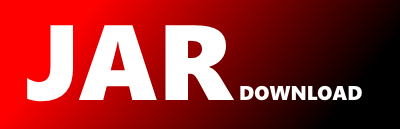
org.cloudfoundry.client.lib.rest.ImmutableCloudControllerRestClientFactory Maven / Gradle / Ivy
Show all versions of cloudfoundry-client-lib Show documentation
package org.cloudfoundry.client.lib.rest;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.cloudfoundry.client.lib.adapters.CloudFoundryClientFactory;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link CloudControllerRestClientFactory}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCloudControllerRestClientFactory.builder()}.
*/
@Generated(from = "CloudControllerRestClientFactory", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableCloudControllerRestClientFactory
extends CloudControllerRestClientFactory {
private final Duration sslHandshakeTimeout;
private final Duration connectTimeout;
private final Integer connectionPoolSize;
private final Integer threadPoolSize;
private final boolean shouldTrustSelfSignedCertificates;
private transient final CloudFoundryClientFactory cloudFoundryClientFactory;
private ImmutableCloudControllerRestClientFactory(ImmutableCloudControllerRestClientFactory.Builder builder) {
this.sslHandshakeTimeout = builder.sslHandshakeTimeout;
this.connectTimeout = builder.connectTimeout;
this.connectionPoolSize = builder.connectionPoolSize;
this.threadPoolSize = builder.threadPoolSize;
if (builder.shouldTrustSelfSignedCertificatesIsSet()) {
initShim.shouldTrustSelfSignedCertificates(builder.shouldTrustSelfSignedCertificates);
}
this.shouldTrustSelfSignedCertificates = initShim.shouldTrustSelfSignedCertificates();
this.cloudFoundryClientFactory = initShim.getCloudFoundryClientFactory();
this.initShim = null;
}
private ImmutableCloudControllerRestClientFactory(
Duration sslHandshakeTimeout,
Duration connectTimeout,
Integer connectionPoolSize,
Integer threadPoolSize,
boolean shouldTrustSelfSignedCertificates) {
this.sslHandshakeTimeout = sslHandshakeTimeout;
this.connectTimeout = connectTimeout;
this.connectionPoolSize = connectionPoolSize;
this.threadPoolSize = threadPoolSize;
initShim.shouldTrustSelfSignedCertificates(shouldTrustSelfSignedCertificates);
this.shouldTrustSelfSignedCertificates = initShim.shouldTrustSelfSignedCertificates();
this.cloudFoundryClientFactory = initShim.getCloudFoundryClientFactory();
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "CloudControllerRestClientFactory", generator = "Immutables")
private final class InitShim {
private byte shouldTrustSelfSignedCertificatesBuildStage = STAGE_UNINITIALIZED;
private boolean shouldTrustSelfSignedCertificates;
boolean shouldTrustSelfSignedCertificates() {
if (shouldTrustSelfSignedCertificatesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (shouldTrustSelfSignedCertificatesBuildStage == STAGE_UNINITIALIZED) {
shouldTrustSelfSignedCertificatesBuildStage = STAGE_INITIALIZING;
this.shouldTrustSelfSignedCertificates = ImmutableCloudControllerRestClientFactory.super.shouldTrustSelfSignedCertificates();
shouldTrustSelfSignedCertificatesBuildStage = STAGE_INITIALIZED;
}
return this.shouldTrustSelfSignedCertificates;
}
void shouldTrustSelfSignedCertificates(boolean shouldTrustSelfSignedCertificates) {
this.shouldTrustSelfSignedCertificates = shouldTrustSelfSignedCertificates;
shouldTrustSelfSignedCertificatesBuildStage = STAGE_INITIALIZED;
}
private byte cloudFoundryClientFactoryBuildStage = STAGE_UNINITIALIZED;
private CloudFoundryClientFactory cloudFoundryClientFactory;
CloudFoundryClientFactory getCloudFoundryClientFactory() {
if (cloudFoundryClientFactoryBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (cloudFoundryClientFactoryBuildStage == STAGE_UNINITIALIZED) {
cloudFoundryClientFactoryBuildStage = STAGE_INITIALIZING;
this.cloudFoundryClientFactory = Objects.requireNonNull(ImmutableCloudControllerRestClientFactory.super.getCloudFoundryClientFactory(), "cloudFoundryClientFactory");
cloudFoundryClientFactoryBuildStage = STAGE_INITIALIZED;
}
return this.cloudFoundryClientFactory;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (shouldTrustSelfSignedCertificatesBuildStage == STAGE_INITIALIZING) attributes.add("shouldTrustSelfSignedCertificates");
if (cloudFoundryClientFactoryBuildStage == STAGE_INITIALIZING) attributes.add("cloudFoundryClientFactory");
return "Cannot build CloudControllerRestClientFactory, attribute initializers form cycle " + attributes;
}
}
/**
* @return The value of the {@code sslHandshakeTimeout} attribute
*/
@Override
public Optional getSslHandshakeTimeout() {
return Optional.ofNullable(sslHandshakeTimeout);
}
/**
* @return The value of the {@code connectTimeout} attribute
*/
@Override
public Optional getConnectTimeout() {
return Optional.ofNullable(connectTimeout);
}
/**
* @return The value of the {@code connectionPoolSize} attribute
*/
@Override
public Optional getConnectionPoolSize() {
return Optional.ofNullable(connectionPoolSize);
}
/**
* @return The value of the {@code threadPoolSize} attribute
*/
@Override
public Optional getThreadPoolSize() {
return Optional.ofNullable(threadPoolSize);
}
/**
* @return The value of the {@code shouldTrustSelfSignedCertificates} attribute
*/
@Override
public boolean shouldTrustSelfSignedCertificates() {
InitShim shim = this.initShim;
return shim != null
? shim.shouldTrustSelfSignedCertificates()
: this.shouldTrustSelfSignedCertificates;
}
/**
* @return The computed-at-construction value of the {@code cloudFoundryClientFactory} attribute
*/
@Override
public CloudFoundryClientFactory getCloudFoundryClientFactory() {
InitShim shim = this.initShim;
return shim != null
? shim.getCloudFoundryClientFactory()
: this.cloudFoundryClientFactory;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudControllerRestClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} attribute.
* @param value The value for sslHandshakeTimeout
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withSslHandshakeTimeout(Duration value) {
Duration newValue = Objects.requireNonNull(value, "sslHandshakeTimeout");
if (this.sslHandshakeTimeout == newValue) return this;
return new ImmutableCloudControllerRestClientFactory(
newValue,
this.connectTimeout,
this.connectionPoolSize,
this.threadPoolSize,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudControllerRestClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for sslHandshakeTimeout
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableCloudControllerRestClientFactory withSslHandshakeTimeout(Optional extends Duration> optional) {
Duration value = optional.orElse(null);
if (this.sslHandshakeTimeout == value) return this;
return new ImmutableCloudControllerRestClientFactory(
value,
this.connectTimeout,
this.connectionPoolSize,
this.threadPoolSize,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudControllerRestClientFactory#getConnectTimeout() connectTimeout} attribute.
* @param value The value for connectTimeout
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withConnectTimeout(Duration value) {
Duration newValue = Objects.requireNonNull(value, "connectTimeout");
if (this.connectTimeout == newValue) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
newValue,
this.connectionPoolSize,
this.threadPoolSize,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudControllerRestClientFactory#getConnectTimeout() connectTimeout} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for connectTimeout
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableCloudControllerRestClientFactory withConnectTimeout(Optional extends Duration> optional) {
Duration value = optional.orElse(null);
if (this.connectTimeout == value) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
value,
this.connectionPoolSize,
this.threadPoolSize,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudControllerRestClientFactory#getConnectionPoolSize() connectionPoolSize} attribute.
* @param value The value for connectionPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withConnectionPoolSize(int value) {
Integer newValue = value;
if (Objects.equals(this.connectionPoolSize, newValue)) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
this.connectTimeout,
newValue,
this.threadPoolSize,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudControllerRestClientFactory#getConnectionPoolSize() connectionPoolSize} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for connectionPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withConnectionPoolSize(Optional optional) {
Integer value = optional.orElse(null);
if (Objects.equals(this.connectionPoolSize, value)) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
this.connectTimeout,
value,
this.threadPoolSize,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link CloudControllerRestClientFactory#getThreadPoolSize() threadPoolSize} attribute.
* @param value The value for threadPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withThreadPoolSize(int value) {
Integer newValue = value;
if (Objects.equals(this.threadPoolSize, newValue)) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
this.connectTimeout,
this.connectionPoolSize,
newValue,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting an optional value for the {@link CloudControllerRestClientFactory#getThreadPoolSize() threadPoolSize} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for threadPoolSize
* @return A modified copy of {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withThreadPoolSize(Optional optional) {
Integer value = optional.orElse(null);
if (Objects.equals(this.threadPoolSize, value)) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
this.connectTimeout,
this.connectionPoolSize,
value,
this.shouldTrustSelfSignedCertificates);
}
/**
* Copy the current immutable object by setting a value for the {@link CloudControllerRestClientFactory#shouldTrustSelfSignedCertificates() shouldTrustSelfSignedCertificates} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for shouldTrustSelfSignedCertificates
* @return A modified copy of the {@code this} object
*/
public final ImmutableCloudControllerRestClientFactory withShouldTrustSelfSignedCertificates(boolean value) {
if (this.shouldTrustSelfSignedCertificates == value) return this;
return new ImmutableCloudControllerRestClientFactory(
this.sslHandshakeTimeout,
this.connectTimeout,
this.connectionPoolSize,
this.threadPoolSize,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableCloudControllerRestClientFactory} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCloudControllerRestClientFactory
&& equalTo((ImmutableCloudControllerRestClientFactory) another);
}
private boolean equalTo(ImmutableCloudControllerRestClientFactory another) {
return Objects.equals(sslHandshakeTimeout, another.sslHandshakeTimeout)
&& Objects.equals(connectTimeout, another.connectTimeout)
&& Objects.equals(connectionPoolSize, another.connectionPoolSize)
&& Objects.equals(threadPoolSize, another.threadPoolSize)
&& shouldTrustSelfSignedCertificates == another.shouldTrustSelfSignedCertificates
&& cloudFoundryClientFactory.equals(another.cloudFoundryClientFactory);
}
/**
* Computes a hash code from attributes: {@code sslHandshakeTimeout}, {@code connectTimeout}, {@code connectionPoolSize}, {@code threadPoolSize}, {@code shouldTrustSelfSignedCertificates}, {@code cloudFoundryClientFactory}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(sslHandshakeTimeout);
h += (h << 5) + Objects.hashCode(connectTimeout);
h += (h << 5) + Objects.hashCode(connectionPoolSize);
h += (h << 5) + Objects.hashCode(threadPoolSize);
h += (h << 5) + Boolean.hashCode(shouldTrustSelfSignedCertificates);
h += (h << 5) + cloudFoundryClientFactory.hashCode();
return h;
}
/**
* Prints the immutable value {@code CloudControllerRestClientFactory} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("CloudControllerRestClientFactory{");
if (sslHandshakeTimeout != null) {
builder.append("sslHandshakeTimeout=").append(sslHandshakeTimeout);
}
if (connectTimeout != null) {
if (builder.length() > 33) builder.append(", ");
builder.append("connectTimeout=").append(connectTimeout);
}
if (connectionPoolSize != null) {
if (builder.length() > 33) builder.append(", ");
builder.append("connectionPoolSize=").append(connectionPoolSize);
}
if (threadPoolSize != null) {
if (builder.length() > 33) builder.append(", ");
builder.append("threadPoolSize=").append(threadPoolSize);
}
if (builder.length() > 33) builder.append(", ");
builder.append("shouldTrustSelfSignedCertificates=").append(shouldTrustSelfSignedCertificates);
builder.append(", ");
builder.append("cloudFoundryClientFactory=").append(cloudFoundryClientFactory);
return builder.append("}").toString();
}
/**
* Creates an immutable copy of a {@link CloudControllerRestClientFactory} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CloudControllerRestClientFactory instance
*/
public static ImmutableCloudControllerRestClientFactory copyOf(CloudControllerRestClientFactory instance) {
if (instance instanceof ImmutableCloudControllerRestClientFactory) {
return (ImmutableCloudControllerRestClientFactory) instance;
}
return ImmutableCloudControllerRestClientFactory.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCloudControllerRestClientFactory ImmutableCloudControllerRestClientFactory}.
*
* ImmutableCloudControllerRestClientFactory.builder()
* .sslHandshakeTimeout(java.time.Duration) // optional {@link CloudControllerRestClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout}
* .connectTimeout(java.time.Duration) // optional {@link CloudControllerRestClientFactory#getConnectTimeout() connectTimeout}
* .connectionPoolSize(Integer) // optional {@link CloudControllerRestClientFactory#getConnectionPoolSize() connectionPoolSize}
* .threadPoolSize(Integer) // optional {@link CloudControllerRestClientFactory#getThreadPoolSize() threadPoolSize}
* .shouldTrustSelfSignedCertificates(boolean) // optional {@link CloudControllerRestClientFactory#shouldTrustSelfSignedCertificates() shouldTrustSelfSignedCertificates}
* .build();
*
* @return A new ImmutableCloudControllerRestClientFactory builder
*/
public static ImmutableCloudControllerRestClientFactory.Builder builder() {
return new ImmutableCloudControllerRestClientFactory.Builder();
}
/**
* Builds instances of type {@link ImmutableCloudControllerRestClientFactory ImmutableCloudControllerRestClientFactory}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CloudControllerRestClientFactory", generator = "Immutables")
public static final class Builder {
private static final long OPT_BIT_SHOULD_TRUST_SELF_SIGNED_CERTIFICATES = 0x1L;
private long optBits;
private Duration sslHandshakeTimeout;
private Duration connectTimeout;
private Integer connectionPoolSize;
private Integer threadPoolSize;
private boolean shouldTrustSelfSignedCertificates;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CloudControllerRestClientFactory} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CloudControllerRestClientFactory instance) {
Objects.requireNonNull(instance, "instance");
Optional sslHandshakeTimeoutOptional = instance.getSslHandshakeTimeout();
if (sslHandshakeTimeoutOptional.isPresent()) {
sslHandshakeTimeout(sslHandshakeTimeoutOptional);
}
Optional connectTimeoutOptional = instance.getConnectTimeout();
if (connectTimeoutOptional.isPresent()) {
connectTimeout(connectTimeoutOptional);
}
Optional connectionPoolSizeOptional = instance.getConnectionPoolSize();
if (connectionPoolSizeOptional.isPresent()) {
connectionPoolSize(connectionPoolSizeOptional);
}
Optional threadPoolSizeOptional = instance.getThreadPoolSize();
if (threadPoolSizeOptional.isPresent()) {
threadPoolSize(threadPoolSizeOptional);
}
shouldTrustSelfSignedCertificates(instance.shouldTrustSelfSignedCertificates());
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} to sslHandshakeTimeout.
* @param sslHandshakeTimeout The value for sslHandshakeTimeout
* @return {@code this} builder for chained invocation
*/
public final Builder sslHandshakeTimeout(Duration sslHandshakeTimeout) {
this.sslHandshakeTimeout = Objects.requireNonNull(sslHandshakeTimeout, "sslHandshakeTimeout");
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getSslHandshakeTimeout() sslHandshakeTimeout} to sslHandshakeTimeout.
* @param sslHandshakeTimeout The value for sslHandshakeTimeout
* @return {@code this} builder for use in a chained invocation
*/
public final Builder sslHandshakeTimeout(Optional extends Duration> sslHandshakeTimeout) {
this.sslHandshakeTimeout = sslHandshakeTimeout.orElse(null);
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getConnectTimeout() connectTimeout} to connectTimeout.
* @param connectTimeout The value for connectTimeout
* @return {@code this} builder for chained invocation
*/
public final Builder connectTimeout(Duration connectTimeout) {
this.connectTimeout = Objects.requireNonNull(connectTimeout, "connectTimeout");
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getConnectTimeout() connectTimeout} to connectTimeout.
* @param connectTimeout The value for connectTimeout
* @return {@code this} builder for use in a chained invocation
*/
public final Builder connectTimeout(Optional extends Duration> connectTimeout) {
this.connectTimeout = connectTimeout.orElse(null);
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getConnectionPoolSize() connectionPoolSize} to connectionPoolSize.
* @param connectionPoolSize The value for connectionPoolSize
* @return {@code this} builder for chained invocation
*/
public final Builder connectionPoolSize(int connectionPoolSize) {
this.connectionPoolSize = connectionPoolSize;
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getConnectionPoolSize() connectionPoolSize} to connectionPoolSize.
* @param connectionPoolSize The value for connectionPoolSize
* @return {@code this} builder for use in a chained invocation
*/
public final Builder connectionPoolSize(Optional connectionPoolSize) {
this.connectionPoolSize = connectionPoolSize.orElse(null);
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getThreadPoolSize() threadPoolSize} to threadPoolSize.
* @param threadPoolSize The value for threadPoolSize
* @return {@code this} builder for chained invocation
*/
public final Builder threadPoolSize(int threadPoolSize) {
this.threadPoolSize = threadPoolSize;
return this;
}
/**
* Initializes the optional value {@link CloudControllerRestClientFactory#getThreadPoolSize() threadPoolSize} to threadPoolSize.
* @param threadPoolSize The value for threadPoolSize
* @return {@code this} builder for use in a chained invocation
*/
public final Builder threadPoolSize(Optional threadPoolSize) {
this.threadPoolSize = threadPoolSize.orElse(null);
return this;
}
/**
* Initializes the value for the {@link CloudControllerRestClientFactory#shouldTrustSelfSignedCertificates() shouldTrustSelfSignedCertificates} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link CloudControllerRestClientFactory#shouldTrustSelfSignedCertificates() shouldTrustSelfSignedCertificates}.
* @param shouldTrustSelfSignedCertificates The value for shouldTrustSelfSignedCertificates
* @return {@code this} builder for use in a chained invocation
*/
public final Builder shouldTrustSelfSignedCertificates(boolean shouldTrustSelfSignedCertificates) {
this.shouldTrustSelfSignedCertificates = shouldTrustSelfSignedCertificates;
optBits |= OPT_BIT_SHOULD_TRUST_SELF_SIGNED_CERTIFICATES;
return this;
}
/**
* Builds a new {@link ImmutableCloudControllerRestClientFactory ImmutableCloudControllerRestClientFactory}.
* @return An immutable instance of CloudControllerRestClientFactory
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCloudControllerRestClientFactory build() {
return new ImmutableCloudControllerRestClientFactory(this);
}
private boolean shouldTrustSelfSignedCertificatesIsSet() {
return (optBits & OPT_BIT_SHOULD_TRUST_SELF_SIGNED_CERTIFICATES) != 0;
}
}
}