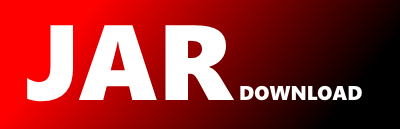
com.sap.cloud.security.ams.dcn.LocalDirectoryUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jakarta-ams Show documentation
Show all versions of jakarta-ams Show documentation
Client Library for integrating Jakarta EE applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.dcn;
import com.sap.cloud.security.ams.dcl.client.dcn.DcnContainer;
import com.sap.cloud.security.ams.dcl.client.dcn.DcnTools;
import com.sap.cloud.security.ams.dcl.spi.pdp.BundleStatusImpl;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* This is an implementation of the {@link BundleUpdater} interface that loads DCN content from a
* local directory.
*/
class LocalDirectoryUpdater implements BundleUpdater {
private final String dcnSrcPath;
private final DcnTools dcnTools;
private final Function> fileStringSupplier;
private final AtomicInteger successfulReads = new AtomicInteger(0);
static final Function> DEFAULT_FILE_STRING_SUPPLIER =
f -> {
try {
return Result.success(Files.readString(f));
} catch (IOException e) {
return Result.failure(new Error(e.getMessage()));
}
};
LocalDirectoryUpdater(String dcnSrcPath) {
this(dcnSrcPath, DcnTools.getInstance(), DEFAULT_FILE_STRING_SUPPLIER);
}
LocalDirectoryUpdater(
String dcnSrcPath,
DcnTools dcnTools,
Function> fileStringSupplier) {
super();
this.dcnSrcPath = dcnSrcPath;
this.dcnTools = dcnTools;
this.fileStringSupplier = fileStringSupplier;
}
Result>> dcnFilesFromPath() {
try (Stream pathStream = Files.walk(Paths.get(this.dcnSrcPath))) {
return Result.success(
pathStream
.filter(Files::isRegularFile)
.filter(f -> f.getFileName().toString().endsWith(".dcn"))
.collect(
Collectors.toMap(
f -> f.getParent().getFileName().toString() + "/" + f.getFileName(),
f -> {
try {
return new Result.Success<>(dcnTools.deserialize(f.toFile()));
} catch (IOException e) {
return new Result.Failure<>(new Error(e.getMessage()));
}
})));
} catch (IOException e) {
return Result.failure(new Error(e.getMessage()));
}
}
Result dataJsonFromPath() {
List dataJsonFiles;
try (Stream pathStream = Files.walk(Paths.get(this.dcnSrcPath))) {
dataJsonFiles =
pathStream
.filter(Files::isRegularFile)
.filter(f -> f.getFileName().toString().equals("data.json"))
.toList();
} catch (IOException e) {
return Result.failure(new Error(e.getMessage()));
}
if (dataJsonFiles.isEmpty()) {
return Result.success(DataJson.NO_DATA);
} else if (dataJsonFiles.size() == 1) {
var fileContent = fileStringSupplier.apply(dataJsonFiles.get(0));
if (fileContent.isFailure()) {
return Result.failure(fileContent.getError());
} else {
return Result.success(DataJson.fromJson(fileContent.getValue()));
}
} else {
return Result.failure(new Error("Unexpected number(>1) of data.json files found."));
}
}
Result getFromDirectory() {
var dcnFiles = dcnFilesFromPath();
var dataJson = dataJsonFromPath();
if (dcnFiles.isFailure()) {
return Result.failure(dcnFiles.getError());
}
if (dataJson.isFailure()) {
return Result.failure(dataJson.getError());
}
successfulReads.incrementAndGet();
return Result.success(
new BundleContent(new Repository(dcnFiles.getValue()), dataJson.getValue()));
}
@Override
public void syncGet(EngineDataHolder engineDataHolder, boolean compatibilityMode) {
var bundleContent = getFromDirectory();
var now = Instant.now();
var bs = new BundleStatusImpl();
bs.setName(this.dcnSrcPath);
bs.setLastRequest(now);
if (bundleContent.isFailure()) {
bs.setBundleError(true);
bs.setMessage(bundleContent.getError().errorMsg());
engineDataHolder.setBundleStatus(bs);
} else {
BundleUpdaterTools.setBundleStatusTimesToNow(bs);
BundleUpdaterTools.setNoError(bs);
var newEngine =
DcnPolicyDecisionPoint.createEngineAndDataJson(
bundleContent.getValue().repository().getDcnRepository(),
bundleContent.getValue().dataJson(),
compatibilityMode);
engineDataHolder.setEngineData(newEngine.getLeft(), newEngine.getRight());
engineDataHolder.setBundleStatus(bs);
}
}
@Override
public void startPeriodicUpdates(EngineDataHolder engineDataHolder, boolean compatibilityMode) {
syncGet(engineDataHolder, compatibilityMode);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy