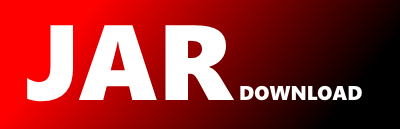
com.sap.cloud.mt.runtime.DataSourceCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-runtime Show documentation
Show all versions of multi-tenant-runtime Show documentation
Spring Boot Enablement Parent
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.mt.runtime;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.stream.Stream;
import javax.sql.DataSource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.sap.cloud.mt.runtime.DataPoolSettings.Parameter;
import com.sap.cloud.mt.subscription.DataSourceInfo;
import com.sap.cloud.mt.subscription.exceptions.InternalError;
public abstract class DataSourceCreator {
private static final Logger logger= LoggerFactory.getLogger(DataSourceCreator.class);
public final DataSource create(DataSourceInfo info, EnvironmentAccess env) throws InternalError{
DataSource dataSource = build(info);
setPoolParameters(dataSource, env);
return dataSource;
}
private final void setPoolParameters(DataSource dataSource, EnvironmentAccess env) {
getPoolParameters().forEach(p -> {
Object para = env.getProperty(p.getNameInEnv(), p.getType());
if (para != null) {
try {
getMethod(dataSource, p).invoke(dataSource, para);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException(e);
}
}
});
}
protected abstract DataSource build(DataSourceInfo info) throws InternalError;
protected abstract Stream getPoolParameters();
private Method getMethod(DataSource dataSource, DataPoolSettings.Parameter p) throws NoSuchMethodException {
try {
return dataSource.getClass().getMethod(p.getSetterName(), p.getType());
} catch (NoSuchMethodException e) {
logger.debug("Try with another type");
if (p.getType() == boolean.class) {
return dataSource.getClass().getMethod(p.getSetterName(), Boolean.class);
} else if (p.getType() == int.class) {
return dataSource.getClass().getMethod(p.getSetterName(), Integer.class);
} else if (p.getType() == long.class) {
return dataSource.getClass().getMethod(p.getSetterName(), Long.class);
} else throw new NoSuchMethodException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy