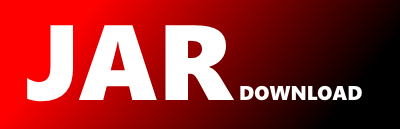
com.sap.cloud.sdk.cloudplatform.cache.CacheKey Maven / Gradle / Ivy
/*
* Copyright (c) 2020 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.cloud.sdk.cloudplatform.cache;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.sap.cloud.sdk.cloudplatform.security.principal.Principal;
import com.sap.cloud.sdk.cloudplatform.security.principal.PrincipalAccessor;
import com.sap.cloud.sdk.cloudplatform.security.principal.exception.PrincipalAccessException;
import com.sap.cloud.sdk.cloudplatform.tenant.Tenant;
import com.sap.cloud.sdk.cloudplatform.tenant.TenantAccessor;
import com.sap.cloud.sdk.cloudplatform.tenant.exception.TenantAccessException;
import io.vavr.control.Option;
import lombok.AccessLevel;
import lombok.AllArgsConstructor;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.ToString;
/**
* CacheKey with either global visibility, tenant isolation, or tenant and principal isolation.
*/
@EqualsAndHashCode
@ToString
@AllArgsConstructor( access = AccessLevel.PRIVATE )
public final class CacheKey implements GenericCacheKey
{
@Nullable
private final String tenantId;
@Nullable
private final String principalId;
@Getter
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy