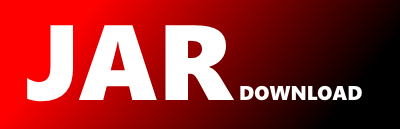
com.sap.cloud.sdk.cloudplatform.metering.OAuthTokenCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of metering-scp-neo Show documentation
Show all versions of metering-scp-neo Show documentation
Implementation of the Cloud platform abstraction for API metering functionality
on the SAP Cloud Platform (Neo).
/*
* Copyright (c) 2018 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.cloudplatform.metering;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URI;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpUriRequest;
import org.slf4j.Logger;
import com.google.common.base.Charsets;
import com.google.gson.Gson;
import com.netflix.hystrix.HystrixCommandGroupKey;
import com.sap.cloud.sdk.cloudplatform.connectivity.Destination;
import com.sap.cloud.sdk.cloudplatform.connectivity.DestinationAccessor;
import com.sap.cloud.sdk.cloudplatform.connectivity.HttpClientAccessor;
import com.sap.cloud.sdk.cloudplatform.logging.CloudLoggerFactory;
import com.sap.cloud.sdk.frameworks.hystrix.Command;
import com.sap.cloud.sdk.frameworks.hystrix.HystrixUtil;
class OAuthTokenCommand extends Command
{
private static final Logger logger = CloudLoggerFactory.getLogger(OAuthTokenCommand.class);
OAuthTokenCommand()
{
super(HystrixCommandGroupKey.Factory.asKey(HystrixUtil.getGlobalKey(OAuthTokenCommand.class)), 5000);
}
@Override
protected OAuthToken run()
throws IOException
{
final String destinationName = AccessTokenDestination.getDefaultName();
final Destination destination = DestinationAccessor.getDestination(destinationName);
final HttpClient httpClient = HttpClientAccessor.getHttpClient(destination);
final URI uri = destination.getUri();
if( logger.isDebugEnabled() ) {
logger.debug("URI from OAuth token destination: " + uri + ".");
}
final HttpUriRequest request = new HttpPost();
final HttpResponse response = httpClient.execute(request);
final HttpEntity entity = response.getEntity();
try( final InputStream stream = entity.getContent() ) {
return new Gson().fromJson(new InputStreamReader(stream, Charsets.UTF_8), OAuthToken.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy