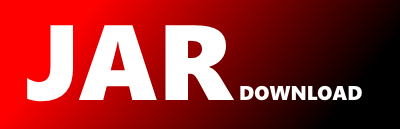
org.cloudfoundry.client.lib.rest.CloudControllerRestClientFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudfoundry-client-lib Show documentation
Show all versions of cloudfoundry-client-lib Show documentation
A Cloud Foundry client library for Java
The newest version!
/*
* Copyright 2009-2012 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cloudfoundry.client.lib.rest;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.text.MessageFormat;
import java.time.Duration;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import org.cloudfoundry.client.CloudFoundryClient;
import org.cloudfoundry.client.lib.CloudCredentials;
import org.cloudfoundry.client.lib.adapters.CloudFoundryClientFactory;
import org.cloudfoundry.client.lib.adapters.ImmutableCloudFoundryClientFactory;
import org.cloudfoundry.client.lib.domain.CloudSpace;
import org.cloudfoundry.client.lib.oauth2.OAuthClient;
import org.cloudfoundry.client.lib.util.RestUtil;
import org.cloudfoundry.doppler.DopplerClient;
import org.cloudfoundry.reactor.ConnectionContext;
import org.immutables.value.Value;
import org.springframework.util.StringUtils;
import org.springframework.web.reactive.function.client.ExchangeFilterFunction;
import org.springframework.web.reactive.function.client.WebClient;
import org.springframework.web.reactive.function.client.WebClient.Builder;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
@Value.Immutable
public abstract class CloudControllerRestClientFactory {
private final Map> infoCache = new HashMap<>();
private final ObjectMapper objectMapper = new ObjectMapper();
private final RestUtil restUtil = new RestUtil();
public abstract Optional getSslHandshakeTimeout();
public abstract Optional getConnectTimeout();
public abstract Optional getConnectionPoolSize();
public abstract Optional getThreadPoolSize();
@Value.Default
public boolean shouldTrustSelfSignedCertificates() {
return false;
}
@Value.Derived
public CloudFoundryClientFactory getCloudFoundryClientFactory() {
ImmutableCloudFoundryClientFactory.Builder builder = ImmutableCloudFoundryClientFactory.builder();
getSslHandshakeTimeout().ifPresent(builder::sslHandshakeTimeout);
getConnectTimeout().ifPresent(builder::connectTimeout);
getConnectionPoolSize().ifPresent(builder::connectionPoolSize);
getThreadPoolSize().ifPresent(builder::threadPoolSize);
return builder.build();
}
public WebClient getGeneralPurposeWebClient() {
return restUtil.createWebClient(shouldTrustSelfSignedCertificates());
}
public CloudControllerRestClient createClient(URL controllerUrl, CloudCredentials credentials, String organizationName,
String spaceName, OAuthClient oAuthClient, List exchangeFilters) {
CloudControllerRestClient clientWithoutTarget = createClient(controllerUrl, credentials, oAuthClient);
CloudSpace target = clientWithoutTarget.getSpace(organizationName, spaceName);
return createClient(controllerUrl, credentials, target, oAuthClient, exchangeFilters);
}
public CloudControllerRestClient createClient(URL controllerUrl, CloudCredentials credentials, OAuthClient oAuthClient) {
return createClient(controllerUrl, credentials, null, oAuthClient, Collections.emptyList());
}
public CloudControllerRestClient createClient(URL controllerUrl, CloudCredentials credentials, CloudSpace target) {
return createClient(controllerUrl, credentials, target, createOAuthClient(controllerUrl, credentials.getOrigin()),
Collections.emptyList());
}
public CloudControllerRestClient createClient(URL controllerUrl, CloudCredentials credentials, CloudSpace target,
OAuthClient oAuthClient, List exchangeFilters) {
WebClient webClient = createWebClient(credentials, oAuthClient, exchangeFilters);
CloudFoundryClient delegate = getCloudFoundryClientFactory().createClient(controllerUrl, oAuthClient);
DopplerClient dopplerClient = getCloudFoundryClientFactory().createDopplerClient(controllerUrl, oAuthClient);
return new CloudControllerRestClientImpl(controllerUrl, credentials, webClient, oAuthClient, delegate, dopplerClient, target);
}
private OAuthClient createOAuthClient(URL controllerUrl, String origin) {
Map infoMap = getInfoMap(controllerUrl);
URL authorizationEndpoint = getAuthorizationEndpoint(infoMap);
if (StringUtils.isEmpty(origin)) {
return restUtil.createOAuthClient(authorizationEndpoint, shouldTrustSelfSignedCertificates());
}
ConnectionContext connectionContext = getCloudFoundryClientFactory().getOrCreateConnectionContext(controllerUrl.getHost());
return restUtil.createOAuthClient(authorizationEndpoint, shouldTrustSelfSignedCertificates(), connectionContext, origin);
}
private Map getInfoMap(URL controllerUrl) {
if (infoCache.containsKey(controllerUrl)) {
return infoCache.get(controllerUrl);
}
String infoResponse = getGeneralPurposeWebClient().get()
.uri(controllerUrl + "/v2/info")
.retrieve()
.bodyToMono(String.class)
.block();
try {
return objectMapper.readValue(infoResponse, new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy