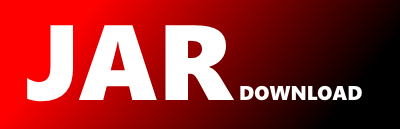
com.sap.cloud.sdk.services.recastai.RecastWebhookResponseWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai;
import java.util.Arrays;
import java.util.List;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.google.common.annotations.Beta;
import com.google.gson.Gson;
import com.sap.cloud.sdk.services.recastai.botresponses.ConversationUpdate;
import com.sap.cloud.sdk.services.recastai.botresponses.ResponseInterface;
/**
* Use this to create the response JSON for a recast webhook request.
*/
@Beta
public class RecastWebhookResponseWrapper
{
private final List replies;
private ConversationUpdate conversation;
/**
* Compose the reply to a webhook request.
*
* @param replies
* required replies
* @param conversation
* optional. The information to add to/change in the conversation.
*/
public RecastWebhookResponseWrapper(
@Nonnull final List replies,
@Nullable final ConversationUpdate conversation )
{
this.replies = replies;
this.conversation = conversation;
}
/**
* Compose the reply to a webhook request. This constructor does not set a conversation update.
*
* @param replies
* required replies
*/
public RecastWebhookResponseWrapper( @Nonnull final ResponseInterface... replies )
{
this.replies = Arrays.asList(replies);
}
/**
* Set the ConversationUpdate to send to recast.
*
* @param conversation
* the information to add to/change in the conversation
*/
public void setConversation( @Nullable final ConversationUpdate conversation )
{
this.conversation = conversation;
}
/**
* Get the json string for this objet.
*
* @return json string
*/
@Nonnull
public String getResponse()
{
final Gson gson = new Gson();
return gson.toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy