ImmutableResult copyOf(CloudTask.Result instance) {
if (instance instanceof ImmutableCloudTask.ImmutableResult) {
return (ImmutableCloudTask.ImmutableResult) instance;
}
return ImmutableCloudTask.ImmutableResult.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudTask.ImmutableResult ImmutableResult}.
*
* ImmutableCloudTask.ImmutableResult.builder()
* .failureReason(String | null) // nullable {@link CloudTask.Result#getFailureReason() failureReason}
* .build();
*
* @return A new ImmutableResult builder
*/
public static ImmutableCloudTask.ImmutableResult.Builder builder() {
return new ImmutableCloudTask.ImmutableResult.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudTask.ImmutableResult ImmutableResult}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudTask.Result", generator = "Immutables")
public static final class Builder {
private String failureReason;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Result} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudTask.Result instance) {
Objects.requireNonNull(instance, "instance");
@Nullable String failureReasonValue = instance.getFailureReason();
if (failureReasonValue != null) {
failureReason(failureReasonValue);
}
return this;
}
/**
* Initializes the value for the {@link CloudTask.Result#getFailureReason() failureReason} attribute.
* @param failureReason The value for failureReason (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("failureReason")
public final Builder failureReason(@Nullable String failureReason) {
this.failureReason = failureReason;
return this;
}
/**
* Builds a new {@link ImmutableCloudTask.ImmutableResult ImmutableResult}.
* @return An immutable instance of Result
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudTask.ImmutableResult build() {
return new ImmutableCloudTask.ImmutableResult(failureReason);
}
}
}
/**
* Immutable implementation of {@link CloudTask.Limits}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudTask.ImmutableLimits.builder()}.
*/
@Generated(from = "CloudTask.Limits", generator = "Immutables")
public static final class ImmutableLimits implements CloudTask.Limits {
private final @Nullable Integer disk;
private final @Nullable Integer memory;
private ImmutableLimits(
@Nullable Integer disk,
@Nullable Integer memory) {
this.disk = disk;
this.memory = memory;
}
/**
* @return The value of the {@code disk} attribute
*/
@JsonProperty("disk")
@Override
public @Nullable Integer getDisk() {
return disk;
}
/**
* @return The value of the {@code memory} attribute
*/
@JsonProperty("memory")
@Override
public @Nullable Integer getMemory() {
return memory;
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask.Limits#getDisk() disk} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for disk (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask.ImmutableLimits withDisk(@Nullable Integer value) {
if (Objects.equals(this.disk, value)) return this;
return new ImmutableCloudTask.ImmutableLimits(value, this.memory);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudTask.Limits#getMemory() memory} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for memory (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudTask.ImmutableLimits withMemory(@Nullable Integer value) {
if (Objects.equals(this.memory, value)) return this;
return new ImmutableCloudTask.ImmutableLimits(this.disk, value);
}
/**
* This instance is equal to all instances of {@code ImmutableLimits} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudTask.ImmutableLimits
&& equalTo((ImmutableCloudTask.ImmutableLimits) another);
}
private boolean equalTo(ImmutableCloudTask.ImmutableLimits another) {
return Objects.equals(disk, another.disk)
&& Objects.equals(memory, another.memory);
}
/**
* Computes a hash code from attributes: {@code disk}, {@code memory}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(disk);
h += (h << 5) + Objects.hashCode(memory);
return h;
}
/**
* Prints the immutable value {@code Limits} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "Limits{"
+ "disk=" + disk
+ ", memory=" + memory
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CloudTask.Limits", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements CloudTask.Limits {
Integer disk;
Integer memory;
@JsonProperty("disk")
public void setDisk(@Nullable Integer disk) {
this.disk = disk;
}
@JsonProperty("memory")
public void setMemory(@Nullable Integer memory) {
this.memory = memory;
}
@Override
public Integer getDisk() { throw new UnsupportedOperationException(); }
@Override
public Integer getMemory() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCloudTask.ImmutableLimits fromJson(Json json) {
ImmutableCloudTask.ImmutableLimits.Builder builder = ImmutableCloudTask.ImmutableLimits.builder();
if (json.disk != null) {
builder.disk(json.disk);
}
if (json.memory != null) {
builder.memory(json.memory);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link CloudTask.Limits} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Limits instance
*/
public static ImmutableCloudTask.ImmutableLimits copyOf(CloudTask.Limits instance) {
if (instance instanceof ImmutableCloudTask.ImmutableLimits) {
return (ImmutableCloudTask.ImmutableLimits) instance;
}
return ImmutableCloudTask.ImmutableLimits.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudTask.ImmutableLimits ImmutableLimits}.
*
* ImmutableCloudTask.ImmutableLimits.builder()
* .disk(Integer | null) // nullable {@link CloudTask.Limits#getDisk() disk}
* .memory(Integer | null) // nullable {@link CloudTask.Limits#getMemory() memory}
* .build();
*
* @return A new ImmutableLimits builder
*/
public static ImmutableCloudTask.ImmutableLimits.Builder builder() {
return new ImmutableCloudTask.ImmutableLimits.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudTask.ImmutableLimits ImmutableLimits}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudTask.Limits", generator = "Immutables")
public static final class Builder {
private Integer disk;
private Integer memory;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Limits} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudTask.Limits instance) {
Objects.requireNonNull(instance, "instance");
@Nullable Integer diskValue = instance.getDisk();
if (diskValue != null) {
disk(diskValue);
}
@Nullable Integer memoryValue = instance.getMemory();
if (memoryValue != null) {
memory(memoryValue);
}
return this;
}
/**
* Initializes the value for the {@link CloudTask.Limits#getDisk() disk} attribute.
* @param disk The value for disk (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("disk")
public final Builder disk(@Nullable Integer disk) {
this.disk = disk;
return this;
}
/**
* Initializes the value for the {@link CloudTask.Limits#getMemory() memory} attribute.
* @param memory The value for memory (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("memory")
public final Builder memory(@Nullable Integer memory) {
this.memory = memory;
return this;
}
/**
* Builds a new {@link ImmutableCloudTask.ImmutableLimits ImmutableLimits}.
* @return An immutable instance of Limits
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudTask.ImmutableLimits build() {
return new ImmutableCloudTask.ImmutableLimits(disk, memory);
}
}
}
}