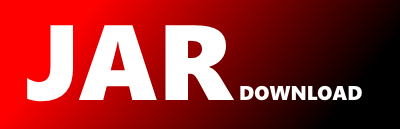
com.sap.cloud.sdk.services.recastai.DialogResponseWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai;
import java.util.Map;
import javax.annotation.Nullable;
import com.google.common.annotations.Beta;
import com.google.gson.Gson;
import com.google.gson.annotations.SerializedName;
import com.sap.cloud.sdk.services.recastai.botresponses.TextResponse;
import lombok.NoArgsConstructor;
/**
* This is a wrapper for a text response with just one message object to be sent to recast. Memory, conversation id and
* language are optional and don't need to be given. This is used internally for Recast Dialog, but can also be used for
* creating own messages.
*
* Be aware that this class is in Beta (as indicated by the annotation) and therefore subject to breaking changes.
*
*/
@Beta
@NoArgsConstructor
public class DialogResponseWrapper
{
private TextResponse message;
private Map memory;
@SerializedName( "conversation_id" )
private String conversationId;
private String language;
/**
* This function generates the json representation of this object and returns it as a string to the user.
*
* @return a stringyfied json of this object
*/
@Nullable
public String getResponse()
{
final Gson gson = new Gson();
return gson.toJson(this);
}
/**
* Returns the memory of this dialog response
*
* @return the memory as a map
*/
@Nullable
public Map getMemory()
{
return memory;
}
/**
* Setter to replace the current memory with a new memory.
*
* @param memory
* a memory containing the new memory
*/
public void setMemory( @Nullable final Map memory )
{
this.memory = memory;
}
/**
* Getter of the conversation id.
*
* @return a string with the conversation id
*/
@Nullable
public String getConversationId()
{
return conversationId;
}
/**
* Setter for the conversation id. This is used to identify the conversation the message should be sent to.
*
* @param conversationId
* the conversation id as a string
*/
public void setConversationId( @Nullable final String conversationId )
{
this.conversationId = conversationId;
}
/**
* This is the Getter for the Language and follows the ISO abbreviation standard.
*
* @return string abbreviation of the language
*/
@Nullable
public String getLanguage()
{
return language;
}
/**
* This function replaces the current language of the response
*
* @param language
* a string abbreviation of the language
*/
public void setLanguage( @Nullable final String language )
{
this.language = language;
}
/**
* This returns the current text response which would be sent to the dialog.
*
* @return the textResponse object
*/
@Nullable
public TextResponse getMessage()
{
return message;
}
/**
* Replaces the current TextResponse object with the new given TextResponse
*
* @param message
* the next TextResponse for this dialog.
*/
public void setMessage( @Nullable final TextResponse message )
{
this.message = message;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy