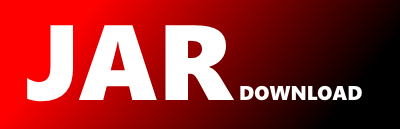
com.sap.cloud.sdk.cloudplatform.servlet.Property Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of servlet Show documentation
Show all versions of servlet Show documentation
Cloud platform abstraction for servlet functionality.
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.cloudplatform.servlet;
import javax.annotation.Nullable;
import lombok.EqualsAndHashCode;
import lombok.Getter;
/**
* Represents a {@link RequestContext} property with a given value (or exception, if the value could not be determined).
*/
@EqualsAndHashCode
@Getter
public class Property
{
@Nullable
private final T value;
@Nullable
private final Exception exception;
private final boolean isConfidential;
/**
* Creates a property.
*
* @param value
* The value of the property, if present.
* @param exception
* The exception that has occurred while retrieving the value, if present.
* @param isConfidential
* Whether the property must be handled confidentially, e.g., it should not be written to log files and
* {@link #toString()} should not reveal the value or exception.
*/
protected Property( @Nullable final T value, @Nullable final Exception exception, final boolean isConfidential )
{
this.value = value;
this.exception = exception;
this.isConfidential = isConfidential;
}
/**
* Creates an empty property.
*/
public static Property empty()
{
return new Property<>(null, null, false);
}
/**
* Creates a non-confidential property from the given value.
*/
public static Property ofValue( @Nullable final T value )
{
return new Property<>(value, null, false);
}
/**
* Creates a non-confidential property from the given exception.
*/
public static Property ofException( @Nullable final Exception exception )
{
return new Property<>(null, exception, false);
}
/**
* Creates a confidential property from the given value.
*/
public static Property ofConfidentialValue( @Nullable final T confidentialValue )
{
return new Property<>(confidentialValue, null, true);
}
/**
* Creates a confidential property from the given exception.
*/
public static Property ofConfidentialException( @Nullable final Exception confidentialException )
{
return new Property<>(null, confidentialException, true);
}
@Override
public String toString()
{
return isConfidential
? "Property(value="
+ (value != null ? "(hidden)" : null)
+ ", exception="
+ (exception != null ? "(hidden)" : null)
+ ")"
: "Property(value=" + value + ", exception=" + exception + ")";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy