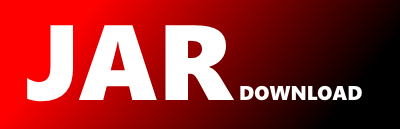
com.sap.cloud.security.ams.dcl.spi.language.DataControlLanguageSpiTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spi Show documentation
Show all versions of spi Show documentation
DCL Service Provider Interface
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cloud.security.ams.dcl.spi.language;
import static com.sap.cloud.security.ams.dcl.client.language.TokenFormat.ALPHA_NUMERIC_EXT;
import com.sap.cloud.security.ams.dcl.client.language.DataControlLanguageTools;
public class DataControlLanguageSpiTools {
private DataControlLanguageSpiTools() {
}
public static String createQualifiedOperation(String dclPackage, String operation) {
if (!ALPHA_NUMERIC_EXT.isToken(operation)) {
DataControlLanguageTools.requireNonNullOrEmpty(operation, "Operation must not be null.",
"Operation must not be an empty string.");
throw new IllegalArgumentException("Operation is not valid: " + operation);
}
if (dclPackage == null || dclPackage.isEmpty()) {
return operation;
}
if (!ALPHA_NUMERIC_EXT.isQualifiedToken(dclPackage)) {
if (dclPackage.charAt(dclPackage.length() - 1) == DataControlLanguageTools.SEPARATOR_CHAR) {
throw new IllegalArgumentException(
"DCL package must not end with '" + DataControlLanguageTools.SEPARATOR_CHAR + "'");
} else {
throw new IllegalArgumentException("The DCL package is not valid: " + dclPackage);
}
}
return dclPackage + DataControlLanguageTools.SEPARATOR_CHAR + operation;
}
//
public static String checkQualifiedOperation(String operation) {
if (!ALPHA_NUMERIC_EXT.isQualifiedToken(operation)) {
DataControlLanguageTools.requireNonNullOrEmpty(operation, "Qualified operation must not be null.",
"Qualified operation must not be empty.");
throw new IllegalArgumentException("The qualified operation is not a valid: " + operation);
}
return operation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy