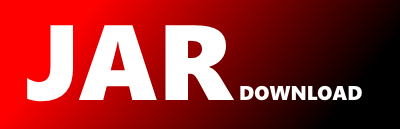
com.sap.cloud.security.ams.dcn.engine.IsNullOperatorNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jakarta-ams Show documentation
Show all versions of jakarta-ams Show documentation
Client Library for integrating Jakarta EE applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.dcn.engine;
import static com.sap.cloud.security.ams.dcl.client.el.Call.QualifiedNames.IS_NULL;
import com.sap.cloud.security.ams.dcl.client.el.Call;
import java.util.Objects;
import java.util.Optional;
/** {@link EvaluationNode} implementation of the IS_NULL operator. */
public class IsNullOperatorNode extends EvaluationNode {
private final EvaluationNode subNode;
/**
* Creates a new IS_NULL operator node for the given sub node.
*
* @param subNode the node to apply the IS_NULL operator to
*/
IsNullOperatorNode(EvaluationNode subNode) {
this.subNode = subNode;
}
@Override
public EvaluationNode deepSimplify() {
return new IsNullOperatorNode(subNode.deepSimplify());
}
/**
* This method returns {@link ValueNode#TRUE} if the sub node evaluates to {@link
* ValueNode#UNSET}, it returns {@link ValueNode#IGNORE} if the sub node evaluates to {@link
* ValueNode#IGNORE}, and it returns {@link ValueNode#FALSE} if the sub node evaluates to any
* other {@link ValueNode}. Otherwise, it returns a new {@link IsNullOperatorNode} instance for
* the evaluation result of the sub node.
*
* @param valueAccessor accessor for attribute values
* @return the result of the IS_NULL operator
*/
@Override
public EvaluationNode evaluate(ValueAccessor valueAccessor) {
EvaluationNode node = subNode.evaluate(valueAccessor);
if (ValueNode.UNSET.equals(node)) {
return ValueNode.TRUE;
}
if (ValueNode.IGNORE.equals(node)) {
return ValueNode.IGNORE;
}
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy