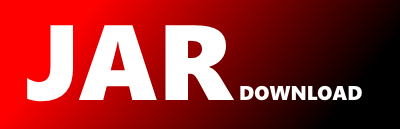
com.sap.cloud.sdk.services.recastai.userrequest.NlpResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of recast-ai Show documentation
Show all versions of recast-ai Show documentation
Integration of SAP Conversational AI, aka Recast AI
(Beta release, still subject to change - up to discontinuation of module).
/*
* Copyright (c) 2019 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.services.recastai.userrequest;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.annotation.Nullable;
import com.google.common.annotations.Beta;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
import lombok.NoArgsConstructor;
/**
* This object represents the results of a NLP analysis from Recast.
*/
@Beta
@NoArgsConstructor
public class NlpResults
{
@SerializedName( "uuid" )
@Expose
private String uuid;
@SerializedName( "source" )
@Expose
private String source;
@SerializedName( "intents" )
@Expose
private List intents = null;
@SerializedName( "act" )
@Expose
private String act;
@SerializedName( "type" )
@Expose
private Object type;
@SerializedName( "sentiment" )
@Expose
private String sentiment;
@SerializedName( "entities" )
@Expose
private Map>> entities;
@SerializedName( "language" )
@Expose
private String language;
@SerializedName( "processing_language" )
@Expose
private String processingLanguage;
@SerializedName( "version" )
@Expose
private String version;
@SerializedName( "timestamp" )
@Expose
private String timestamp;
@SerializedName( "status" )
@Expose
private Integer status;
/**
* The universally unique id for this request
*
* @return string representation of the UUID
*/
@Nullable
public String getUuid()
{
return uuid;
}
/**
* Returns the text Recast processed.
*
* @return the input text
*/
@Nullable
public String getSource()
{
return source;
}
/**
* The intents Recast found, sorted by probability
*
* @return a list of intent objects
*/
@Nullable
public List getIntents()
{
return intents;
}
/**
* The act of the request, more info on recasts website
*
* @return a string helping to classify the sentence
*/
@Nullable
public String getAct()
{
return act;
}
/**
* The type of the request, more info on recasts website
*
* @return a string helping to classify the sentence
*/
@Nullable
public Object getType()
{
return type;
}
/**
* The sentiment of the request, more info on recasts website
*
* @return a string helping to classify the sentence
*/
@Nullable
public String getSentiment()
{
return sentiment;
}
/**
* Every keys are an array of entity, more infos on recasts website
*
* @return a map with entity names as keys
*/
@Nullable
public Map>> getEntities()
{
return entities;
}
/**
* The language detected (or given) from the processed sentence, follows the ISO 639-1 standard
*
* @return string abbreviation of the language
*/
@Nullable
public String getLanguage()
{
return language;
}
/**
* The language used to process the sentence, follows the ISO 639-1 standard
*
* @return string abbreviation of the language
*/
@Nullable
public String getProcessingLanguage()
{
return processingLanguage;
}
/**
* The version of Recasts JSON, follows the Semantic Versioning Specification
*
* @return the version number wrapped in a string
*/
@Nullable
public String getVersion()
{
return version;
}
/**
* The UTC timestamp at the end of Recasts processing, follows the ISO 8061 standard
*
* @return the timestamp as string representation
*/
@Nullable
public String getTimestamp()
{
return timestamp;
}
/**
* The status of Recasts Natural Language processor
*
* @return should return 200
*/
@Nullable
public Integer getStatus()
{
return status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy