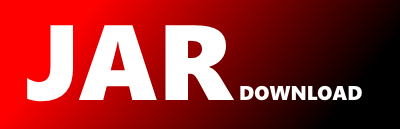
org.cloudfoundry.client.lib.domain.ImmutableUpload Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.domain;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.client.lib.domain.annotation.Nullable;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link Upload}.
*
* Use the builder to create immutable instances:
* {@code ImmutableUpload.builder()}.
*/
@Generated(from = "Upload", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableUpload implements Upload {
private final Status status;
private final @Nullable ErrorDetails errorDetails;
private ImmutableUpload(
Status status,
@Nullable ErrorDetails errorDetails) {
this.status = status;
this.errorDetails = errorDetails;
}
/**
* @return The value of the {@code status} attribute
*/
@JsonProperty("status")
@Override
public Status getStatus() {
return status;
}
/**
* @return The value of the {@code errorDetails} attribute
*/
@JsonProperty("errorDetails")
@Override
public @Nullable ErrorDetails getErrorDetails() {
return errorDetails;
}
/**
* Copy the current immutable object by setting a value for the {@link Upload#getStatus() status} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for status
* @return A modified copy of the {@code this} object
*/
public final ImmutableUpload withStatus(Status value) {
if (this.status == value) return this;
Status newValue = Objects.requireNonNull(value, "status");
if (this.status.equals(newValue)) return this;
return new ImmutableUpload(newValue, this.errorDetails);
}
/**
* Copy the current immutable object by setting a value for the {@link Upload#getErrorDetails() errorDetails} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for errorDetails (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableUpload withErrorDetails(@Nullable ErrorDetails value) {
if (this.errorDetails == value) return this;
return new ImmutableUpload(this.status, value);
}
/**
* This instance is equal to all instances of {@code ImmutableUpload} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableUpload
&& equalTo((ImmutableUpload) another);
}
private boolean equalTo(ImmutableUpload another) {
return status.equals(another.status)
&& Objects.equals(errorDetails, another.errorDetails);
}
/**
* Computes a hash code from attributes: {@code status}, {@code errorDetails}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + status.hashCode();
h += (h << 5) + Objects.hashCode(errorDetails);
return h;
}
/**
* Prints the immutable value {@code Upload} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "Upload{"
+ "status=" + status
+ ", errorDetails=" + errorDetails
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "Upload", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements Upload {
Status status;
ErrorDetails errorDetails;
@JsonProperty("status")
public void setStatus(Status status) {
this.status = status;
}
@JsonProperty("errorDetails")
public void setErrorDetails(@Nullable ErrorDetails errorDetails) {
this.errorDetails = errorDetails;
}
@Override
public Status getStatus() { throw new UnsupportedOperationException(); }
@Override
public ErrorDetails getErrorDetails() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableUpload fromJson(Json json) {
ImmutableUpload.Builder builder = ImmutableUpload.builder();
if (json.status != null) {
builder.status(json.status);
}
if (json.errorDetails != null) {
builder.errorDetails(json.errorDetails);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link Upload} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Upload instance
*/
public static ImmutableUpload copyOf(Upload instance) {
if (instance instanceof ImmutableUpload) {
return (ImmutableUpload) instance;
}
return ImmutableUpload.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableUpload ImmutableUpload}.
*
* ImmutableUpload.builder()
* .status(org.cloudfoundry.client.lib.domain.Status) // required {@link Upload#getStatus() status}
* .errorDetails(org.cloudfoundry.client.lib.domain.ErrorDetails | null) // nullable {@link Upload#getErrorDetails() errorDetails}
* .build();
*
* @return A new ImmutableUpload builder
*/
public static ImmutableUpload.Builder builder() {
return new ImmutableUpload.Builder();
}
/**
* Builds instances of type {@link ImmutableUpload ImmutableUpload}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Upload", generator = "Immutables")
public static final class Builder {
private static final long INIT_BIT_STATUS = 0x1L;
private long initBits = 0x1L;
private Status status;
private ErrorDetails errorDetails;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Upload} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Upload instance) {
Objects.requireNonNull(instance, "instance");
status(instance.getStatus());
@Nullable ErrorDetails errorDetailsValue = instance.getErrorDetails();
if (errorDetailsValue != null) {
errorDetails(errorDetailsValue);
}
return this;
}
/**
* Initializes the value for the {@link Upload#getStatus() status} attribute.
* @param status The value for status
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("status")
public final Builder status(Status status) {
this.status = Objects.requireNonNull(status, "status");
initBits &= ~INIT_BIT_STATUS;
return this;
}
/**
* Initializes the value for the {@link Upload#getErrorDetails() errorDetails} attribute.
* @param errorDetails The value for errorDetails (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("errorDetails")
public final Builder errorDetails(@Nullable ErrorDetails errorDetails) {
this.errorDetails = errorDetails;
return this;
}
/**
* Builds a new {@link ImmutableUpload ImmutableUpload}.
* @return An immutable instance of Upload
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableUpload build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableUpload(status, errorDetails);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_STATUS) != 0) attributes.add("status");
return "Cannot build Upload, some of required attributes are not set " + attributes;
}
}
}