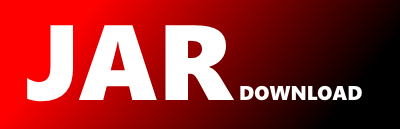
com.sap.cloud.sdk.frameworks.hystrix.ScpNeoHystrixConcurrencyStrategy Maven / Gradle / Ivy
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.frameworks.hystrix;
import java.util.concurrent.Callable;
import org.slf4j.Logger;
import com.netflix.hystrix.strategy.concurrency.HystrixConcurrencyStrategy;
import com.sap.cloud.sdk.cloudplatform.concurrency.ScpNeoTenantCallable;
import com.sap.cloud.sdk.cloudplatform.concurrency.ScpNeoUserSessionCallable;
import com.sap.cloud.sdk.cloudplatform.exception.ShouldNotHappenException;
import com.sap.cloud.sdk.cloudplatform.logging.CloudLoggerFactory;
import com.sap.cloud.sdk.cloudplatform.security.user.ScpNeoUser;
import com.sap.cloud.sdk.cloudplatform.security.user.User;
import com.sap.cloud.sdk.cloudplatform.security.user.UserAccessor;
import com.sap.cloud.sdk.cloudplatform.security.user.exception.UserAccessException;
import com.sap.cloud.sdk.cloudplatform.security.user.exception.UserNotAuthenticatedException;
import com.sap.cloud.sdk.cloudplatform.servlet.RequestContextAccessor;
import com.sap.cloud.sdk.cloudplatform.tenant.ScpNeoTenant;
import com.sap.cloud.sdk.cloudplatform.tenant.Tenant;
import com.sap.cloud.sdk.cloudplatform.tenant.TenantAccessor;
import com.sap.cloud.sdk.cloudplatform.tenant.exception.TenantAccessException;
import com.sap.cloud.sdk.cloudplatform.tenant.exception.TenantNotAvailableException;
/**
* Implementation of a {@link HystrixConcurrencyStrategy} that adapts to the multi-tenant environment of SAP CP Neo,
* initializing request variables and running the respective Hystrix {@link Callable}s within a request's tenant and
* user context.
*/
public class ScpNeoHystrixConcurrencyStrategy extends HystrixConcurrencyStrategy
{
private static final Logger logger = CloudLoggerFactory.getLogger(ScpNeoHystrixConcurrencyStrategy.class);
private ScpNeoTenant getCurrentTenant()
throws TenantNotAvailableException,
TenantAccessException
{
final Tenant tenant = TenantAccessor.getCurrentTenant();
if( !(tenant instanceof ScpNeoTenant) ) {
throw new ShouldNotHappenException(
"The current "
+ Tenant.class.getSimpleName()
+ " is not an instance of "
+ ScpNeoTenant.class.getSimpleName()
+ ". Please make sure to specify a dependency to com.sap.cloud.s4hana.cloudplatform:tenant-scp-neo.");
}
return (ScpNeoTenant) tenant;
}
private ScpNeoUser getCurrentUser()
throws UserNotAuthenticatedException,
UserAccessException
{
final User user = UserAccessor.getCurrentUser();
if( !(user instanceof ScpNeoUser) ) {
throw new ShouldNotHappenException(
"The current "
+ User.class.getSimpleName()
+ " is not an instance of "
+ ScpNeoUser.class.getSimpleName()
+ ". Please make sure to specify a dependency to com.sap.cloud.s4hana.cloudplatform:security-scp-neo.");
}
return (ScpNeoUser) user;
}
@Override
public Callable wrapCallable( final Callable callable )
{
Callable userSessionCallable;
try {
userSessionCallable =
new ScpNeoUserSessionCallable<>(
RequestContextAccessor.getCurrentRequest().orElse(null),
getCurrentUser(),
callable);
if( logger.isDebugEnabled() ) {
logger.debug("Successfully wrapped callable in " + userSessionCallable + ".");
}
}
catch( final UserNotAuthenticatedException | UserAccessException e ) {
if( logger.isDebugEnabled() ) {
logger.debug(
"User not available. Skip wrapping of callable in "
+ ScpNeoUserSessionCallable.class.getSimpleName()
+ ".",
e);
}
userSessionCallable = callable;
}
Callable tenantCallable;
try {
tenantCallable = new ScpNeoTenantCallable<>(getCurrentTenant(), userSessionCallable);
if( logger.isDebugEnabled() ) {
logger.debug("Successfully wrapped callable in " + tenantCallable + ".");
}
}
catch( final TenantNotAvailableException | TenantAccessException e ) {
if( logger.isDebugEnabled() ) {
logger.debug(
"Tenant not available. Skip wrapping of callable in "
+ ScpNeoTenantCallable.class.getSimpleName()
+ ".",
e);
}
tenantCallable = userSessionCallable;
}
return tenantCallable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy