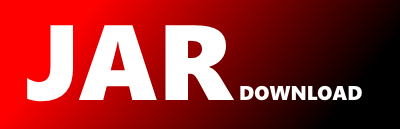
com.sap.cloud.mt.subscription.SidecarAccess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-subscription Show documentation
Show all versions of multi-tenant-subscription Show documentation
Spring Boot Enablement Parent
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.mt.subscription;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.sap.cloud.mt.subscription.exceptions.InternalError;
import com.sap.cloud.mt.subscription.exceptions.NotFound;
import com.sap.cloud.mt.subscription.json.DeletePayload;
import com.sap.cloud.mt.subscription.json.SidecarUnSubscriptionPayload;
import com.sap.cloud.mt.subscription.json.SidecarUpgradePayload;
import com.sap.cloud.mt.subscription.json.SubscriptionPayload;
import com.sap.cloud.mt.tools.api.RequestEnhancer;
import com.sap.cloud.mt.tools.api.ResilienceConfig;
import com.sap.cloud.mt.tools.api.ServiceCall;
import com.sap.cloud.mt.tools.api.ServiceEndpoint;
import com.sap.cloud.mt.tools.api.ServiceResponse;
import com.sap.cloud.mt.tools.exception.InternalException;
import com.sap.cloud.mt.tools.exception.ServiceException;
import com.sap.xsa.core.instancemanager.client.InstanceCreationOptions;
import org.apache.http.HttpHeaders;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import static com.sap.cloud.mt.subscription.MtxTools.extractJobId;
import static org.apache.http.HttpStatus.SC_ACCEPTED;
import static org.apache.http.HttpStatus.SC_BAD_GATEWAY;
import static org.apache.http.HttpStatus.SC_CREATED;
import static org.apache.http.HttpStatus.SC_GATEWAY_TIMEOUT;
import static org.apache.http.HttpStatus.SC_INTERNAL_SERVER_ERROR;
import static org.apache.http.HttpStatus.SC_NOT_FOUND;
import static org.apache.http.HttpStatus.SC_NO_CONTENT;
import static org.apache.http.HttpStatus.SC_OK;
import static org.apache.http.HttpStatus.SC_SERVICE_UNAVAILABLE;
public class SidecarAccess {
private static final String SIDECAR_SUBSCRIBE_ENDPOINT = "/mtx/v1/internal/provisioning/subscribe";
private static final String SIDECAR_UNSUBSCRIBE_ENDPOINT = "/mtx/v1/internal/provisioning/unsubscribe";
private static final String SIDECAR_UPGRADE_ENDPOINT = "/mtx/v1/model/asyncUpgrade";
private static final String SIDECAR_STATUS_ENDPOINT = "/mtx/v1/model/status/";
private static final String MTX_SIDECAR_DESTINATION = "com.sap.cds.mtxSidecar";
private static final String UNEXPECTED_RETURN_CODE = "Unexpected return code ";
private final ServiceEndpoint upgradeEndpoint;
private final ServiceEndpoint getStatusEndpoint;
private final ServiceEndpoint subscribeEndpoint;
private final ServiceEndpoint unsubscribeEndpoint;
private final RequestEnhancer requestEnhancer;
SidecarAccess(String sidecarUrl, ResilienceConfig resilienceConfig, RequestEnhancer requestEnhancer) throws InternalError {
this.requestEnhancer = requestEnhancer;
Set retryCodes = new HashSet<>();
retryCodes.add(SC_BAD_GATEWAY);
retryCodes.add(SC_GATEWAY_TIMEOUT);
retryCodes.add(SC_INTERNAL_SERVER_ERROR);
retryCodes.add(SC_SERVICE_UNAVAILABLE);
retryCodes.add(SC_NOT_FOUND);
try {
upgradeEndpoint = ServiceEndpoint.create()
.at(sidecarUrl)
.destinationName(MTX_SIDECAR_DESTINATION)
.path(SIDECAR_UPGRADE_ENDPOINT)
.returnCodeChecker(c -> {
if (c != SC_OK) {
return new InternalError(UNEXPECTED_RETURN_CODE + c);
} else {
return null;
}
}).retry().forReturnCodes(retryCodes)
.config(resilienceConfig)
.end();
getStatusEndpoint = ServiceEndpoint.create()
.at(sidecarUrl)
.destinationName(MTX_SIDECAR_DESTINATION)
.path(SIDECAR_STATUS_ENDPOINT)
.returnCodeChecker(c -> {
if (c == SC_NOT_FOUND) {
return new NotFound("Job id not known");
}
if (c != SC_OK) {
return new InternalError(UNEXPECTED_RETURN_CODE + c);
}
return null;
}).retry().forReturnCodes(retryCodes)
.config(resilienceConfig)
.end();
subscribeEndpoint = ServiceEndpoint.create()
.at(sidecarUrl)
.destinationName(MTX_SIDECAR_DESTINATION)
.path(SIDECAR_SUBSCRIBE_ENDPOINT)
.returnCodeChecker(c -> {
if (c != SC_CREATED && c != SC_OK && c != SC_ACCEPTED) {
return new InternalError(UNEXPECTED_RETURN_CODE + c);
}
return null;
}).retry().forReturnCodes(retryCodes)
.config(resilienceConfig)
.end();
unsubscribeEndpoint = ServiceEndpoint.create()
.at(sidecarUrl)
.destinationName(MTX_SIDECAR_DESTINATION)
.path(SIDECAR_UNSUBSCRIBE_ENDPOINT)
.returnCodeChecker(c -> {
if (c != SC_ACCEPTED && c != SC_NO_CONTENT) {
return new InternalError(UNEXPECTED_RETURN_CODE + c);
}
return null;
}).retry().forReturnCodes(retryCodes)
.config(resilienceConfig)
.end();
} catch (InternalException e) {
throw new InternalError(e);
}
}
public String callSidecarUpgrade(SidecarUpgradePayload upgradePayload) throws InternalError {
try {
ServiceCall upgrade = upgradeEndpoint.createServiceCall()
.http()
.post()
.payload(upgradePayload)
.noPathParameter()
.noQuery()
.enhancer(requestEnhancer)
.end();
ServiceResponse response = upgrade.execute(String.class);
return response.getPayload().orElse("");
} catch (InternalException e) {
throw new InternalError(e);
} catch (ServiceException e) {
if (e.getCause() instanceof InternalError) {
throw (InternalError) e.getCause();
}
throw new InternalError(e);
}
}
public Map callSidecarStatus(String jobId) throws InternalError, NotFound {
try {
ServiceCall getStatus = getStatusEndpoint.createServiceCall()
.http()
.get()
.withoutPayload()
.pathParameter(jobId)
.noQuery()
.enhancer(requestEnhancer)
.end();
ServiceResponse
© 2015 - 2025 Weber Informatics LLC | Privacy Policy