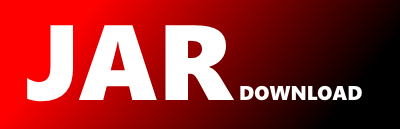
com.sap.cloud.sdk.services.recastai.botresponses.MediaResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai.botresponses;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.google.common.annotations.Beta;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
/**
* This class wraps around a URL to send a picture or video to a conversation.
*/
@Beta
public class MediaResponse implements ResponseInterface
{
@SerializedName( "type" )
@Expose
private final String type;
@SerializedName( "content" )
@Expose
private String content;
/**
* The contructor to generate a new video response with an URL.
*
* @param content
* a url to a video (playable in the users environment, probably by a browser)
*
* @return the newly created response
*/
@Nonnull
public static MediaResponse createVideoResponse( @Nonnull final String content )
{
return new MediaResponse(content, "video");
}
/**
* The contructor to generate a new picture response with an URL.
*
* @param content
* a url to a picture
*
* @return the newly created response
*/
@Nonnull
public static MediaResponse createPictureResponse( @Nonnull final String content )
{
return new MediaResponse(content, "picture");
}
/**
* The contructor to generate a new media Response with an URL
*
* @param content
* a url to an image
* @param type
* the type (picture or video)
*/
public MediaResponse( @Nonnull final String content, @Nonnull final String type )
{
this.content = content;
this.type = type;
}
/**
* Returns the content of this response.
*
* @return the url of this response
*/
@Nullable
public String getContent()
{
return content;
}
/**
* Function to allow the change of the content url
*
* @param content
* the new url
*/
public void setContent( @Nonnull final String content )
{
this.content = content;
}
/**
* returns the present type, which is immutable to the user. Follows the Response types of Recast
*
* @return the type of this response (picture or video)
*/
@Nullable
@Override
public String getType()
{
return type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy