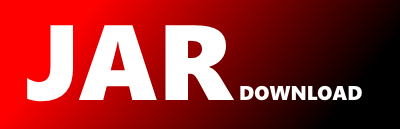
com.sap.cloud.security.ams.factory.AmsPolicyDecisionPointFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jakarta-ams Show documentation
Show all versions of jakarta-ams Show documentation
Client Library for integrating Jakarta EE applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2023 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.factory;
import com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPoint;
import com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPointFactory;
import com.sap.cloud.security.ams.logging.PolicyEvaluationSlf4jLogger;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
/**
* This shall be called with {@code PolicyDecisionPoint.create(DEFAULT)}. It is
* registered as service to
* {@code META-INF.services/com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPointFactory}.
*/
public class AmsPolicyDecisionPointFactory extends PolicyDecisionPointFactory {
/**
* Choose this {@code PolicyDecisionPoint} kind, in case you like to leverage
* the policy engine that is available on the url provided with {@code ADC_URL}
* system environment variable. This is the default on Cloud Foundry
* environment, when running using the buildpack.
*/
public static final String DEFAULT = "default";
public static final String ENV_ADC_URL = "ADC_URL";
private static final Logger LOGGER = LoggerFactory.getLogger(AmsPolicyDecisionPointFactory.class);
private static final String DCL_RUNTIME_CLIENT = "client:opa";
private static final Map CACHE = new ConcurrentHashMap<>();
private static final int PRIORITY = 100;
@Override
protected PolicyDecisionPoint tryCreate(String kind, Object[] arguments) {
if (DEFAULT.equalsIgnoreCase(kind)) {
Object[] extArguments = Arrays.copyOf(arguments, arguments.length + 2);
extArguments[arguments.length] = "url";
extArguments[arguments.length + 1] = getUrl();
return getOrCreate(DCL_RUNTIME_CLIENT, extArguments);
}
return null; // can not handle this kind
}
@Override
protected int getPriority() {
return PRIORITY;
}
PolicyDecisionPoint getOrCreate(String kind, Object[] arguments) {
String key = kind + Arrays.toString(arguments);
PolicyDecisionPoint pdp = CACHE.get(key);
if (Objects.isNull(pdp) || pdp.isClosed()) {
synchronized (CACHE) {
pdp = CACHE.get(key);
if (Objects.isNull(pdp) || pdp.isClosed()) {
LOGGER.debug("instantiate PolicyDecisionPoint for kind {} and args {}.", DCL_RUNTIME_CLIENT,
arguments);
pdp = PolicyDecisionPoint.create(kind, arguments);
pdp.registerListener(PolicyEvaluationSlf4jLogger.getInstance());
CACHE.put(key, pdp);
}
}
}
return CACHE.get(key);
}
URL getUrl() {
String adcUrl = readUrlSystemEnv();
if (Objects.isNull(adcUrl)) {
LOGGER.debug("ADC_URL System environment variable was null, using default value");
adcUrl = "http://localhost:8181";
}
try {
return new URL(adcUrl);
} catch (MalformedURLException urlException) {
LOGGER.error("PolicyDecisionPoint instantiation failed due to erroneous ADC_URL {}.", adcUrl);
throw new IllegalStateException(
"PolicyDecisionPoint instantiation failed due to erroneous ADC_URL: " + adcUrl, urlException);
}
}
String readUrlSystemEnv() {
return System.getenv(ENV_ADC_URL);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy