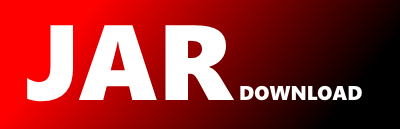
com.sap.cloud.sdk.services.recastai.RecastMessenger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai;
import javax.annotation.Nonnull;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.slf4j.Logger;
import com.google.common.annotations.Beta;
import com.sap.cloud.sdk.cloudplatform.exception.ShouldNotHappenException;
import com.sap.cloud.sdk.cloudplatform.logging.CloudLoggerFactory;
import com.sap.cloud.sdk.services.recastai.botresponses.ResponseInterface;
/**
* Sends messages to the given conversation id if new versions of api are released the uri can be adapted.
*
* Be aware that this class is in Beta (as indicated by the annotation) and therefore subject to breaking changes.
*
*/
@Beta
public class RecastMessenger
{
private static final Logger logger = CloudLoggerFactory.getLogger(RecastMessenger.class);
private String uriStart = "https://api.recast.ai/connect/v1/conversations/";
private String uriEnd = "/messages";
private String broadcastUri = "https://api.recast.ai/connect/v1/messages";
private final String token;
/**
* Allows to create an object for communication for sending messages to a conversation.
*
* @param token
* the plain token as given by recast without "Token"
*/
public RecastMessenger( @Nonnull final String token )
{
this.token = "Token " + token;
}
/**
* note that start and string url each have to start and end with a / save "the.start/uri/" and "/uriEnd" the final
* composition with the conversation Id is: "the.start/uri/{conversationID}/uriEnd"
*
* @param token
* the token to be used
* @param uriStart
* the start/beginning of the uri as given in the example above
* @param uriEnd
* the ending of the uri as given in the example above
*/
public RecastMessenger( @Nonnull final String token, @Nonnull final String uriStart, @Nonnull final String uriEnd )
{
this.token = "Token " + token;
this.uriStart = uriStart;
this.uriEnd = uriEnd;
}
/**
* Allows the creation with a new broadcast uri
*
* @param token
* the token as taken from Recast without "Token"
* @param broadcastUri
* the uri as a string
*/
public RecastMessenger( @Nonnull final String token, @Nonnull final String broadcastUri )
{
this.broadcastUri = broadcastUri;
this.token = "Token " + token;
}
/**
* Sends an array of Messages to the given conversation
*
* @param conversationId
* the conversation id as a string
* @param responseMessages
* an array of response messages. Can include multiple of texts, cards, ...
* @return the status code. If no conversation was found 404 is returned. 201 is a successful transmission
* @throws ShouldNotHappenException
* if an error occurs
*/
public int sendMessage( @Nonnull final String conversationId, @Nonnull final ResponseInterface... responseMessages )
throws ShouldNotHappenException
{
try( final CloseableHttpClient client = HttpClients.createDefault() ) {
final HttpPost httpPost = new HttpPost(uriStart + conversationId + uriEnd);
final MessageResponseWrapper messageResponseWrapper = new MessageResponseWrapper();
messageResponseWrapper.setMessages(responseMessages);
if( messageResponseWrapper.getResponse() == null ) {
return -1;
}
final StringEntity entity = new StringEntity(messageResponseWrapper.getResponse());
httpPost.setEntity(entity);
httpPost.setHeader("Authorization", token);
httpPost.setHeader("Content-Type", "application/json");
final CloseableHttpResponse recast_response = client.execute(httpPost);
return recast_response.getStatusLine().getStatusCode();
}
catch( final Exception e ) {
final String errorMsg = "Failed to send message: " + e.getMessage();
logger.debug(errorMsg, e);
throw new ShouldNotHappenException(errorMsg, e);
}
}
/**
* broadcasts a message to all conversations
*
* @param responseMessages
* an array of response messages. Can include multiple of texts, cards, ...
* @return the status code as returned by recast. 201 is successful
* @throws ShouldNotHappenException
* if an error occurs
*/
public int broadcastMessage( @Nonnull final ResponseInterface... responseMessages )
throws ShouldNotHappenException
{
try( final CloseableHttpClient client = HttpClients.createDefault() ) {
final HttpPost httpPost = new HttpPost(broadcastUri);
final MessageResponseWrapper messageResponseWrapper = new MessageResponseWrapper();
messageResponseWrapper.setMessages(responseMessages);
if( messageResponseWrapper.getResponse() == null ) {
return -1;
}
final StringEntity entity = new StringEntity(messageResponseWrapper.getResponse());
httpPost.setEntity(entity);
httpPost.setHeader("Authorization", token);
httpPost.setHeader("Content-Type", "application/json");
final CloseableHttpResponse recast_response = client.execute(httpPost);
return recast_response.getStatusLine().getStatusCode();
}
catch( final Exception e ) {
final String errorMsg = "Failed to broadcast message: " + e.getMessage();
logger.debug(errorMsg, e);
throw new ShouldNotHappenException(errorMsg, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy