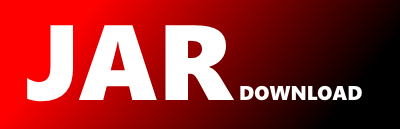
com.sap.cloud.mt.subscription.SaasRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-subscription Show documentation
Show all versions of multi-tenant-subscription Show documentation
Spring Boot Enablement Parent
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.mt.subscription;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.sap.cloud.mt.subscription.exceptions.CommunicationError;
import com.sap.cloud.mt.subscription.exceptions.InternalError;
import com.sap.cloud.mt.subscription.json.SaasCallBackPayload;
import org.apache.http.HttpEntity;
import org.apache.http.HttpHeaders;
import org.apache.http.HttpStatus;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.client.methods.RequestBuilder;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import java.io.IOException;
import java.net.URISyntaxException;
import java.net.URL;
public class SaasRegistry {
private static final String APPLICATION_JSON = "application/json";
static final String SUCCEEDED = "SUCCEEDED";
static final String FAILED = "FAILED";
private final ClientCredentialJwtAccess jwtAccess;
private final String saasRegistryUrl;
private static final String AUTHENTIFICATION_SCHEME = "Bearer";
public SaasRegistry(ClientCredentialJwtReader jwtReader, String saasRegistryUrl) {
jwtAccess = new ClientCredentialJwtAccess(jwtReader);
this.saasRegistryUrl = saasRegistryUrl;
}
public void callBackSaasRegistry(boolean success, String message, String subscriptionUrl, String callbackUrl) throws InternalError {
String completeUrl = saasRegistryUrl + callbackUrl;
try (CloseableHttpClient httpClient = HttpClientBuilder.create().build()) {
SaasCallBackPayload payload = new SaasCallBackPayload();
payload.message = message;
if (success) {
payload.status = SUCCEEDED;
} else {
payload.status = FAILED;
}
payload.subscriptionUrl = subscriptionUrl;
ObjectMapper objectMapper = new ObjectMapper();
String jsonAsStr = objectMapper.writeValueAsString(payload);
HttpEntity entity = new StringEntity(jsonAsStr, ContentType.APPLICATION_JSON);
String jwt = jwtAccess.getJwt();
if (jwt == null || jwt.isEmpty()) {
throw new InternalError("Could not call saas registry as jwt is empty");
}
HttpUriRequest putRequest = RequestBuilder.put(new URL(completeUrl).toURI())
.setHeader(HttpHeaders.AUTHORIZATION, AUTHENTIFICATION_SCHEME + " " + jwt)
.setHeader(HttpHeaders.ACCEPT, APPLICATION_JSON)
.setHeader(HttpHeaders.CONTENT_TYPE, APPLICATION_JSON)
.setEntity(entity)
.build();
try (CloseableHttpResponse response = httpClient.execute(putRequest)) {
int responseCode = response.getStatusLine().getStatusCode();
if (responseCode != HttpStatus.SC_OK) {
throw new InternalError("Saas registry returned http status " + responseCode + " . Called saas registry url is " + completeUrl);
}
}
} catch (URISyntaxException e) {
throw new InternalError("Saas registry url " + callbackUrl + " has wrong format " +
" . Called saas registry url is " + completeUrl, e);
} catch (IOException | CommunicationError e) {
throw new InternalError("Could not call saas registry. Reason is " + e.getMessage() +
" . Called saas registry url is " + completeUrl, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy