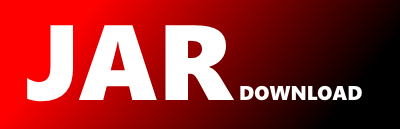
com.sap.cloud.security.ams.spring.adapter.PolicyDecisionPointSecurityExpression Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-ams Show documentation
Show all versions of spring-ams Show documentation
Client Library for integrating Spring applications with SAP Authorization Management Service (AMS)
The newest version!
/************************************************************************
* © 2019-2023 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.spring.adapter;
import static com.sap.cloud.security.ams.dcl.client.pdp.Attributes.Names.APP;
import static com.sap.cloud.security.ams.dcl.client.pdp.Attributes.Names.ENV;
import com.sap.cloud.security.ams.api.Principal;
import com.sap.cloud.security.ams.api.PrincipalBuilder;
import com.sap.cloud.security.ams.dcl.client.pdp.PolicyDecisionPoint;
import java.util.Arrays;
import java.util.Collections;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.security.access.expression.method.MethodSecurityExpressionOperations;
import org.springframework.security.authentication.AuthenticationTrustResolver;
import org.springframework.security.authentication.AuthenticationTrustResolverImpl;
import org.springframework.security.core.Authentication;
import java.util.Map;
/**
* An extension class for Method Security expressions
*/
public class PolicyDecisionPointSecurityExpression implements MethodSecurityExpressionOperations {
private final Logger logger = LoggerFactory.getLogger(getClass());
private PolicyDecisionPoint policyDecisionPoint;
private Principal principal;
private Object filterObject;
private Object returnObject;
protected final Authentication authentication;
private final AuthenticationTrustResolver trustResolver = new AuthenticationTrustResolverImpl();
public PolicyDecisionPointSecurityExpression(Authentication authentication) {
this.authentication = authentication;
logger.debug("Create PolicyDecisionPointWebSecurityExpression for anonymous user.");
}
public PolicyDecisionPointSecurityExpression(Authentication authentication, Map claims) {
this.authentication = authentication;
logger.debug("Create PolicyDecisionPointWebSecurityExpression with authentication.");
this.principal = PrincipalBuilder.create(claims).build();
}
public PolicyDecisionPointSecurityExpression(Authentication authentication, Principal principal) {
this.authentication = authentication;
logger.debug("Create PolicyDecisionPointWebSecurityExpression with authentication and principal.");
this.principal = principal;
}
public PolicyDecisionPointSecurityExpression policyDecisionPoint(PolicyDecisionPoint policyDecisionPoint) {
this.policyDecisionPoint = policyDecisionPoint;
return this;
}
public boolean forAction(String action, String... attributes) {
return forResourceAction(null, action, attributes);
}
public boolean forResource(String resource, String... attributes) {
return forResourceAction(resource, null, attributes);
}
public boolean forResourceAction(String resource, String action, String... attributes) {
if (isAnonymous() || principal == null) {
return false;
}
return PolicyDecisionPointPermissionEvaluator.hasPermission(policyDecisionPoint, principal,
resource, action,
attributes);
}
public boolean hasBaseAuthority(String action, String resource) {
if (isAnonymous() || principal == null) {
return false;
}
var attributes = this.principal.getAttributes();
var temp = attributes.getIgnores();
attributes.setIgnores(Collections.unmodifiableList(Arrays.asList(APP, ENV)));
boolean hasBaseAuthority = forResourceAction(resource, action);
attributes.setIgnores(temp);
logger.debug("{} hasBaseAuthority() for action '{}' on resource '{}' ? {}.", principal, action, resource,
hasBaseAuthority);
return hasBaseAuthority;
}
@Override
public final Authentication getAuthentication() {
return this.authentication;
}
@Override
public boolean hasAuthority(String action) {
if (isAnonymous() || principal == null) {
return false;
}
return PolicyDecisionPointPermissionEvaluator.hasPermission(policyDecisionPoint, principal, action);
}
@Override
public boolean hasAnyAuthority(String... actions) {
if (isAnonymous()) {
return false;
}
for (String action : actions) {
if (hasAuthority(action)) {
return true;
}
}
return false;
}
@Override
public final boolean hasRole(String s) {
throw new UnsupportedOperationException("method hasRole() is not supported");
}
@Override
public final boolean hasAnyRole(String... strings) {
throw new UnsupportedOperationException("method hasAnyRole() is not supported");
}
@Override
public final boolean permitAll() {
return true;
}
@Override
public final boolean denyAll() {
return false;
}
@Override
public boolean isAnonymous() {
return trustResolver.isAnonymous(authentication);
}
@Override
public final boolean isAuthenticated() {
return !isAnonymous();
}
@Override
public final boolean isRememberMe() {
return trustResolver.isRememberMe(authentication);
}
@Override
public final boolean isFullyAuthenticated() {
return !trustResolver.isAnonymous(authentication)
&& !trustResolver.isRememberMe(authentication);
}
@Override
public boolean hasPermission(Object o, Object o1) {
throw new UnsupportedOperationException("method hasPermission() is not supported");
}
@Override
public boolean hasPermission(Object o, String s, Object o1) {
throw new UnsupportedOperationException("method hasPermission() is not supported");
}
@Override
public void setFilterObject(Object filterObject) {
this.filterObject = filterObject;
}
@Override
public Object getFilterObject() {
return filterObject;
}
@Override
public void setReturnObject(Object returnObject) {
this.returnObject = returnObject;
}
@Override
public Object getReturnObject() {
return returnObject;
}
@Override
public Object getThis() {
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy