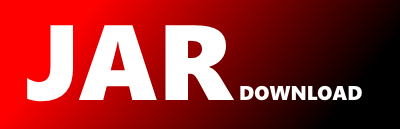
com.sap.cloud.mt.subscription.ClientCredentialJwtAccess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-subscription Show documentation
Show all versions of multi-tenant-subscription Show documentation
Spring Boot Enablement Parent
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cloud.mt.subscription;
import com.sap.cloud.mt.subscription.exceptions.CommunicationError;
import com.sap.cloud.security.xsuaa.client.OAuth2TokenResponse;
import java.time.Instant;
/**
* Class providing access to JWT tokens, a retrieved JWT is cached until it expires
*/
public class ClientCredentialJwtAccess {
private final ClientCredentialJwtReader jwtReader;
// response is buffered as long as JWT is valid
//volatile on object level is ok, as parameters of response never change
private volatile OAuth2TokenResponse oAuth2TokenResponse; //NOSONAR
/**
* @param jwtReader object that retrieves a new JWT from XSUAA
*/
public ClientCredentialJwtAccess(ClientCredentialJwtReader jwtReader) {
this.jwtReader = jwtReader;
}
/**
* Returns a valid JWT
*
* @return the JWT as string
* @throws CommunicationError
*/
public String getJwt() throws CommunicationError {
// see double checked locking for this pattern
OAuth2TokenResponse localRef = oAuth2TokenResponse;
if (isTokenExpired(localRef)) {
synchronized (this) {
localRef = oAuth2TokenResponse;
if (isTokenExpired(localRef)) {
oAuth2TokenResponse = localRef = jwtReader.getJwt();
}
}
}
return localRef.getAccessToken();
}
private static boolean isTokenExpired(OAuth2TokenResponse tokenResponse) {
return tokenResponse == null || Instant.now().isAfter(tokenResponse.getExpiredAt());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy