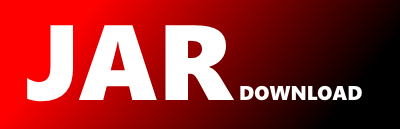
com.sap.cloud.mt.runtime.TenantAwareDataSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-runtime Show documentation
Show all versions of multi-tenant-runtime Show documentation
Spring Boot Enablement Parent
The newest version!
/*******************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved.
******************************************************************************/
package com.sap.cloud.mt.runtime;
import com.sap.cloud.mt.subscription.DataSourceInfo;
import com.sap.cloud.mt.subscription.DbIdentifiers;
import com.sap.cloud.mt.subscription.exceptions.InternalError;
import com.sap.cloud.mt.subscription.exceptions.UnknownTenant;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.sql.DataSource;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.function.BiFunction;
import java.util.function.Supplier;
public class TenantAwareDataSource implements DataSource {
private static final Logger logger = LoggerFactory.getLogger(TenantAwareDataSource.class);
private final Supplier tenantProvider;
private final DataSourceLookup dataSourceLookup;
// db driver,is one data source per db
private final BiFunction connectionProviderFunction = new ConnectionProviderFunction();
public TenantAwareDataSource(Supplier tenantProvider, DataSourceLookup dataSourceLookup) {
this.tenantProvider = tenantProvider;
this.dataSourceLookup = dataSourceLookup;
if (this.tenantProvider == null) {
throw new IllegalArgumentException("No tenant provider set");
}
if (this.dataSourceLookup == null) {
throw new IllegalArgumentException("No data source lookup object set");
}
}
public TenantAwareDataSource(TenantProvider tenantProvider, DataSourceLookup dataSourceLookup) {
this((Supplier) tenantProvider, dataSourceLookup);
}
private DataSourceAndInfo getDataSourceAndInfo() throws InternalError, UnknownTenant {
return dataSourceLookup.getDataSourceAndInfo(tenantProvider.get());
}
@Override
public Connection getConnection() throws SQLException {
logger.debug("A new connection is requested");
ConnectionProvider connectionProvider = null;
DataSourceAndInfo dsAndInfo = null;
try {
dsAndInfo = getDataSourceAndInfo();
} catch (InternalError | UnknownTenant error) {
throw new SQLException(error);
}
DataSourceInfo info = dsAndInfo.getDataSourceInfo();
connectionProvider = connectionProviderFunction.apply(info.getDriver(), dataSourceLookup.isOneDataSourcePerDb());
if (connectionProvider == null) {
throw new SQLException("No connection provider determined for driver " + info.getDriver() +
"and flag oneDataSourcePerDb=" + dataSourceLookup.isOneDataSourcePerDb());
}
try {
return connectionProvider.getConnection(tenantProvider.get(), dsAndInfo);
} catch (SQLException e) {
if (!dataSourceLookup.isAuthenticationProblem(e)) {
throw e;
}
logger.debug("Could not retrieve a connection due to authentication issue => fix data source.", e);
try {
dataSourceLookup.fixDataSourceAfterCredentialChange(tenantProvider.get(), dsAndInfo.getDataSource());
return connectionProvider.getConnection(tenantProvider.get(), getDataSourceAndInfo());
} catch (InternalError | UnknownTenant | SQLException error) {
logger.debug("Could not fix it", error);
throw e;
}
}
}
@Override
public Connection getConnection(String username, String password) throws SQLException {
throw new UnsupportedOperationException("getConnection with username and password isn't supported");
}
@Override
@SuppressWarnings("unchecked")
public T unwrap(Class iface) throws SQLException {
if (iface.isInstance(this)) {
return (T) this;
}
try {
DataSource dataSource = getDataSourceAndInfo().getDataSource();
if (iface.isInstance(dataSource)) {
return (T) dataSource;
}
return dataSource.unwrap(iface);
} catch (InternalError | UnknownTenant | SQLException e) {
throw new SQLException(e);
}
}
@Override
public boolean isWrapperFor(Class> iface) throws SQLException {
try {
if (iface.isInstance(this)) {
return true;
}
DataSource dataSource = getDataSourceAndInfo().getDataSource();
return (iface.isInstance(dataSource) || dataSource.isWrapperFor(iface));
} catch (InternalError | UnknownTenant | SQLException e) {
throw new SQLException(e);
}
}
@Override
public PrintWriter getLogWriter() throws SQLException {
logger.error("Operation getLogWriter is not supported");
throw new UnsupportedOperationException("Operation getLogWriter is not supported");
}
@Override
public void setLogWriter(PrintWriter out) throws SQLException {
logger.error("Operation setLogWriter is not supported");
throw new UnsupportedOperationException("Operation setLogWriter is not supported");
}
@Override
public void setLoginTimeout(int seconds) throws SQLException {
logger.error("Operation setLoginTimeout is not supported");
throw new UnsupportedOperationException("Operation setLoginTimeout is not supported");
}
@Override
public int getLoginTimeout() throws SQLException {
return 0;
}
@Override
public java.util.logging.Logger getParentLogger() throws SQLFeatureNotSupportedException {
return java.util.logging.Logger.getLogger(java.util.logging.Logger.GLOBAL_LOGGER_NAME);
}
public void deleteFromCache(String tenantId) {
dataSourceLookup.deleteFromCache(tenantId);
}
DataSourceLookup getDataSourceLookup() {
return dataSourceLookup;
}
public Supplier getTenantProvider() {
return tenantProvider;
}
boolean doesTenantExist(String tenantId) throws InternalError {
return dataSourceLookup.doesTenantExist(tenantId);
}
public DbIdentifiers.DB getDbType() {
return dataSourceLookup.getDbType();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy