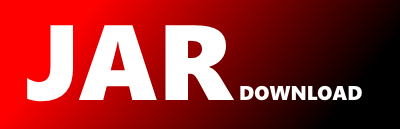
com.sap.cloud.mt.runtime.DataSourceCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-runtime Show documentation
Show all versions of multi-tenant-runtime Show documentation
Spring Boot Enablement Parent
The newest version!
/*******************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved.
******************************************************************************/
package com.sap.cloud.mt.runtime;
import com.sap.cloud.mt.runtime.DataPoolSettings.Parameter;
import com.sap.cloud.mt.subscription.DataSourceInfo;
import com.sap.cloud.mt.subscription.exceptions.InternalError;
import com.sap.cloud.mt.subscription.exceptions.MtLibError;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.sql.DataSource;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Map;
import java.util.Properties;
import java.util.stream.Stream;
public abstract class DataSourceCreator {
private static final Logger logger = LoggerFactory.getLogger(DataSourceCreator.class);
public final DataSource create(DataSourceInfo info, EnvironmentAccess env) throws InternalError {
DataSource dataSource = build(info);
setPoolParameters(dataSource, env);
return dataSource;
}
private void setPoolParameters(DataSource dataSource, EnvironmentAccess env) {
getPoolParameters().forEach(p -> {
Object para;
String normalizedNameInEnv = p.getNormalizedNameInEnv();
if (p.getType() != Properties.class) {
para = env.getProperty(normalizedNameInEnv, p.getType());
} else {
para = env.getPropertiesForPrefix(normalizedNameInEnv);
}
if (para != null) {
try {
if (p.getType() != Properties.class) {
getMethod(dataSource, p).invoke(dataSource, para);
} else {
Properties properties = new Properties();
properties.putAll((Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy