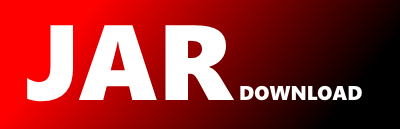
com.sap.cloud.security.ams.dcn.engine.AnyOfNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jakarta-ams Show documentation
Show all versions of jakarta-ams Show documentation
Client Library for integrating Jakarta EE applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.dcn.engine;
import static com.sap.cloud.security.ams.dcl.client.el.Call.QualifiedNames.OR;
import com.sap.cloud.security.ams.dcl.client.el.Call;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
/** {@link EvaluationNode} implementation of the n-ary logical AND operator. */
public class AnyOfNode extends EvaluationNode {
private final Set subNodes = new HashSet<>();
private AnyOfConditionWriter conditionWriter;
/**
* Creates a new instance having the given sub nodes. By default, a call to {@link #toCondition()}
* will convert the node into a {@link Call} object with name {@link Call.QualifiedNames#OR} while
* recursively applying {@link #toCondition()} to its sub nodes. If there are no sub nodes, the
* {@link AllOfNode} node will be converted to false. If there is only a single sub node, the node
* will be converted to the condition of that sub node. To override this behavior, use {@link
* #writtenBy} to set an alternate condition writer.
*
* @param subNodes the sub nodes
*/
AnyOfNode(Collection subNodes) {
this(
subNodes,
(nodes) ->
switch (nodes.size()) {
case 0 -> false;
case 1 -> nodes.iterator().next().toCondition();
default ->
Call.createFrom(OR, nodes.stream().map(EvaluationNode::toCondition).toList());
});
}
private AnyOfNode(Collection subNodes, AnyOfConditionWriter conditionWriter) {
this.subNodes.addAll(subNodes);
this.conditionWriter = conditionWriter;
}
/**
* Sets an alternate condition writer for this node which is called by {@link #toCondition()}.
* This is used to apply post-evaluation transformations which establish OPA-compatibility.
*
* @param conditionWriter the condition writer
* @return this node
*/
AnyOfNode writtenBy(AnyOfConditionWriter conditionWriter) {
this.conditionWriter = conditionWriter;
return this;
}
@Override
public EvaluationNode deepSimplify() {
return new AnyOfNode(
subNodes.stream().map(EvaluationNode::deepSimplify).toList(), conditionWriter)
.simplify();
}
/**
* Simplifies this node by merging sub nodes and removing redundant nodes. While sub nodes that
* evaluate to {@link ValueNode#FALSE} are ignored, a single sub node that evaluates to {@link
* ValueNode#TRUE} makes this method return {@link ValueNode#TRUE} as well. Sub nodes that are of
* type {@link AnyOfNode} are removed and their sub nodes are handled as if they were direct sub
* nodes of this node. All remaining sub nodes are merged with each other if possible (see {@link
* EvaluationNode#orMerge}) and collected into a set of new sub nodes. If the set of new sub nodes
* is empty, this method returns {@link ValueNode#FALSE}. If the set of new sub nodes contains
* only a single node, this method returns that node. Otherwise, this method returns a new {@link
* AnyOfNode} instance with the new sub nodes.
*
* @return the simplified node
*/
@Override
public EvaluationNode simplify() {
Set newNodes = new HashSet<>();
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy