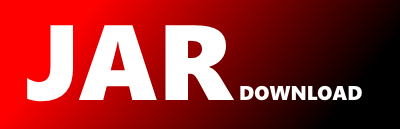
com.sap.cloud.sdk.services.recastai.RecastAnalyzer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai;
import javax.annotation.Nullable;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.BasicResponseHandler;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.slf4j.Logger;
import com.google.common.annotations.Beta;
import com.google.gson.Gson;
import com.sap.cloud.sdk.cloudplatform.exception.ShouldNotHappenException;
import com.sap.cloud.sdk.cloudplatform.logging.CloudLoggerFactory;
import com.sap.cloud.sdk.services.recastai.userrequest.NlpResponse;
/**
* Manages the natural language analysis of a sentence via recast.
*
* Be aware that this class is in Beta (as indicated by the annotation) and therefore subject to breaking changes.
*
*/
@Beta
public class RecastAnalyzer
{
private static final Logger logger = CloudLoggerFactory.getLogger(RecastAnalyzer.class);
private String uri = "https://api.recast.ai/v2/request";
private final String token;
/**
* creates an analyser for a trained bot on Recast
*
* @param token
* String representation of the token
*/
public RecastAnalyzer( final String token )
{
this.token = "Token " + token;
}
/**
* allow additional parameter in case a new API is released
*
* @param token
* String representation of the token
* @param uri
* the uri to use
*/
public RecastAnalyzer( final String token, final String uri )
{
this.token = "Token " + token;
this.uri = uri;
}
/**
* gets the analysis of the given sentence and returns an object with the parsed JSON
*
* @param content
* the sentence to be analysed
* @param language
* the language of the content
*
* @return return RecastAnalysis object
*
* @throws ShouldNotHappenException
* if an error occurs
*/
@Nullable
public NlpResponse getRecastAnalysis( final String content, final String language )
throws ShouldNotHappenException
{
try( final CloseableHttpClient client = HttpClients.createDefault() ) {
final NlpResponseWrapper nlpResponseWrapper = new NlpResponseWrapper(content, language);
final Gson gson = new Gson();
final StringEntity entity = new StringEntity(gson.toJson(nlpResponseWrapper));
final HttpPost httpPost = new HttpPost(uri);
httpPost.setEntity(entity);
httpPost.setHeader("Authorization", token);
httpPost.setHeader("Content-Type", "application/json");
//send request
final CloseableHttpResponse recastResponse = client.execute(httpPost);
//handle response
final BasicResponseHandler basicResponseHandler = new BasicResponseHandler();
final String myResponse = basicResponseHandler.handleResponse(recastResponse);
//wrap up
recastResponse.close();
return gson.fromJson(myResponse, NlpResponse.class);
}
catch( final Exception e ) {
final String errorMsg = "Failed to get recast analysis: " + e.getMessage();
logger.debug(errorMsg, e);
throw new ShouldNotHappenException(errorMsg, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy