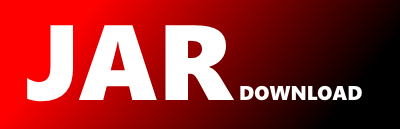
com.sap.cloud.mt.runtime.DataSourceCreatorAtomikos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-runtime Show documentation
Show all versions of multi-tenant-runtime Show documentation
Spring Boot Enablement Parent
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.mt.runtime;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.stream.Stream;
import javax.sql.DataSource;
import com.sap.cloud.mt.runtime.DataPoolSettings.Parameter;
import com.sap.cloud.mt.subscription.DataSourceInfo;
import com.sap.cloud.mt.subscription.exceptions.InternalError;
public class DataSourceCreatorAtomikos extends DataSourceCreator {
private final DataPoolSettings poolSettings;
public DataSourceCreatorAtomikos(DataPoolSettings poolSettings) {
this.poolSettings = poolSettings;
}
@Override
protected DataSource build(DataSourceInfo info) throws InternalError {
try {
Object xaDataSource = null;
if (info.getDriver().equals("com.sap.db.jdbc.Driver")) {
Class> xaClazz = Class.forName("com.sap.db.jdbcext.XADataSourceSAP");
xaDataSource = xaClazz.newInstance();
Method setUrlMethod = xaClazz.getMethod("setUrl", String.class);
Method setUserMethod = xaClazz.getMethod("setUser", String.class);
Method setPasswordMethod = xaClazz.getMethod("setPassword", String.class);
Method setSchemaMethod = xaClazz.getMethod("setSchema", String.class);
Method setConnectionProperty = xaClazz.getMethod("setConnectionProperty", String.class, String.class);
setUrlMethod.invoke(xaDataSource, info.getUrl());
setUserMethod.invoke(xaDataSource, info.getUser());
setPasswordMethod.invoke(xaDataSource, info.getPassword());
setSchemaMethod.invoke(xaDataSource, info.getSchema());
if (info.getUrl().contains("?encrypt=true")) setConnectionProperty.invoke(xaDataSource, "encrypt", "true");
} else if (info.getDriver().equals("org.h2.Driver")) {
Class> xaClazz = Class.forName("org.h2.jdbcx.JdbcDataSource");
xaDataSource = xaClazz.newInstance();
Method setUrlMethod = xaClazz.getMethod("setUrl", String.class);
Method setUserMethod = xaClazz.getMethod("setUser", String.class);
Method setPasswordMethod = xaClazz.getMethod("setPassword", String.class);
setUrlMethod.invoke(xaDataSource, info.getUrl());
setUserMethod.invoke(xaDataSource, info.getUser());
setPasswordMethod.invoke(xaDataSource, info.getPassword());
} else {
throw new InternalError("Driver " + info.getDriver() + " is not supported");
}
Class> atomikosClazz = Class.forName("com.atomikos.jdbc.AtomikosDataSourceBean");
Object atomikosDataSourceBean = atomikosClazz.newInstance();
Method setXaDataSourceMethod = atomikosClazz.getMethod("setXaDataSource", Class.forName("javax.sql.XADataSource"));
Method setUniqueResourceNameMethod = atomikosClazz.getMethod("setUniqueResourceName", String.class);
setXaDataSourceMethod.invoke(atomikosDataSourceBean, xaDataSource);
//ToDo
setUniqueResourceNameMethod.invoke(atomikosDataSourceBean, info.getTenantId() + info.getSchema());
return (DataSource) atomikosDataSourceBean;
} catch (ClassNotFoundException | NoSuchMethodException | InstantiationException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException(e);
}
}
@Override
protected Stream getPoolParameters() {
return poolSettings.getAtomikosParameters();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy