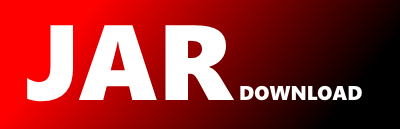
com.sap.cloud.security.ams.dcn.engine.EffectivePoliciesNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jakarta-ams Show documentation
Show all versions of jakarta-ams Show documentation
Client Library for integrating Jakarta EE applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.dcn.engine;
import static com.sap.cloud.security.ams.dcl.client.pdp.Attributes.Names.DCL_POLICIES;
import com.sap.cloud.security.ams.dcl.client.pdp.Attributes;
import java.util.Optional;
/**
* Leaf {@link EvaluationNode} representing the effective policies of the current principal. Unless
* the policies are overridden using the attribute $dcl.policies, the reference in
* $dcl.principal2policies is used to retrieve the policies from the data.json.
*/
public class EffectivePoliciesNode extends EvaluationNode {
private final EvaluationNode dclPoliciesAttributeNode;
EffectivePoliciesNode(EvaluationNodeFactory factory) {
this.dclPoliciesAttributeNode = factory.attributeNode(DCL_POLICIES);
}
/**
* If the policies to be used are overridden (or set to UNKNOWN / IGNORE) using the $dcl.policies
* attribute, then this node evaluates to the evaluation result of $dcl.policies. Otherwise (i.e.
* if $dcl.policies is UNSET), this node evaluates to the policies of the current principal as
* referenced by the $dcl.principal2policies attribute.
*
* @param valueAccessor accessor for attribute values and principal to policies mapping
* @return the effective policies evaluation result
*/
@Override
public EvaluationNode evaluate(ValueAccessor valueAccessor) {
EvaluationNode dclPolicies = dclPoliciesAttributeNode.evaluate(valueAccessor);
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy