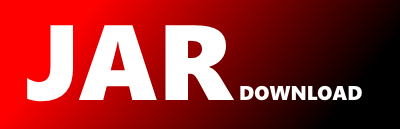
com.sap.cloud.security.ams.dcn.engine.EvaluationNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jakarta-ams Show documentation
Show all versions of jakarta-ams Show documentation
Client Library for integrating Jakarta EE applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.dcn.engine;
import java.util.Optional;
/**
* Base class for all nodes in the evaluation tree (see {@link Engine}).
*
* Inner nodes represent logical and value operators (e.g. AND, OR, IN) and hold references to
* sub nodes representing their operands. Except for a special node used for policy selection (see
* {@link EffectivePoliciesNode}), all leaf nodes are either representing a concrete value (of
* boolean, number, string or array type) or an unresolved attribute (e.g. $app.foo).
*/
public abstract class EvaluationNode {
/**
* Returns an empty optional unless this node represents a concrete value.
*
* @see ValueNode#value()
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy