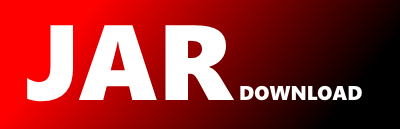
com.sap.cloud.sdk.services.recastai.userrequest.Nlp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai.userrequest;
import java.util.List;
import java.util.Map;
import javax.annotation.Nullable;
import com.google.common.annotations.Beta;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
import lombok.NoArgsConstructor;
/**
* Represents the NLP analysis JSON Object as sent by Recast.
*/
@Beta
@NoArgsConstructor
public class Nlp
{
@SerializedName( "uuid" )
@Expose
private String uuid;
@SerializedName( "source" )
@Expose
private String source;
@SerializedName( "intents" )
@Expose
private List intents = null;
@SerializedName( "act" )
@Expose
private String act;
@SerializedName( "type" )
@Expose
private Object type;
@SerializedName( "sentiment" )
@Expose
private String sentiment;
@SerializedName( "entities" )
@Expose
private Map>> entities;
@SerializedName( "language" )
@Expose
private String language;
@SerializedName( "processing_language" )
@Expose
private String processingLanguage;
@SerializedName( "version" )
@Expose
private String version;
@SerializedName( "timestamp" )
@Expose
private String timestamp;
@SerializedName( "status" )
@Expose
private Integer status;
/**
* Each input gets an UUID which can be obtained via this function.
*
* @return unique id as set by Recast
*/
@Nullable
public String getUuid()
{
return uuid;
}
/**
* This function returns the input sentence.
*
* @return a string of the input sentence
*/
@Nullable
public String getSource()
{
return source;
}
/**
* The list contains all identified intents
*
* @return a list will all intents, who each carry further information
*/
@Nullable
public List getIntents()
{
return intents;
}
/**
* The act of the request, more info on the recast website
*
* @return helps to classify the sentence
*/
@Nullable
public String getAct()
{
return act;
}
/**
* The type of the request, more info on the recast website
*
* @return helps to classify the sentence
*/
@Nullable
public Object getType()
{
return type;
}
/**
* This function returns the Sentiment as identified by recast.
*
* @return a adjective describing the sentiment
*/
@Nullable
public String getSentiment()
{
return sentiment;
}
/**
* Entities have different fields depending on the type. E.g. locations might have coordinates. This function
* returns a map with an array list of Maps for all the entities and their fields
*
* @return a map where the entitiy name serves as a key
*/
@Nullable
public Map>> getEntities()
{
return entities;
}
/**
* The language detected (or given) from the processed sentence, follows the ISO 639-1 standard
*
* @return a string abbreviation of the language
*/
@Nullable
public String getLanguage()
{
return language;
}
/**
* The language used to process the sentence, follows the ISO 639-1 standard
*
* @return a string abbreviation of the language
*/
@Nullable
public String getProcessingLanguage()
{
return processingLanguage;
}
/**
* The version of Recasts JSON, follows the Semantic Versioning Specification
*
* @return a string with the version number.
*/
@Nullable
public String getVersion()
{
return version;
}
/**
* The UTC timestamp at the end of Recasts processing, follows the ISO 8061 standard
*
* @return timestamp as a string
*/
@Nullable
public String getTimestamp()
{
return timestamp;
}
/**
* The status of Recasts Natural Language processor
*
* @return should be 200.
*/
@Nullable
public Integer getStatus()
{
return status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy