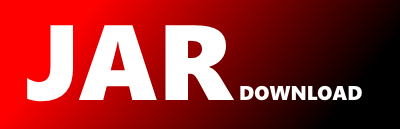
com.sap.cloud.mt.runtime.TenantOverwrite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-runtime Show documentation
Show all versions of multi-tenant-runtime Show documentation
Spring Boot Enablement Parent
The newest version!
/*******************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved.
******************************************************************************/
package com.sap.cloud.mt.runtime;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import static java.lang.StackWalker.Option.RETAIN_CLASS_REFERENCE;
public class TenantOverwrite implements AutoCloseable {
private static final Logger logger = LoggerFactory.getLogger(TenantOverwrite.class);
private static final ThreadLocal overwritePerThread = new ThreadLocal<>();
public TenantOverwrite(String tenantId) {
if (logger.isDebugEnabled()) {
logger.debug("Tenant overwrite called with tenant id {}", tenantId);
var walker = StackWalker.getInstance(RETAIN_CLASS_REFERENCE);
var frame = walker.walk(stream -> stream.skip(1).findFirst().orElse(null));
if (frame != null) {
logger.debug("class={} method={}", frame.getClassName(), frame.getMethodName());
}
}
TenantAndCount tenantAndCount = overwritePerThread.get();
if (tenantAndCount != null) {
logger.debug("There is already a tenant overwrite with count {}", tenantAndCount.callDepth);
if (!tenantAndCount.getTenantId().equals(tenantId)) {
throw new UnsupportedOperationException("It is not supported to stack overwrites with different tenants");//NOSONAR
}
} else {
tenantAndCount = new TenantAndCount(tenantId);
overwritePerThread.set(tenantAndCount);
}
tenantAndCount.inc();
}
public static boolean isOverwritten() {
return (overwritePerThread.get() != null);
}
public static String getTenantId() {
return overwritePerThread.get() != null ? overwritePerThread.get().getTenantId() : null;
}
@Override
public void close() {
logger.debug("Tenant overwrite close called");
if (overwritePerThread.get() != null) {
overwritePerThread.get().dec();
if (overwritePerThread.get().counterIsZero()) {
logger.debug("Tenant overwrite is deleted");
overwritePerThread.remove();
}
}
}
private static class TenantAndCount {
private String tenantId;
private int callDepth = 0;
public TenantAndCount(String tenantId) {
this.tenantId = tenantId;
}
public void inc() {
callDepth++;
}
public void dec() {
callDepth--;
}
public boolean counterIsZero() {
return callDepth == 0;
}
public String getTenantId() {
return tenantId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy