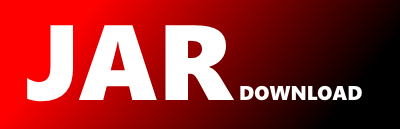
com.sap.mobile.services.client.push.ApnsNotification Maven / Gradle / Ivy
The newest version!
package com.sap.mobile.services.client.push;
import java.util.Date;
import java.util.List;
import lombok.AccessLevel;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.RequiredArgsConstructor;
/**
* The APNS specific notification request element. Use the
* {@link Builder} to define and build the APNS specific notification element.
*/
public interface ApnsNotification {
static Builder builder() {
return new Builder();
}
Date getExpiration();
String getCategory();
Boolean getContentAvailable();
PushType getPushType();
String getCustomValues();
String getSound();
ApnsCustomSound getCustomSound();
String getTopic();
String getAlertBody();
String getLocalizedAlertKey();
List getLocalizedAlertArguments();
String getAlertTitle();
String getLocalizedAlertTitleKey();
List getLocalizedAlertTitleArguments();
String getAlertSubtitle();
String getLocalizedAlertSubtitleKey();
List getLocalizedAlertSubtitleArguments();
String getLaunchImageFileName();
Boolean getShowActionButton();
String getActionButtonLabel();
String getLocalizedActionButtonKey();
List getUrlArguments();
String getThreadId();
Boolean getMutableContent();
InterruptionLevel getInterruptionLevel();
String getTargetContentId();
Float getRelevanceScore();
/**
* APNS specific notification properties. See
*
* @see
* Generating a remote notification.
*/
@NoArgsConstructor(access = AccessLevel.PRIVATE)
@AllArgsConstructor(access = AccessLevel.PRIVATE)
final class Builder {
private Date expiration;
private String category;
private Boolean contentAvailable;
private PushType pushType;
private String customValues;
private String sound;
private ApnsCustomSound customSound;
private String topic;
private String alertBody;
private String localizedAlertKey;
private List localizedAlertArguments;
private String alertTitle;
private String localizedAlertTitleKey;
private List localizedAlertTitleArguments;
private String alertSubtitle;
private String localizedAlertSubtitleKey;
private List localizedAlertSubtitleArguments;
private String launchImageFileName;
private Boolean showActionButton;
private String actionButtonLabel;
private String localizedActionButtonKey;
private List urlArguments;
private String threadId;
private Boolean mutableContent;
private InterruptionLevel interruptionLevel;
private String targetContentId;
private Float relevanceScore;
/**
* expire notification at given timestamp
*/
public Builder expiration(Date expiration) {
return new Builder(expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Mobile apps can register actions during launch grouped as category. This
* value allows actions to be executed directly by the notification in the
* notification center.
*/
public Builder category(String category) {
return new Builder(this.expiration, category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Content available marks background notification without visual text.
*/
public Builder contentAvailable(Boolean contentAvailable) {
return new Builder(this.expiration, this.category, contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Push Type, see
*
* @see
* Sending notification requests
*/
public Builder pushType(PushType pushType) {
return new Builder(this.expiration, this.category, this.contentAvailable, pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Custom values for APNS only
*/
public Builder customValues(String customValues) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Reference to a sound resource in the app. default will play the system sound
*
* @deprecated please use {@link Builder#customSound}
*/
public Builder sound(String sound) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Detailed APNS sound definition. Use this when sending critical
* notifications.
*/
public Builder customSound(ApnsCustomSound customSound) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The topic for the notification. In general, the topic is your app’s bundle
* ID/app ID. It can have a suffix based on the type of push notification.
*/
public Builder topic(String topic) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The notification body. {@link Builder#localizedAlertKey}
* should
* be used to retrieve the subtitle from the localized resources of the app
* based on the user's language configuration when sending templated messages.
*/
public Builder alertBody(String alertBody) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Should be used to retrieve the title from the localized resources of the app
* based on the user's language configuration.
*/
public Builder localizedAlertKey(String localizedAlertKey) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Localization arguments for the body
*/
public Builder localizedAlertArguments(List localizedAlertArguments) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The notification title.
* {@link Builder#localizedAlertTitleKey} should
* be used to retrieve the subtitle from the localized resources of the app
* based on the user's language configuration when sending templated messages.
*/
public Builder alertTitle(String alertTitle) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Should be used to retrieve the title from the localized resources of the app
* based on the user's language configuration.
*/
public Builder localizedAlertTitleKey(String localizedAlertTitleKey) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Localization arguments for the title
*/
public Builder localizedAlertTitleArguments(List localizedAlertTitleArguments) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The notification subtitle.
* {@link Builder#localizedAlertSubtitleKey} should
* be used to retrieve the subtitle from the localized resources of the app
* based on the user's language configuration when sending templated messages.
*/
public Builder alertSubtitle(String alertSubtitle) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Should be used to retrieve the subtitle from the localized resources of the
* app
* based on the user's language configuration.
*/
public Builder localizedAlertSubtitleKey(String localizedAlertSubtitleKey) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Localization arguments for the subtitle
*/
public Builder localizedAlertSubtitleArguments(List localizedAlertSubtitleArguments) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The name of the image or storyboard to use when your app launches because of
* the notification.
*/
public Builder launchImageFileName(String launchImageFileName) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Safari only: Show notification action button
*/
public Builder showActionButton(Boolean showActionButton) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Safari only: The literal text of the action button to be shown for the push
* notification.
*/
public Builder actionButtonLabel(String actionButtonLabel) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Safari only: Localized key of the action button to be shown for the push
* notification.
*/
public Builder localizedActionButtonKey(String localizedActionButtonKey) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* List of arguments to populate placeholders in the urlFormatString associated
* with a Safari push notification.
*/
public Builder urlArguments(List urlArguments) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Thread-Ids allows grouping of notification on application level
*/
public Builder threadId(String threadId) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* Notifications with mutable content set and no visual alert is defined are
* processed by the mobile app, prior displayed in the notification center
*/
public Builder mutableContent(Boolean mutableContent) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The interruption-level is optional and defines the importance and delivery
* timing of a notification
*/
public Builder interruptionLevel(InterruptionLevel interruptionLevel) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, interruptionLevel, this.targetContentId, this.relevanceScore);
}
/**
* The identifier of the window brought forward. The value of this key will be
* populated on the UNNotificationContent object created from the push payload.
*/
public Builder targetContentId(String targetContentId) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, targetContentId, this.relevanceScore);
}
/**
* The relevance score, a number between 0 and 1, that the system uses to sort
* the notifications from your app. The highest score gets featured in the
* notification summary.
*/
public Builder relevanceScore(Float relevanceScore) {
return new Builder(this.expiration, this.category, this.contentAvailable, this.pushType, this.customValues,
this.sound, this.customSound, this.topic, this.alertBody, this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, relevanceScore);
}
public ApnsNotification build() {
return new ApnsNotificationObject(this.expiration, this.category, this.contentAvailable, this.pushType,
this.customValues, this.sound, this.customSound, this.topic, this.alertBody,
this.localizedAlertKey,
this.localizedAlertArguments, this.alertTitle, this.localizedAlertTitleKey,
this.localizedAlertTitleArguments, this.alertSubtitle, this.localizedAlertSubtitleKey,
this.localizedAlertSubtitleArguments, this.launchImageFileName, this.showActionButton,
this.actionButtonLabel, this.localizedActionButtonKey, this.urlArguments, this.threadId,
this.mutableContent, this.interruptionLevel, this.targetContentId, this.relevanceScore);
}
@Getter
@RequiredArgsConstructor(access = AccessLevel.PRIVATE)
private static class ApnsNotificationObject implements ApnsNotification {
private final Date expiration;
private final String category;
private final Boolean contentAvailable;
private final PushType pushType;
private final String customValues;
private final String sound;
private final ApnsCustomSound customSound;
private final String topic;
private final String alertBody;
private final String localizedAlertKey;
private final List localizedAlertArguments;
private final String alertTitle;
private final String localizedAlertTitleKey;
private final List localizedAlertTitleArguments;
private final String alertSubtitle;
private final String localizedAlertSubtitleKey;
private final List localizedAlertSubtitleArguments;
private final String launchImageFileName;
private final Boolean showActionButton;
private final String actionButtonLabel;
private final String localizedActionButtonKey;
private final List urlArguments;
private final String threadId;
private final Boolean mutableContent;
private final InterruptionLevel interruptionLevel;
private final String targetContentId;
private final Float relevanceScore;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy