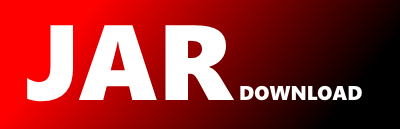
com.sap.cloud.mt.runtime.TenantOverwrite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-runtime Show documentation
Show all versions of multi-tenant-runtime Show documentation
Spring Boot Enablement Parent
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.mt.runtime;
public class TenantOverwrite implements AutoCloseable {
private static final ThreadLocal overwritePerThread = new ThreadLocal<>();
public TenantOverwrite(String tenantId) {
TenantAndCount tenantAndCount = overwritePerThread.get();
if (tenantAndCount != null) {
if (!tenantAndCount.getTenantId().equals(tenantId)) {
throw new UnsupportedOperationException("It is not supported to stack overwrites with different tenants");//NOSONAR
}
} else {
tenantAndCount = new TenantAndCount(tenantId);
overwritePerThread.set(tenantAndCount);
}
tenantAndCount.inc();
}
static public boolean isOverwritten() {
return (overwritePerThread.get() != null);
}
static public String getTenantId() {
return overwritePerThread.get() != null ? overwritePerThread.get().getTenantId() : null;
}
@Override
public void close() {
if (overwritePerThread.get() != null) {
overwritePerThread.get().dec();
if (overwritePerThread.get().counterIsZero()) {
overwritePerThread.remove();
}
}
}
private static class TenantAndCount {
private String tenantId;
private int callDepth = 0;
public TenantAndCount(String tenantId) {
this.tenantId = tenantId;
}
public void inc() {
callDepth++;
}
public void dec() {
callDepth--;
}
public boolean counterIsZero() {
return callDepth == 0;
}
public String getTenantId() {
return tenantId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy