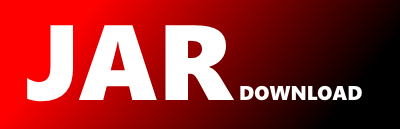
com.sap.it.commons.data.Pair Maven / Gradle / Ivy
package com.sap.it.commons.data;
import java.io.Serializable;
import com.sap.it.commons.lang.Equals;
import com.sap.it.commons.lang.Hash;
public class Pair implements Serializable {
private static final long serialVersionUID = 8719382431393826469L;
private final T first;
private final U second;
public Pair(T a, U b) {
this.first = a;
this.second = b;
}
public T getFirst() {
return first;
}
public U getSecond() {
return second;
}
@Override
public int hashCode() {
return Hash.hash(first, second);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Pair)) {
return false;
}
@SuppressWarnings("unchecked")
Pair other = (Pair) obj;
return Equals.equals(first, other.first) && //
Equals.equals(second, other.second);
}
@Override
public String toString() {
return "[" + first + ", " + second + "]"; //$NON-NLS-1$ //$NON-NLS-2$ //$NON-NLS-3$
}
public static Pair create(T a, U b) {
return new Pair(a, b);
}
@SuppressWarnings("unchecked")
public static final Pair nullPair() {
return (Pair) NULL_PAIR;
}
@SuppressWarnings("rawtypes")
public static final Pair NULL_PAIR = new Pair
© 2015 - 2025 Weber Informatics LLC | Privacy Policy