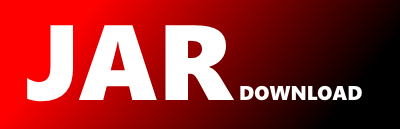
com.sap.cloud.sdk.services.recastai.RecastDialog Maven / Gradle / Ivy
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai;
import java.io.IOException;
import javax.annotation.Nullable;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.BasicResponseHandler;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.slf4j.Logger;
import com.google.common.annotations.Beta;
import com.google.gson.Gson;
import com.sap.cloud.sdk.cloudplatform.exception.ShouldNotHappenException;
import com.sap.cloud.sdk.cloudplatform.logging.CloudLoggerFactory;
import com.sap.cloud.sdk.services.recastai.botresponses.TextResponse;
import com.sap.cloud.sdk.services.recastai.userrequest.DialogResponse;
/**
* Manages the handling of a dialog in recast.
*
* Be aware that this class is in Beta (as indicated by the annotation) and therefore subject to breaking changes.
*
*/
@Beta
public class RecastDialog
{
private static final Logger logger = CloudLoggerFactory.getLogger(RecastDialog.class);
private String uri = "https://api.recast.ai/build/v1/dialog";
private final String token;
/**
* Initiates a Recast dialog object with URI="https://api.recast.ai/build/v1/dialog" and the given token.
*
* @param token
* the token to be used.
*/
public RecastDialog( final String token )
{
this.token = "Token " + token;
}
/**
* Initiates a Recast dialog object with URI given by the user.
*
* @param token
* the token to be used.
* @param uri
* the uri to be used
*/
public RecastDialog( final String token, final String uri )
{
this.token = "Token " + token;
this.uri = uri;
}
/**
* Sends a message and awaits a response from the bot
*
* @param message
* a single message to be sent
* @param conversationId
* the conversation the message should be send to
* @return the response from the bot, returns null in case of failure
* @throws ShouldNotHappenException
* if an error occurs
*/
@Nullable
public DialogResponse sendInteraction( final String message, final String conversationId )
throws ShouldNotHappenException
{
try( final CloseableHttpClient client = HttpClients.createDefault() ) {
final HttpPost httpPost = new HttpPost(uri);
final DialogResponseWrapper dialogResponseWrapper = new DialogResponseWrapper();
dialogResponseWrapper.setMessage(new TextResponse(message));
dialogResponseWrapper.setConversationId(conversationId);
httpPost.addHeader("Authorization", token);
httpPost.addHeader("Content-Type", "application/json");
httpPost.addHeader("Accept", "application/json");
return getDialogResponse(dialogResponseWrapper, client, httpPost);
}
catch( final IOException e ) {
// using ShouldNotHappenException here to remain compatible with the current API for now
throw new ShouldNotHappenException(e);
}
}
/**
* Sends a message and awaits a response from the bot, allows more freedom for memory settings
*
* @param dialogResponseWrapper
* a simple response as set by the user
* @return the response from the bot, returns null in case of failure
* @throws ShouldNotHappenException
* if an error occurs
*/
@Nullable
public DialogResponse sendInteraction( final DialogResponseWrapper dialogResponseWrapper )
throws ShouldNotHappenException
{
try( final CloseableHttpClient client = HttpClients.createDefault() ) {
final HttpPost httpPost = new HttpPost(uri);
httpPost.addHeader("Authorization", token);
httpPost.addHeader("Content-Type", "application/json");
httpPost.addHeader("Accept", "application/json");
return getDialogResponse(dialogResponseWrapper, client, httpPost);
}
catch( final IOException e ) {
// using ShouldNotHappenException here to remain compatible with the current API for now
throw new ShouldNotHappenException(e);
}
}
private DialogResponse getDialogResponse(
final DialogResponseWrapper dialogResponseWrapper,
final CloseableHttpClient client,
final HttpPost httpPost )
{
try {
final StringEntity entity = new StringEntity(dialogResponseWrapper.getResponse());
entity.setContentEncoding("UTF-8");
entity.setContentType("application/json");
httpPost.setEntity(entity);
final CloseableHttpResponse recastResponse = client.execute(httpPost);
final BasicResponseHandler basicResponseHandler = new BasicResponseHandler();
final String myResponse = basicResponseHandler.handleResponse(recastResponse);
recastResponse.close();
final Gson gson = new Gson();
return gson.fromJson(myResponse, DialogResponse.class);
}
catch( final Exception e ) {
final String errorMsg = "Failed to send interaction: " + e.getMessage();
logger.debug(errorMsg, e);
throw new ShouldNotHappenException(errorMsg, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy