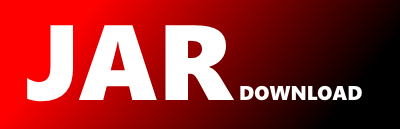
com.sap.cloud.security.token.validation.validators.JsonWebKeySet Maven / Gradle / Ivy
package com.sap.cloud.security.token.validation.validators;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.HashSet;
import java.util.Set;
import java.util.stream.Stream;
class JsonWebKeySet {
private Set jsonWebKeys;
JsonWebKeySet() {
jsonWebKeys = new HashSet<>();
}
@Nullable
public JsonWebKey getKeyByAlgorithmAndId(JwtSignatureAlgorithm keyAlgorithm, String keyId) {
return getTokenStreamWithTypeAndKeyId(keyAlgorithm, keyId)
.findFirst()
.orElse(null);
}
public Set getAll() {
return jsonWebKeys;
}
public boolean put(@Nonnull JsonWebKey jsonWebKey) {
return jsonWebKeys.add(jsonWebKey);
}
public void putAll(JsonWebKeySet jsonWebKeySet) {
jsonWebKeys.addAll(jsonWebKeySet.getAll());
}
private Stream getTokenStreamWithTypeAndKeyId(JwtSignatureAlgorithm algorithm, String keyId) {
String kid = keyId != null ? keyId : JsonWebKey.DEFAULT_KEY_ID;
return jsonWebKeys.stream()
.filter(jwk -> algorithm.equals(jwk.getKeyAlgorithm()))
.filter(jwk -> kid.equals(jwk.getId()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy