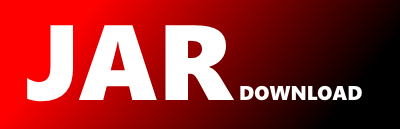
com.sap.cloud.sdk.s4hana.connectivity.ServiceUriBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connectivity Show documentation
Show all versions of connectivity Show documentation
Connectivity to SAP S/4HANA.
/*
* Copyright (c) 2018 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.s4hana.connectivity;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Set;
import com.google.common.collect.Sets;
/**
* Used to build S/4HANA service URI, considering parameters such as base URI and relative path.
*/
public class ServiceUriBuilder
{
protected static final String ERP_ENDPOINT_CLOUD = "/sap/bc/rest/sap/fi_map/";
protected static final String ERP_ENDPOINT_ON_PREMISE = "/sap/hcpint/map/bm/";
private static final Set testSystemPaths = Sets.newHashSet();
/**
* Adds a test system path. For internal use only.
*/
public static synchronized void registerTestSystem( final URI uri )
{
testSystemPaths.add(encloseSlashesIfMissing(uri.getPath()));
}
protected static String prependSlashIfMissing( final String path )
{
return path.startsWith("/") ? path : "/" + path;
}
protected static String appendSlashIfMissing( final String path )
{
return path.endsWith("/") ? path : path + "/";
}
protected static String encloseSlashesIfMissing( final String path )
{
return prependSlashIfMissing(appendSlashIfMissing(path));
}
protected String removeErpEndpointPaths( final URI baseUri )
{
String path = encloseSlashesIfMissing(baseUri.getPath());
path = path.replaceAll(ERP_ENDPOINT_CLOUD, "");
path = path.replaceAll(ERP_ENDPOINT_ON_PREMISE, "");
for( final String testSystemPath : testSystemPaths ) {
path = path.replaceAll(testSystemPath, "");
}
return encloseSlashesIfMissing(path);
}
/**
* Builds a ERP service URI for a given relative path.
*/
public URI build( final URI baseUri, final String relativePath )
{
try {
final String path = removeErpEndpointPaths(baseUri) + relativePath.replaceFirst("^/", "");
return new URI(
baseUri.getScheme(),
baseUri.getUserInfo(),
baseUri.getHost(),
baseUri.getPort(),
path,
baseUri.getQuery(),
baseUri.getFragment());
}
catch( final URISyntaxException e ) {
throw new IllegalArgumentException("Failed to build ERP service URI.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy