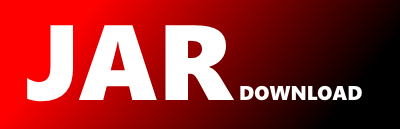
com.sap.cloud.sdk.services.recastai.botresponses.ButtonCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai.botresponses;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Nonnull;
import com.google.common.annotations.Beta;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
/**
* Collects buttons to be included in a Response and allows to set a title.
*
* @param
* the button type this collection contains.
*/
@Beta
public class ButtonCollection
{
@SerializedName( "title" )
@Expose
private String title;
@SerializedName( "buttons" )
@Expose
private final List buttons = new ArrayList();
/**
* Creates a new button collection
*
* @param title
* the title for this button collection
*/
public ButtonCollection( @Nonnull final String title )
{
this.title = title;
}
/**
* This function adds a button to the current button collection.
*
* @param button
* another slim button or superclass thereof
*/
public void appendButton( @Nonnull final B button )
{
buttons.add(button);
}
/**
* Funtion adds a string to the current title.
*
* @param append
* a string representation of the title
*/
public void appendToTitle( @Nonnull final String append )
{
title = title + append;
}
/**
* Get the buttons of the collection.
*
* @return the buttons list
*/
@Nonnull
public List getButtons()
{
return buttons;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy