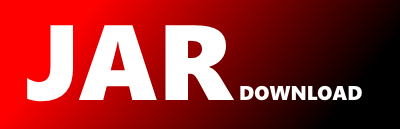
com.sap.cloud.sdk.services.recastai.RecastWebhookData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.google.common.annotations.Beta;
import com.google.gson.Gson;
import com.sap.cloud.sdk.services.recastai.userrequest.Conversation;
import com.sap.cloud.sdk.services.recastai.userrequest.Nlp;
/**
* Use this to parse the data from a webhook request your extension should respond to.
*/
@Beta
public class RecastWebhookData
{
private Conversation conversation;
private Nlp nlp;
private String actionId;
/**
* Use this to parse the content provided by recast in a webhook request.
*
* @param recastData
* the raw json data available in the webhook request body.
* @return newly created RecastWebhookData object.
*/
@Nonnull
public static RecastWebhookData parseString( @Nonnull final String recastData )
{
return new Gson().fromJson(recastData, RecastWebhookData.class);
}
/**
* Get the conversation information from recast, such as the memory status.
*
* @return the conversation object.
*/
@Nullable
public Conversation getConversation()
{
return conversation;
}
/**
* Set conversation information.
*
* @param conversation
* the conversation information to set
*/
public void setConversation( @Nullable final Conversation conversation )
{
this.conversation = conversation;
}
/**
* Get the natural language processing information from recast.
*
* @return nlp object with recognized entities and intent(s).
*/
@Nullable
public Nlp getNlp()
{
return nlp;
}
/**
* Set the nlp information
*
* @param nlp
* to set
*/
public void setNlp( @Nullable final Nlp nlp )
{
this.nlp = nlp;
}
/**
* Access to the id of the action.
*
* @return action id string
*/
@Nullable
public String getActionId()
{
return actionId;
}
/**
* Set the action id.
*
* @param actionId
* to set
*/
public void setActionId( @Nullable final String actionId )
{
this.actionId = actionId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy