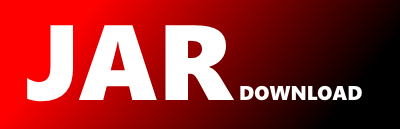
com.sap.cloud.security.ams.factory.TestDcnPolicyDecisionPointFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-ams-test Show documentation
Show all versions of java-ams-test Show documentation
Testing Utilities for applications using SAP Authorization Management Service (AMS) and it's client libraries
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.factory;
import com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPoint;
import com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPointFactory;
import com.sap.cloud.security.ams.logging.PolicyEvaluationSlf4jLogger;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This shall be called with {@code PolicyDecisionPoint.create(DEFAULT)} or {@code
* PolicyDecisionPoint.create(TEST_SERVER)}. It has to be registered as service to {@code
* META-INF.services/com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPointFactory}.
*/
public class TestDcnPolicyDecisionPointFactory extends PolicyDecisionPointFactory {
public static final String DEFAULT = "default";
public static final String TEST_SERVER = "test-server";
public static final String SKIP_CACHE = "skip_cache";
private static final Logger LOGGER =
LoggerFactory.getLogger(TestDcnPolicyDecisionPointFactory.class);
/**
* The output location can be overwritten to support setups, where the compiled results are
* located project specific. Also, other build infrastructures (e.g. gradle) can be integrated.
*/
static final String DCL_DEFAULT_LOCATION_PROPERTY = "sap.security.authorization.dcl.test-sources";
/** Default output location for maven builds. */
static final String DCL_DEFAULT_LOCATION = "target" + File.separatorChar + "dcl_opa";
private static final String DCL_RUNTIME_SERVER = "server:dcn-new";
private static final int PRIORITY = 90;
@Override
protected PolicyDecisionPoint tryCreate(String kind, Object[] arguments) throws IOException {
if (DEFAULT.equalsIgnoreCase(kind) || TEST_SERVER.equalsIgnoreCase(kind)) {
Object[] extArguments = Arrays.copyOf(arguments, arguments.length + 2);
extArguments[arguments.length] = "sources";
extArguments[arguments.length + 1] = getDclSourcesLocation();
return getOrCreate(DCL_RUNTIME_SERVER, extArguments);
}
return null; // can not handle this kind
}
@Override
protected int getPriority() {
return PRIORITY; // Overwrites the DEFAULT of AmsPolicyDecisionPointFactory by priority
}
PolicyDecisionPoint getOrCreate(String kind, Object[] arguments) {
Object[] extArguments = Arrays.copyOf(arguments, arguments.length + 2);
extArguments[arguments.length] = SKIP_CACHE;
extArguments[arguments.length + 1] = true;
PolicyDecisionPoint pdp = PolicyDecisionPoint.create(kind, extArguments);
pdp.registerListener(PolicyEvaluationSlf4jLogger.getInstance());
return pdp;
}
File getDclSourcesLocation() throws IOException {
File file = new File(readDclLocationSystemProperty()).getCanonicalFile();
if (!file.isDirectory()) {
String message =
"PolicyDecisionPoint instantiation failed due to erroneous dcl source folder '"
+ file
+ "'. Cannot start DCL runtime.";
LOGGER.error(message);
throw new IllegalStateException(message);
}
LOGGER.debug("PolicyDecisionPoint gets initialized with files from {}", file);
return file;
}
String readDclLocationSystemProperty() {
return System.getProperty(DCL_DEFAULT_LOCATION_PROPERTY, DCL_DEFAULT_LOCATION).trim();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy